This article discloses how to write a Python Program to Reverse a Number using the While Loop, Functions, slicing, and Recursion. To reverse a number, first, you must find the last digit in a number. Next, add it to the first position of the other variable, then remove that last digit from the original number.
Python Program to Reverse a Number Using While Loop
This Python program to reverse a number allows the user to enter any positive integer using a while loop. This program iterates each digit to inverse them.
Number = int(input("Please Enter any Number: ")) Reverse = 0 while(Number > 0): Reminder = Number %10 Reverse = (Reverse *10) + Reminder Number = Number //10 print("\n Reverse of entered number is = %d" %Reverse)
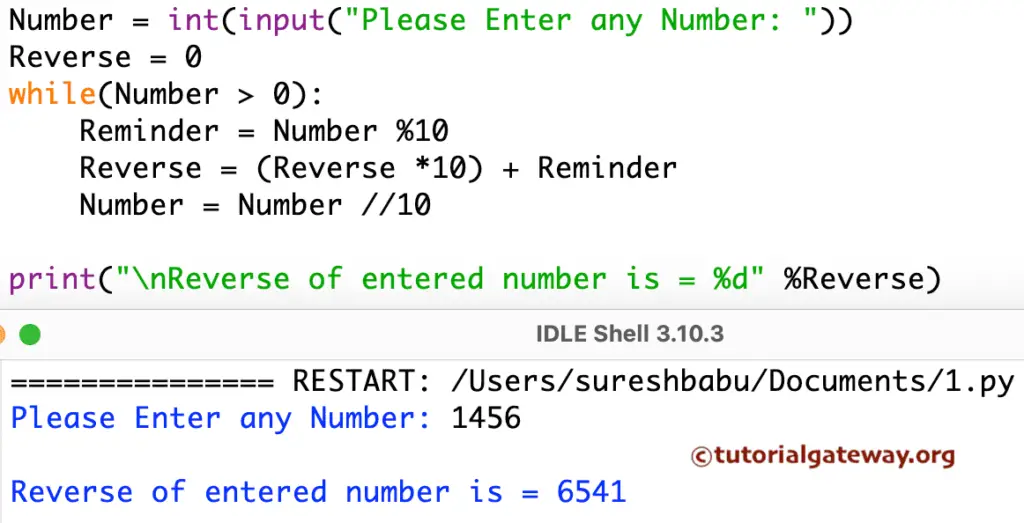
This program allows the user to enter any positive integer. Then, that number is assigned to a variable Number. Next, Condition in the Python While loop ensures that the given number is greater than 0.
From the above Python example program, the User Entered integer value: Number = 1456 and Reverse = 0
First Iteration
Reminder= Number%10
Reminder= 1456%10 = 6
Reverse= Reverse*10 + Reminder
Reverse= 0 * 10 + 6 = 0 + 6 = 6
Number= Number//10
Number= 1456 //10 = 145
Second Iteration: From the While Loop first Iteration, both the Number and Reverse values changed as Number= 145 and Reverse= 6.
Reminder= 145 % 10 = 5
Reverse = 6 * 10 + 5 => 60 + 5 = 65
Number= 145 //10 = 14
Third Iteration: From the Second Iteration of the Python reverse the int Number program, Number= 14 and Reverse= 65
Reminder= 14%10 = 4
Reverse= 65 * 10 + 4 => 650 + 4 = 654
Number= 14//10 = 1
Fourth Iteration: From the third Iteration, Number = 1 and Reverse = 654
Reminder = 1 %10 = 1
Reverse= 654 * 10 + 1 => 6540 + 1 = 6541
Number= 1//10 = 0
Here, For the next iteration, Number= 0. So, the while loop condition fails. So, the final output is 6541.
