This article shows how to write a Python program to print the Alphabet K star pattern using the for loop, while loop, and functions with an example.
The below alphabet K star pattern example accepts the user-entered rows and the nested for loop iterates the rows. The If else condition is to print stars at a few positions to get the Alphabetical K pattern of stars and skip others.
rows = int(input("Enter Alphabet K of Stars Rows = ")) print("====The Alphabet K Star Pattern====") n = rows // 2 for i in range(rows): print("*", end="") for j in range(n + 1): if j == abs(n - i): print("*", end="") else: print(end=" ") print()
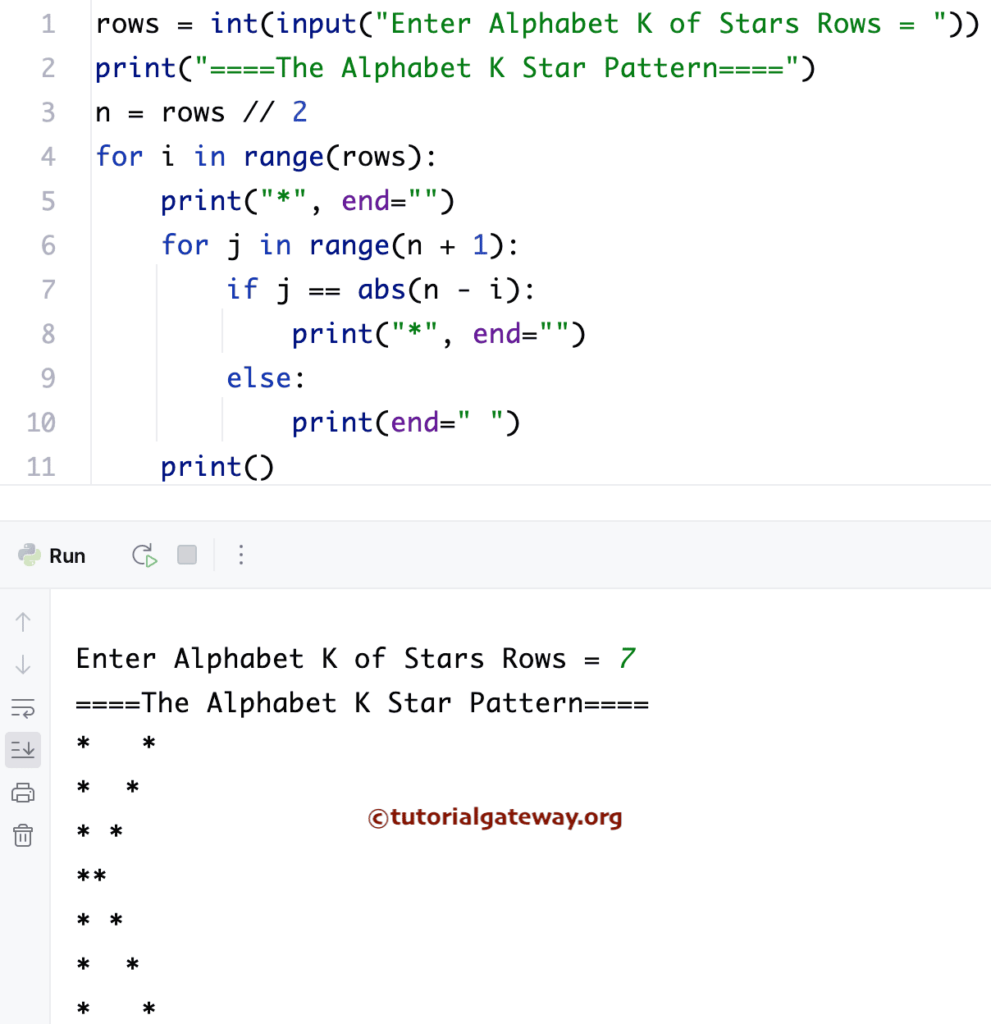
Python program to print the Alphabet K Star pattern using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet K pattern rows and prints the stars at the required positions. Here, the abs function returns the absolute positive value. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet K of Stars Rows = ")) n = rows // 2 i = 0 while i < rows: print("*", end="") j = 0 while j < n + 1: if j == abs(n - i): print("*", end="") else: print(end=" ") j = j + 1 print() i = i + 1
Enter Alphabet K of Stars Rows = 13
* *
* *
* *
* *
* *
* *
**
* *
* *
* *
* *
* *
* *
In this Python example, we created a KPattern function that accepts the rows and the symbol or character to print the Alphabet K pattern of the given symbol.
def KPattern(rows, ch): n = rows // 2 for i in range(rows): print('%c' %ch, end='') for j in range(n + 1): if j == abs(n - i): print('%c' %ch, end='') else: print(end=" ") print() row = int(input("Enter Alphabet K of Stars Rows = ")) sy = input("Symbol for K Star Pattern = ") KPattern(row, sy)
Enter Alphabet K of Stars Rows = 14
Symbol for K Star Pattern = $
$ $
$ $
$ $
$ $
$ $
$ $
$ $
$$
$ $
$ $
$ $
$ $
$ $
$ $