In this article, we will show the list of Python programs that print the star pattern of the alphabet from A to Z using the for loop with an example. In each example, we have provided a hyperlink to navigate to the individual articles that explain the Alphabet star pattern with the While loop and functions.
Python Program to Print Star Pattern of Alphabets
The following list will show the star pattern of the alphabet from A to Z using the for loop.
A Pattern of Stars
For more information on the Alphabet A Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) temp = n for i in range(n): for j in range(2 * n): if j == temp or j == ((2 * n) - temp) or (i == (n // 2) and temp < j < ((2 * n) - temp)): print('*', end='') else: print(end=' ') print() temp = temp - 1
Enter Number = 11
*
* *
* *
* *
* *
***********
* *
* *
* *
* *
* *
B Pattern of Stars
For more information on the Alphabet B Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): print('*', end='') for j in range(2 * n - 1): if (i == 0 or i == n - 1 or i == n // 2) and j < (2 * n) - 2: print('*', end='') elif j == (2 * n) - 2 and not (i == 0 or i == n - 1 or i == n // 2): print('*', end='') else: print(end=' ') print()
Enter Rows = 9
*****************
* *
* *
* *
*****************
* *
* *
* *
*****************
C Pattern of Stars
For more information on the Alphabet C Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) n = n // 2 + 1 print(" " + "*" * n) for i in range(n // 2): print("*" + " " * (n - 1)) print(" " + "*" * n)
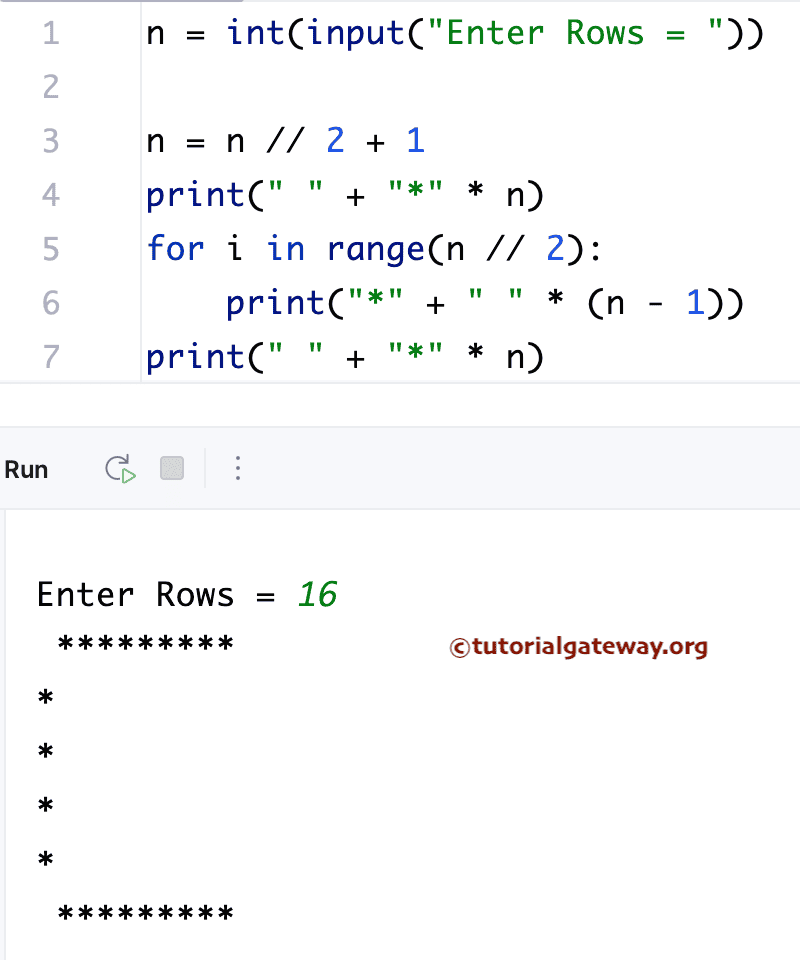
D Pattern of Stars
For more information on the Alphabet D Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) temp = n // 2 + 1 print("*" * temp + " " * (n - 1)) for i in range(n - 2): print("*" + " " * (n - 2) + "*") print("*" * temp + " " * (n - 1))
Enter Number = 11
******
* *
* *
* *
* *
* *
* *
* *
* *
* *
******
Python Program to Print Star Pattern of Alphabet E
For more information on the Alphabet E Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): print('*', end='') for j in range(n): if (i == 0 or i == n - 1) or (i == n // 2 and j <= n // 2): print('*', end='') else: continue print()
Enter Number = 13
**************
*
*
*
*
*
********
*
*
*
*
*
**************
F Pattern of Stars
For more information on the Alphabet F Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): print('*', end='') for j in range(n): if i == 0 or (i == n // 2 and j <= n // 2): print('*', end='') else: continue print()
Enter Number = 11
************
*
*
*
*
*******
*
*
*
*
*
G Pattern of Stars
For more information on the Alphabet G Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(2 * n): if (i == 0 or i == n - 1) and (j == 0 or j == (2 * n) - 2): print(end=" ") elif j == 0 or (i == 0 and j <= n) or (i == n // 2 and j > n // 2) or \ (i > n // 2 and j == (2 * n) - 1) or (i == n - 1 and j < 2 * n): print("*", end="") else: print(end=" ") print()
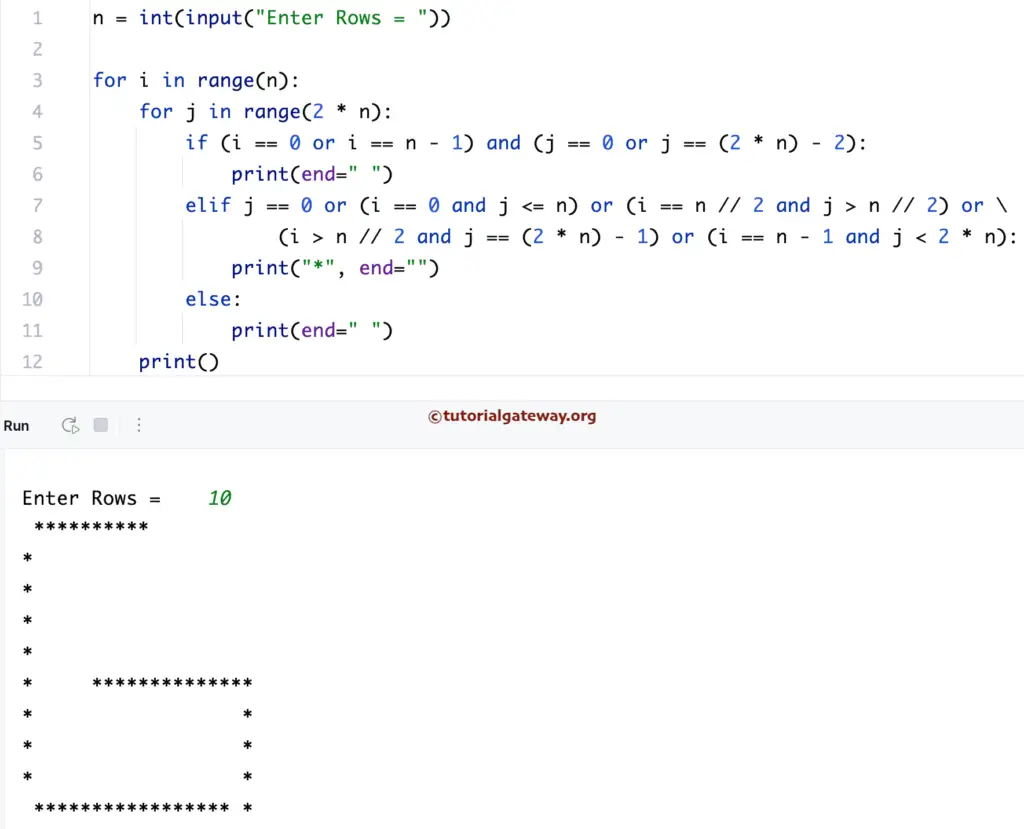
H Pattern of Stars
For more information on the Alphabet H Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): print('*', end='') for j in range(n): if i == n // 2 or j == n - 1: print('*', end='') else: print(end=' ') print()