Python Program to Reverse a Number using for loop
This program uses the for loop range to iterate digits in a number and reverse them.
num = int(input("Enter any Number = ")) rv = 0 for i in range(len(str(num))): rv = rv * 10 + num % 10 num = num // 10 print("The Result = ", rv)
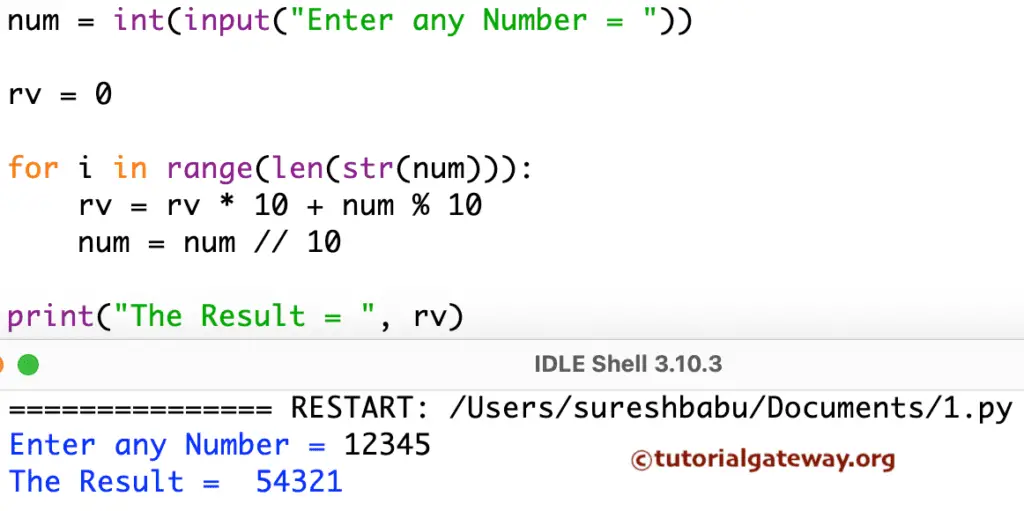
Enter any Number = 12345
The Result = 54321
Python Program to Reverse a Number using Functions
This program allows the user to enter any positive integer, and then we were going to reverse an int number using the Functions.
def rev_Integer(num): rev = 0 while(num > 0): rem = num %10 rev = (rev *10) + rem num = num //10 return rev num = int(input("Please Enter any Num: ")) rev = rev_Integer(num) print("\n Result = %d" %rev)
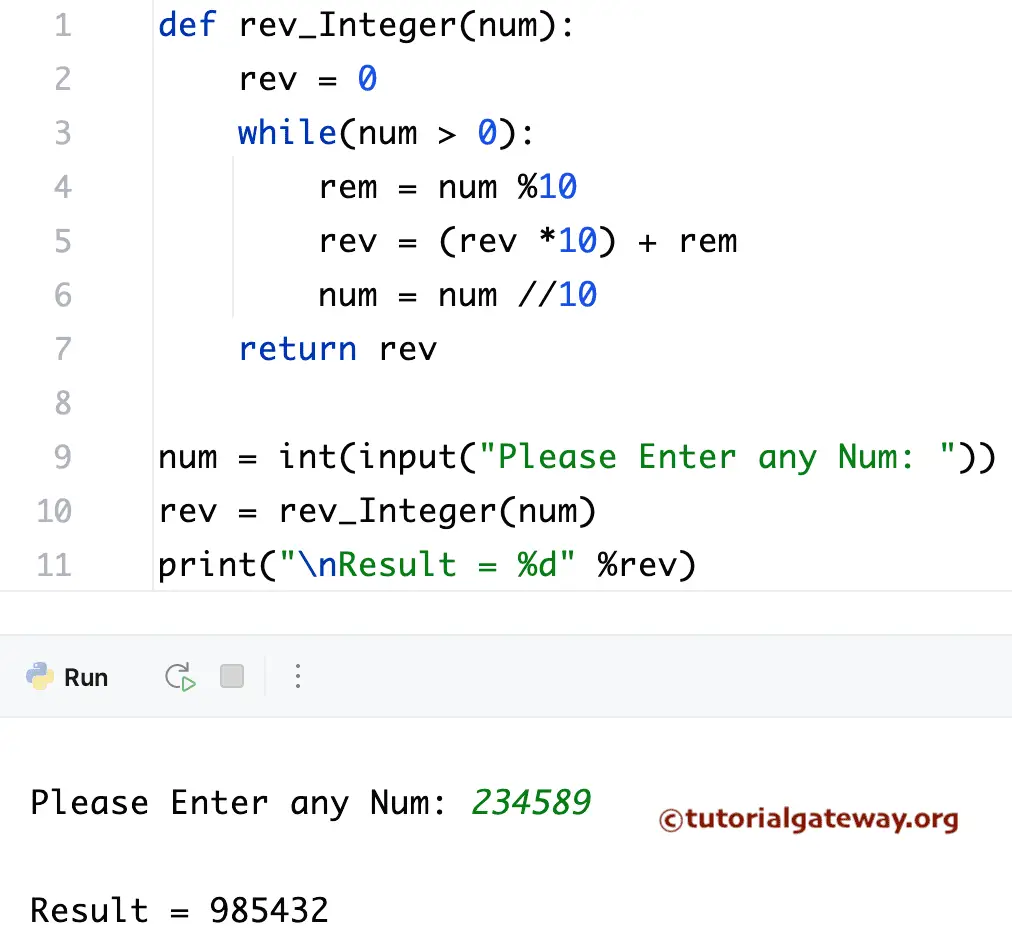
Within this program, When it reaches the rev = rev_Integer (num) line in the program, the compiler immediately jumps to the below function:
