Write a Python Program to Find Prime Number using For Loop, While Loop, and Functions. Any natural number that is not divisible by any other except 1 and itself is called a Prime.
The list goes like 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, etc. 2 is the only even num.
Python Program to find Prime Number using For Loop
This Python program allows the user to enter any integer value and checks whether the given number is a Prime or Not using For Loop.
Number = int(input(" Please Enter any Number: ")) count =Number = int(input("Please Enter any Value: ")) count = 0 for i in range(2, (Number//2 + 1)): if(Number % i == 0): count = count + 1 break if (count == 0 and Number != 1): print(" %d is a Prime" %Number) else: print(" %d is Not" %Number)
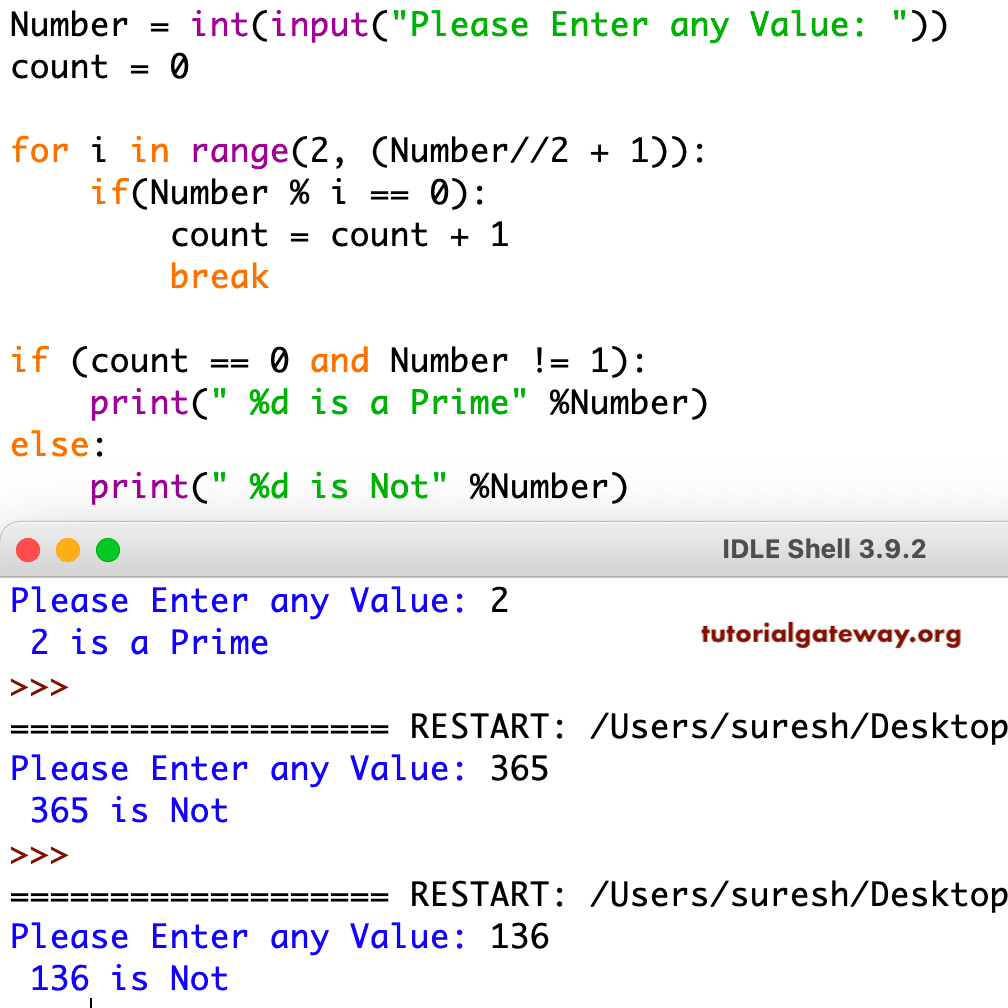
Within the for loop, there is an If statement to check whether the value divisible by i is exactly equal to 0 or not. If the condition is True, then the Count value is incremented, and then the Break Statement is executed. Next, we used another If statement to check whether the Count is Zero and whether the Num is Not equal to 1.
The user entered integer in the above example is 365.
First Iteration: for i in range(2, 365//2). It means, for i in range (2, 182.5) – The condition is True. Now, Check the if condition – if (365%2 == 0). As you know, the condition is False. Next, i become 3
Do the same for the remaining For loop iterations of the Python Program to find the Prime Number.
Next, it enters into the Python If statement. if(count == 0 && Number != 1 ). In all the above iterations, If the condition failed, the Count Value has not incremented from initialized o. And the one that we used is 365 (not zero). So, the condition is True, which means Prime.
Python Program to find Prime Number using While Loop
This program is the same as the above. We just replaced the For loop in the above program with While.