Enter Number = 12
* *
* *
* *
* *
* *
* *
*************
* *
* *
* *
* *
* *
I Pattern of Stars
For more information on the Alphabet I Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if i == 0 or i == n - 1 or j == n // 2: print("*", end="") else: print(end=" ") print()
Enter Rows = 12
************
*
*
*
*
*
*
*
*
*
*
************
Python Program to Print Star Pattern of Alphabet J
For more information on the Alphabet J Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if i == n - 1 and (0 < j < n - 1): print("*", end="") elif (j == n - 1 and i != n - 1) or (i > n // 2 and j == 0 and i != n - 1): print("*", end="") else: print(end=" ") print()
Enter Rows = 12
*
*
*
*
*
*
*
* *
* *
* *
* *
**********
K Pattern of Stars
For more information on the Alphabet K Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) temp = n // 2 for i in range(n): print("*", end="") for j in range(temp + 1): if j == abs(temp - i): print("*", end="") else: print(end=" ") print()
Enter Rows = 13
* *
* *
* *
* *
* *
* *
**
* *
* *
* *
* *
* *
* *
L Pattern of Stars
For more information on the Alphabet L Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(n + 1): if i == n - 1 or j == 0: print("*", end="") else: print(end=" ") print()
Enter Number = 10
*
*
*
*
*
*
*
*
*
***********
M Pattern of Stars
For more information on the Alphabet M Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): print("*", end="") for j in range(n + 1): if j == n or (j == i - 1 and j < n // 2) or (j == n - i and j > n // 2): print("*", end="") else: print(end=" ") print()
Enter Rows = 10
* *
** **
* * * *
* * * *
* * * *
* * *
* *
* *
* *
* *
Python Program to Print Star Pattern of Alphabet N
For more information on the Alphabet N Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): print("*", end="") for j in range(n + 1): if j == n or j == i: print("*", end="") else: print(end=" ") print()
Enter Rows = 12
** *
* * *
* * *
* * *
* * *
* * *
* * *
* * *
* * *
* * *
* * *
* **
O Pattern of Stars
For more information on the Alphabet O Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if ((i == 0 or i == n - 1) and (0 < j < n - 1)) or \ ((j == 0 or j == n - 1) and (0 < i < n - 1)): print("*", end="") else: print(end=" ") print()
Enter Rows = 10
********
* *
* *
* *
* *
* *
* *
* *
* *
********
P Pattern of Stars
For more information on the Alphabet P Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if j == 0 or (i == 0 or i == n // 2) and j < n - 1: print("*", end="") elif i != 0 and i < n // 2 and j == n - 1: print("*", end="") else: print(end=" ") print()
Enter Rows = 11
**********
* *
* *
* *
* *
**********
*
*
*
*
*
Python Program to Print Star Pattern of Alphabet Q
For more information on the Alphabet Q Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(n): if (i == 0 or i == n - 2) and (0 < j < n - 1) or \ (j == 0 or j == n - 1) and (0 < i < n - 2) or \ (n // 2 < i < n) and (n // 2 < j == i): print("*", end=" ") else: print(" ", end=" ") print()
Enter Number = 10
* * * * * * * *
* *
* *
* *
* *
* *
* * *
