Write a Python program to perform cloning or copy one list to another. In this language, we can use the list function to clone or copy.
orgList = [10, 20, 40, 60, 70] print("Original List Items = ", orgList) print("Original List Datatype = ", type(orgList)) newList = list(orgList) print("New List Items = ", newList) print("New List Datatype = ", type(newList))
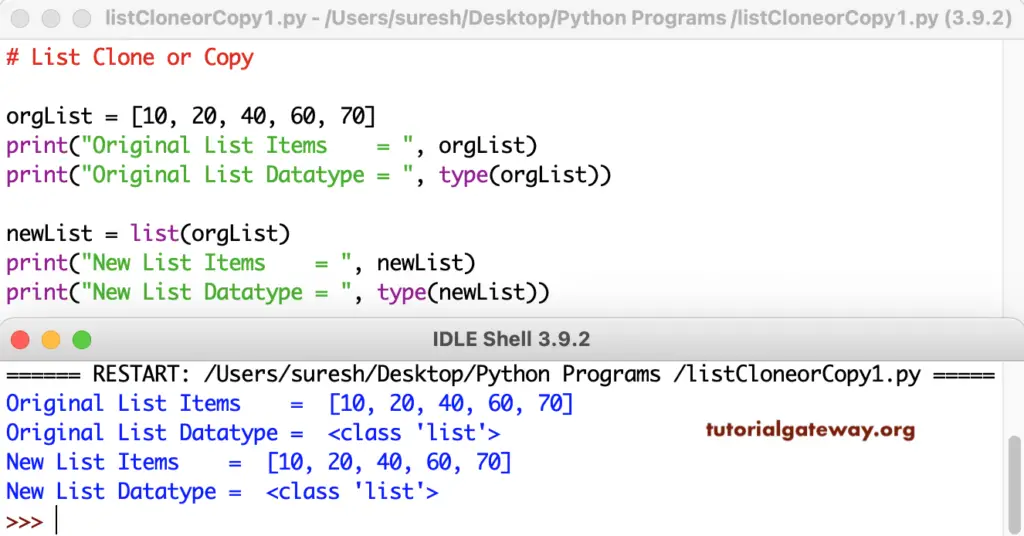
Python Program to Clone or Copy a List
In this example, we slice the list without the start and stop values, which eventually return the total items.
orgList = [40, 60, 80, 100, 120] print("Original Items = ", orgList) newList = orgList[:] print("New Items = ", newList)
Original Items = [40, 60, 80, 100, 120]
New Items = [40, 60, 80, 100, 120]
In this Program, we used the extend function to copy the list to another.
orgList = [22, 33, 44, 55, 66, 77, 88, 99] print("Original Items = ", orgList) newList = [] newList.extend(orgList) print("New Items = ", newList)
Original Items = [22, 33, 44, 55, 66, 77, 88, 99]
New Items = [22, 33, 44, 55, 66, 77, 88, 99]
This Python program uses list comprehension to clone our copy of the items.
orgList = [12, 23, 34, 45, 56, 67, 78] print("Original Items = ", orgList) newList = [lVal for lVal in orgList] print("New Items = ", newList)
copy using list comprehension output
Original Items = [12, 23, 34, 45, 56, 67, 78]
New Items = [12, 23, 34, 45, 56, 67, 78]
Python Program to Clone or Copy a List using for loop
We used the for loop (for lVal in orgList) and for loop range (for i in range(len(orgList))) to iterate the list items. Within the loops, the append function adds or appends each list item to the new list.
orgList = [25, 35, 45, 55, 65, 75, 85] print("Original Items = ", orgList) newList = [] for lVal in orgList: newList.append(lVal) print("New Items = ", newList) newList2 = [] for i in range(len(orgList)): newList2.append(orgList[i]) print("New Items = ", newList2)
Original Items = [25, 35, 45, 55, 65, 75, 85]
New Items = [25, 35, 45, 55, 65, 75, 85]
New Items = [25, 35, 45, 55, 65, 75, 85]
There is a built-in list copy function to copy or clone the list items.
orgList = [24, 44, 54, 64, 76, 85] print("Original Items = ", orgList) newList = orgList.copy() print("New Items = ", newList)
Original Items = [24, 44, 54, 64, 76, 85]
New Items = [24, 44, 54, 64, 76, 85]