def rev_Integer(Number):
The last line ends with a return Reverse statement.
Python Program to Reverse a Number using Recursion
This program allows the user to enter any positive integer. And then, we are going to reverse a number using Recursion.
rv = 0 def rv_Int(nm): global rv if(nm > 0): Reminder = nm %10 rv = (rv *10) + Reminder rv_Int(nm //10) return rv nm = int(input("Please Enter any Value : ")) rv = rv_Int(nm) print("\n The Result of entered is = %d" %rv)
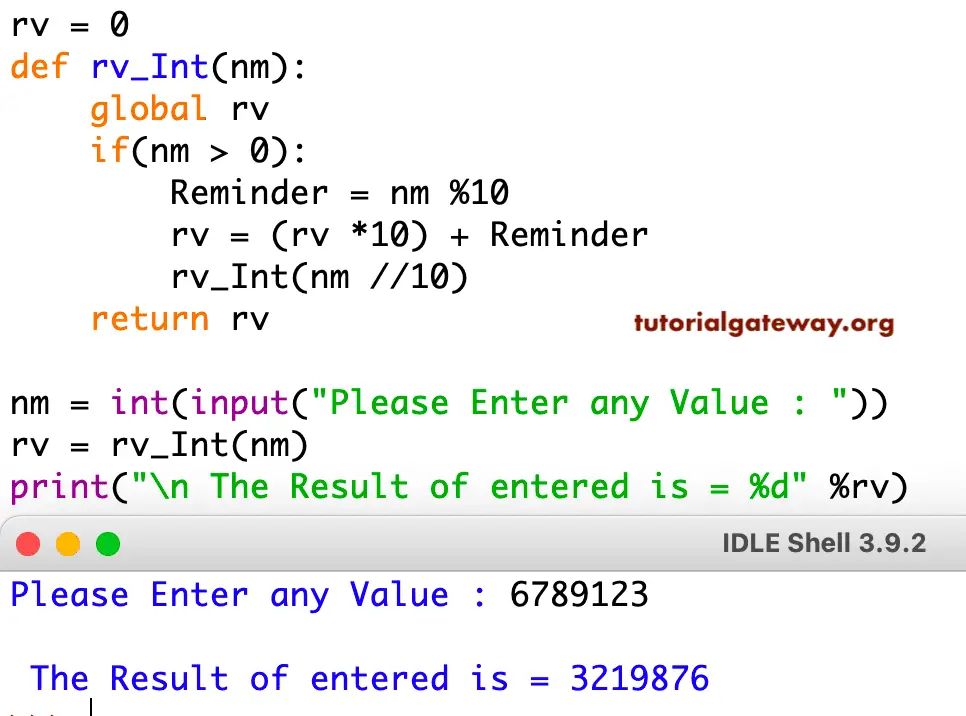
In this example, When the compiler reaches to rv = rv_Int(nm) line in the program, then the compiler immediately jumps to the below function:
def rv_Int(nm):
In this function, the statement below helps call the function Recursively with the updated value. If you miss this statement, it terminates after completing the first line.
