How to write a Python program to find Perfect Number using For Loop, While Loop, and Functions.
Python Perfect Number
Any number can be the perfect number in Python if the sum of its positive divisors excluding the number itself is equal to that number.
For example, 6 is a perfect number in Python because 6 is divisible by 1, 2, 3, and 6. So, the sum of these values are 1+2+3 = 6 (Remember, we have to exclude the number itself. That’s why we haven’t added 6 here). Some of the perfect numbers are 6, 28, 496, 8128 and 33550336 so on.
Python Program to find Perfect Number using For loop
This Python program for Perfect Number allows the user to enter any number. Using this number it will calculate whether the number is a Perfect number or not using the Python For Loop.
# find Perfect Number using For loop Number = int(input(" Please Enter any Number: ")) Sum = 0 for i in range(1, Number): if(Number % i == 0): Sum = Sum + i if (Sum == Number): print(" %d is a Perfect Number" %Number) else: print(" %d is not a Perfect Number" %Number)
Within this Python perfect number program, the following statement will ask the user to enter any number and store the user entered value in the variable Number
Number = int(input(" Please Enter any Number: "))
Next line, We declared the integer variable Sum = 0. Next, we used the for loop with range() and we are not going to explain the for loop here. If you don’t understand for loop then please visit our article Python For Loop.
for i in range(1, Number):
Inside the For loop, we placed Python If Statement to test the condition
if(Number % i == 0): Sum = Sum + i
The If statement inside the for loop will check whether the Number is Perfectly divisible by i value or not. If the number is perfectly divisible by i then i value will be added to the Sum. Please refer to the If Statement article to understand the If statement in Python.
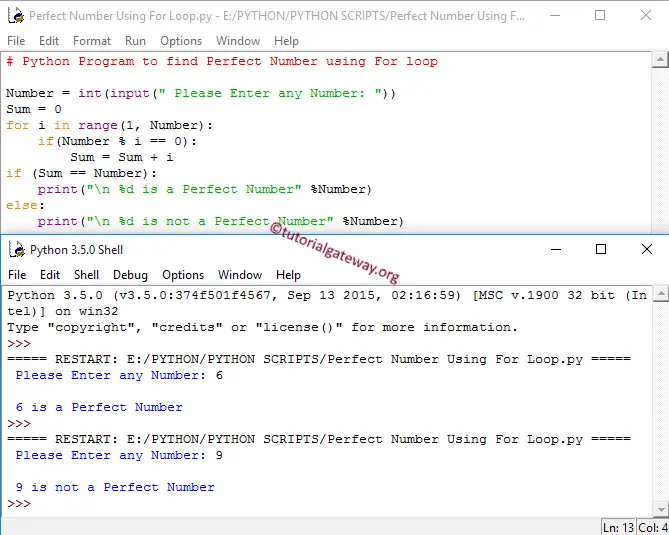
From the above Python perfect number example, the User Entered value is: Number = 6
First Iteration: For the first Iteration, Number = 6, Sum = 0 and i = 1
If (Number % i == 0)
6 % 1 == 0
The If statement is succeeded here So,
Sum = Sum + i
Sum = 0 +1 = 1
Second Iteration: For the second Iteration the values of both Sum and i have been changed as: Sum = 1 and i = 2
If (Number % i == 0)
6 % 2 == 0
The If statement is succeeded here So,
Sum = Sum + i
Sum = 1 + 2 = 3
Third Iteration: For the third Iteration o this Python perfect number program, the values of both Sum and i have been changed as Sum = 3 and i = 3.
If (Number % i == 0)
6 % 3 == 0
The If statement is succeeded here So,
Sum = Sum + i
Sum = 3 + 3 = 6
For the fourth and fifth iterations condition inside the if condition will fail.
6 % 4 == 0 (FALSE)
6 % 5 == 0 (FALSE)
So, the compiler will terminate the for loop.
In the next line, we have an If statement to check whether the value inside the Sum variable is exactly equal to the given Number or Not.
If the condition (Sum == Number) is TRUE, the print statement below will execute.
print(" %d is a Perfect Number" %Number)
If the condition (Sum == Number) is FALSE, the print statement below will execute.
print(" %d is not a Perfect Number" %Number)
For this example (Sum == Number) is True. So, the given Number is the Perfect Number.