* * *
* * * * * * * *
*
R Pattern of Stars
For more information on the Alphabet R Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if (i == 0 or i == n // 2) and 0 < j < n - 1: print("*", end=" ") elif i != 0 and (j == 0 or (j == n - 1 and i < n // 2)): print("*", end=" ") elif i == j and i >= n // 2: print("*", end=" ") else: print(" ", end=" ") print()
Enter Rows = 11
* * * * * * * * *
* *
* *
* *
* *
* * * * * * * * * *
* *
* *
* *
* *
* *
S Pattern of Stars
For more information on the Alphabet S Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if (i == 0 or i == n // 2 or i == n - 1) and j != 0 and j != n - 1: print("*", end=" ") elif (i != 0 and j == 0 and i < n // 2) or (j == n - 1 and i > n // 2 and i != n - 1): print("*", end=" ") else: print(" ", end=" ") print()
Enter Rows = 13
* * * * * * * * * * *
*
*
*
*
*
* * * * * * * * * * *
*
*
*
*
*
* * * * * * * * * * *
T Pattern of Stars
For more information on the Alphabet T Pattern of Stars >> Click Here!
n = int(input("Enter Rows = ")) for i in range(n): for j in range(n): if i == 0 or j == n // 2: print("*", end=" ") else: print(" ",end=" ") print()
Enter Rows = 13
* * * * * * * * * * * * *
*
*
*
*
*
*
*
*
*
*
*
*
U Pattern of Stars
For more information on the Alphabet U Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(n): if ((j == 0 or j == n - 1) and i != n - 1) or (i == n - 1 and j != 0 and j != n - 1): print("*", end=" ") else: print(" ",end=" ") print()
Enter Number = 12
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* * * * * * * * * *
V Pattern of Stars
For more information on the Alphabet V Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(2 * n): if (i + j == 2 * n - 2 or i == j) and i < 2 * n / 2: print("*", end=" ") else: print(" ", end=" ") print()
Enter Number = 9
* *
* *
* *
* *
* *
* *
* *
* *
*
W Pattern of Stars
For more information on the Alphabet W Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(n): if (j == 0 or j == n - 1) or ((i + j == n - 1 or i == j) and i >= n // 2): print("*", end=" ") else: print(" ", end=" ") print()
Enter Number = 11
* *
* *
* *
* *
* *
* * *
* * * *
* * * *
* * * *
* * * *
* *
X Pattern of Stars
For more information on the Alphabet X Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(n): if i == j or j == n - 1 - i: print('*', end='') else: print(' ', end='') print()
Enter Number = 11
* *
* *
* *
* *
* *
*
* *
* *
* *
* *
* *
Y Pattern of Stars
For more information on the Alphabet Y Pattern of Stars >> Click Here!
n = int(input("Enter Number = ")) for i in range(n): for j in range(n): if (i + j == n - 1 or i == j) and (i < n // 2) or (j == n // 2 and i > n // 2 - 1): print("*", end=" ") else: print(" ", end=" ") print()
Enter Number = 11
* *
* *
* *
* *
* *
*
*
*
*
*
*
Z Pattern of Stars
For more information on the Alphabet Z Pattern of Stars >> Click Here!
number = int(input("Enter Number = ")) for i in range(number): for j in range(number): if i == 0 or i == number - 1 or j == number - i - 1: print("*", end=" ") else: print(" ",end=" ") print()
Enter Number = 10
* * * * * * * * * *
*
*
*
*
*
*
*
*
* * * * * * * * * *