Rerv_Int(nm //10)
For example, Number = 459 produces the output as 9
Let’s see the If Statement,
if (nm > 0), check whether the number is greater than 0 or not. For Recursive functions, placing a condition before using the function recursively is essential. Otherwise, we end up in infinite execution (Same as an infinite Loop).
Using recursion Example 2
def rev_int(n): if n < 10: return n else: return int(str(n % 10) + str(rev_int(n // 10))) num = int(input("Enter any Value = ")) rv = rev_int(num) print("The Result = ", rv)
Enter any Value = 12345
The Result = 54321
Python Program to Reverse a Number using slicing
In this example, str(num) will convert the given number to a string, and the [::-1] will slice the string and return characters in reverse. Next, the int() function will convert the reversed digits back to the integer.
num = int(input("Enter any Number: ")) rev = int(str(num)[::-1]) print("Result = ", rev)
Enter any Number: 23987
Result = 78932
Using list Comprehension
This example code uses the list comprehension and reversed function.
num = int(input("Enter any Number = ")) rv = int(''.join([str(i) for i in reversed(str(num))])) print("The Result = ", rv)
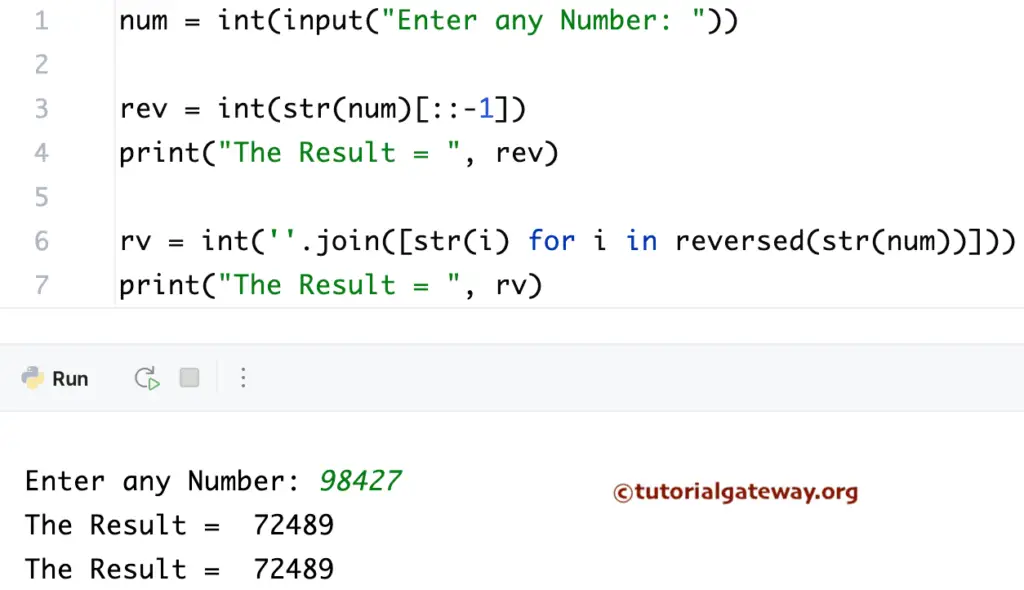
Comments are closed.