Python Program to find Perfect Number using While Loop
This Python perfect number program allows the user to enter any number. Using this number it will calculate whether the user input is a Perfect number or not using Python While loop.
# find Perfect Number using While loop Number = int(input(" Please Enter any Number: ")) i = 1 Sum = 0 while(i < Number): if(Number % i == 0): Sum = Sum + i i = i + 1 if (Sum == Number): print(" %d is a Perfect Number" %Number) else: print(" %d is not the Perfect Number" %Number)
We haven’t done anything special in this example. We just replaced the For loop in the above Python program with While Loop and if you find it difficult to understand the While loop functionality then please refer Python While Loop article.
Please Enter any Number: 496
496 is a Perfect Number
>>>
Please Enter any Number: 525
525 is not the Perfect Number
Python Program to find Perfect Number using Functions
This program for Perfect Number allows the user to enter any number. Using this number it will calculate whether the number is a Perfect number or not using the Functions.
# Python Program to find Perfect Number using Functions def Perfect_Number(Number): Sum = 0 for i in range(1, Number): if(Number % i == 0): Sum = Sum + i return Sum # Taking input from the user Number = int(input("Please Enter any number: ")) if (Number == Perfect_Number(Number)): print("\n %d is a Perfect Number" %Number) else: print("\n %d is not a Perfect Number" %Number)
If you observe the above code, We haven’t done anything special in this example. We just defined the new user defined function and added the code we mentioned in For loop example.
Please Enter any number: 33550336
33550336 is a Perfect Number
>>>
Please Enter any number: 54
54 is not a Perfect Number
In this example, we used the for loop and the if statement to iterate and find divisors. Next, we assign those divisors to a list and use the list sum()method to find the total.
def isPerfectNumber(n): divisors = [] for i in range(1, n): if n % i == 0: divisors.append(i) if n == sum(divisors): return True else: return False num = int(input("Enter any Value = ")) if isPerfectNumber(num): print(num," is a Perfect Number.") else: print(num, " is Not.")
Enter any Value = 28
28 is a Perfect Number.
Python Program to find Perfect Number using List Comprehension
def isPerfectNumber(n): divisors = [i for i in range(1, n) if n % i == 0] return sum(divisors) num = int(input("Enter any Value = ")) if num == isPerfectNumber(num): print(num," is a Perfect Number.") else: print(num, " is Not.")
Enter any Value = 225
225 is Not.
We can also write the above program
def isPerfectNumber(n): divisors = sum([i for i in range(1, n) if n % i == 0]) return n == divisors num = int(input("Enter any Value = ")) if isPerfectNumber(num): print(num," is a Perfect Number.") else: print(num, " is Not.")
Enter any Value = 496
496 is a Perfect Number.
Python Program to Find Perfect Number between 1 to 1000
This program allows the user to enter minimum and maximum values. Next, this program will find the Perfect Number between the Minimum and Maximum values.
# Python Program to find Perfect Number between 1 to 1000 # Taking input from the user Minimum = int(input("Please Enter any Minimum Value: ")) Maximum = int(input("Please Enter any Maximum Value: ")) for Number in range(Minimum, Maximum - 1): Sum = 0 for n in range(1, Number - 1): if(Number % n == 0): Sum = Sum + n # display the result if(Sum == Number): print(" %d " %Number)
Output
Please Enter any Minimum Value: 1
Please Enter any Maximum Value: 100
6
28
>>>
Please Enter any Minimum Value: 1
Please Enter any Maximum Value: 1000
6
28
496
Within this perfect number program, this For Loop helps the compiler to iterate between Minimum and Maximum Variables, iteration starts at the Minimum and then it will not exceed the Maximum variable.
for Number in range(Minimum, Maximum):
Inside the for loop we are checking whether that number is a perfect number or not. We already explained the for loop iteration in the first example.
Python Program to Print Perfect Numbers between 1 to 1000 using list comprehension
def isPerfectNumber(n): divisors = [i for i in range(1, n) if n % i == 0] return n == sum(divisors) for i in range(1, 1001): if isPerfectNumber(i): print(i, end= ' ')
6 28 496