# using While Loop Number = int(input(" Please Enter any Num: ")) count = 0 i = 2 while(i <= Number//2): if(Number % i == 0): count = count + 1 break i = i + 1 if (count == 0 and Number != 1): print(" %d is a Prime" %Number) else: print(" %d is not" %Number)
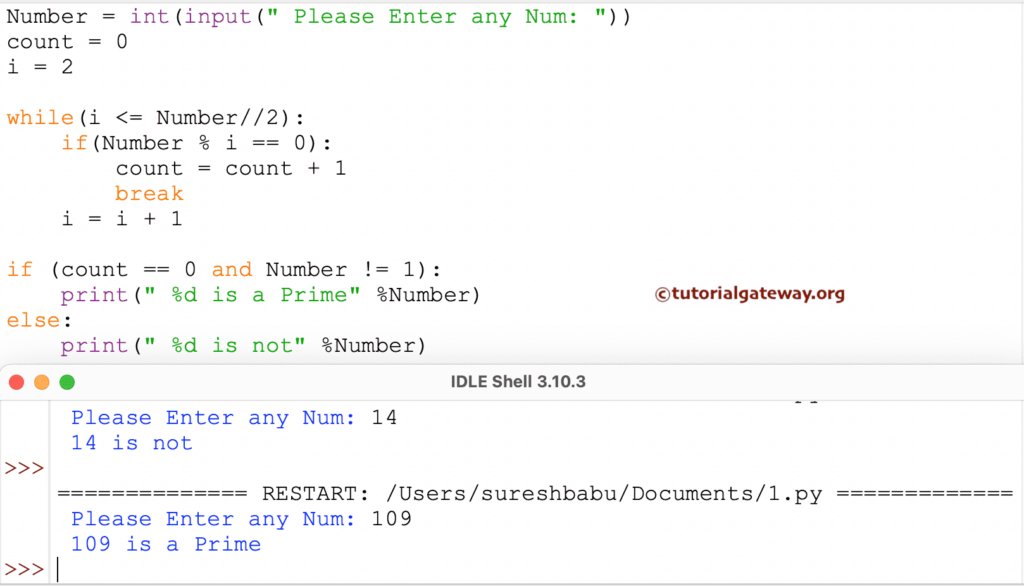
Python Program to find Prime Number using Functions
This program is the same as the first example. However, we separated the factor’s logic by defining the new Function. Next, the If Else statement checks whether the given number is prime or not.
# using Functions def finding_factors(Number): count = 0 for i in range(2, (Number//2 + 1)): if(Number % i == 0): count = count + 1 return count Num = int(input(" Please Enter any Num: ")) cnt = finding_factors(Num) if (cnt == 0 and Num != 1): print(" %d is a Prime" %Num) else: print(" %d is not" %Num)
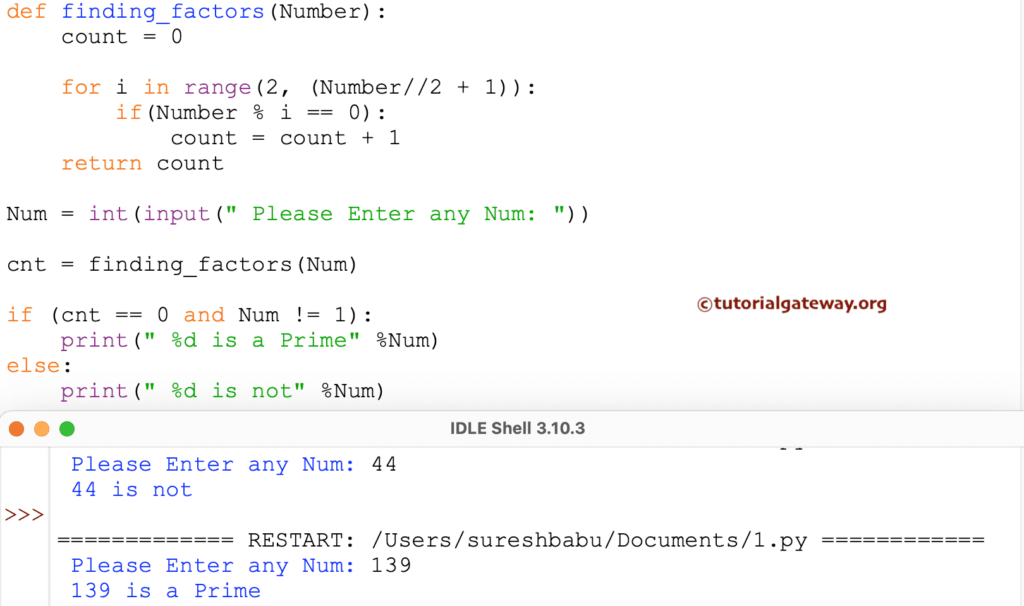