The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones. This blog post will show how to write a Python program to generate the Fibonacci Series of numbers using While Loop, For Loop, and Recursion. We will also explore finding the sum of Fibonacci Series numbers using loops.
Python programming language provides several approaches to generate the Fibonacci series of numbers, each with its own benefits and concerns. By understanding and executing these Python techniques, you get the idea of which program of Fibonacci series numbers works efficiently.
Although there are several program options to generate the Fibonacci series in Python, there are two main ways you should consider.
- Iterative approach: Using for loop or while loop with the combination of the If else statement to find the following numbers in a sequence. This approach is a straightforward and efficient method.
- Recursive Function approach: This approach recursively calls the function repeatedly with the updated numbers using the math formula F(n) = F(n-1) + F(n-2).
Python Fibonacci Series
The Fibonacci sequence starts with 0 and 1 numbers. Each subsequent number is the sum of the two preceding numbers (0 + 1, 1 + 1, ….). The sequence of Fibonacci series numbers are 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, …, N. In mathematical terms, the Fibonacci sequence is defined as follows:
F(0) = 0
F(1) = 1
F(n) = F(n-1) + F(n-2)
Python Fibonacci Series program using while Loop
This program allows the user to enter any positive integer. Next, this Python program displays the Fibonacci series numbers from 0 to user-specified numbers using while Loop.
Number = int(input("\nPlease Enter the Range : ")) # Initializing First and Second Values i = 0 First_Value = 0 Second_Value = 1 # Find & Displaying while(i < Number): if(i <= 1): Next = i else: Next = First_Value + Second_Value First_Value = Second_Value Second_Value = Next print(Next) i = i + 1
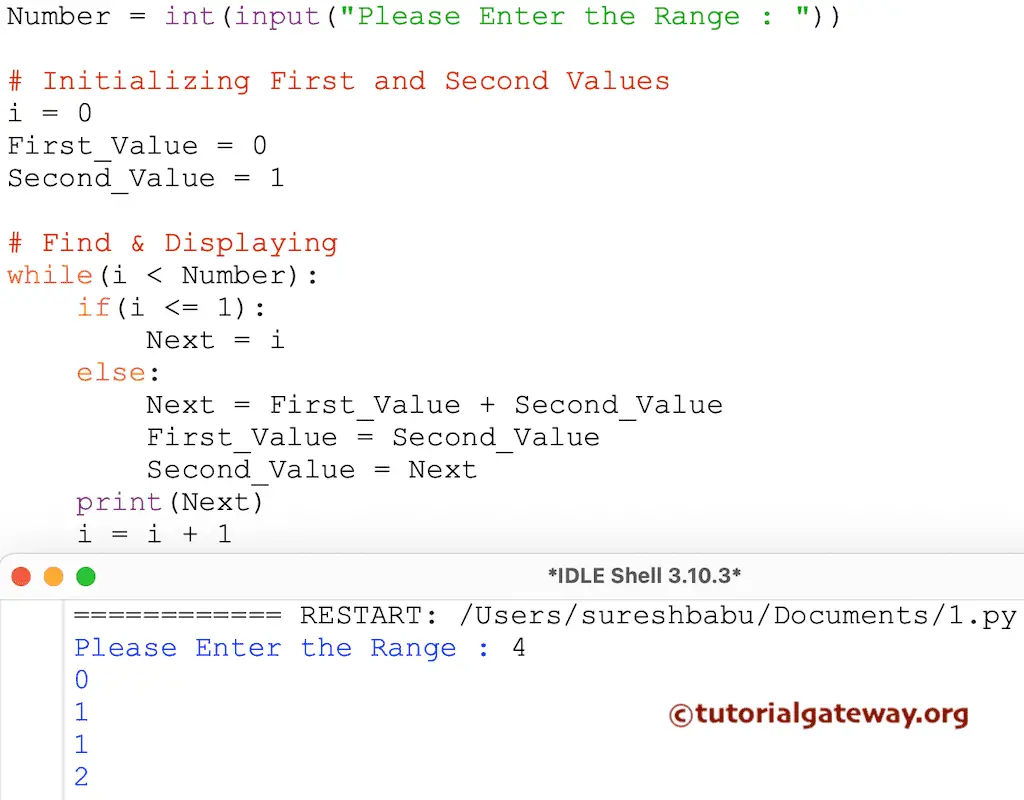
This program allows the user to enter any positive integer, which is then assigned to a variable Number. Next, We declared three integer variables i, First_Value, Second_Value, and assigned values.
The below while Loop makes sure that the loop starts from 0 and is less than the user-given number. We used the If statement within the While loop of the Python Fibonacci series program.
- If i value is less than or equal to 1, then Next = i
- If i value is greater than 1, perform calculations inside the Else block.
Let us see the working principle of this while loop in this Python Fibonacci Series example program iteration-wise. In this example, User Entered value: Number = 4 and i = 0, First_Value = 0, Second_Value = 1.
while Loop First Iteration
- While (0 < 4) is TRUE. So, the program starts executing statements inside the while.
- We have the If statement within the while loop, and the condition if (0 <= 1) is TRUE. So, Next = 0, and the compiler exit from the if statement block.
- Print statement print(Next) print the value 0.
- Lastly, i incremented to 1.
The Second Iteration of the Python Fibonacci Series program while loop.
- While (1 < 4) is TRUE.
- if (1 <= 1) is TRUE. So, Next = 1
- print(Next) print the value 1.
- i incremented to 1.
Third Iteration: While (2 < 4) is TRUE in this Python Fibonacci Series example program. The condition if (2 <= 1) is FALSE, so statements inside the else block start executing.
Next = First_Value + Second_Value
Next = 0 + 1 = 1
First_Value = Second_Value = 1
Second_Value = Next = 1
Next, Print statement print(Next) in the Python Fibonacci Series program prints the value 1. Lastly, i incremented to 1
Fourth Iteration: while (3 < 4) is TRUE. So, the program starts executing statements inside the while.
The condition if (3 <= 1) is FALSE
Next = 1 + 1 = 2
First_Value = Second_Value = 1
Second_Value = Next = 2
Next, Print statement print(Next) print the value 2. Lastly, i incremented to 1
Fifth Iteration: While (4 < 4) is FALSE, it exits from the while loop. Please refer to For Loop.
Our final output of the Next values are: 0 1 1 2
Python Fibonacci Series program using For Loop
This Python program displays the Fibonacci series of numbers from 0 to user-specified value using For Loop.
# It will start at 0 and travel upto below value Number = int(input("Please Enter the Range : ")) # Initializing First and Second Values First = 0 Second = 1 # Find & Displaying for Num in range(0, Number): if(Num <= 1): Next = Num else: Next = First + Second First = Second Second = Next print(Next)
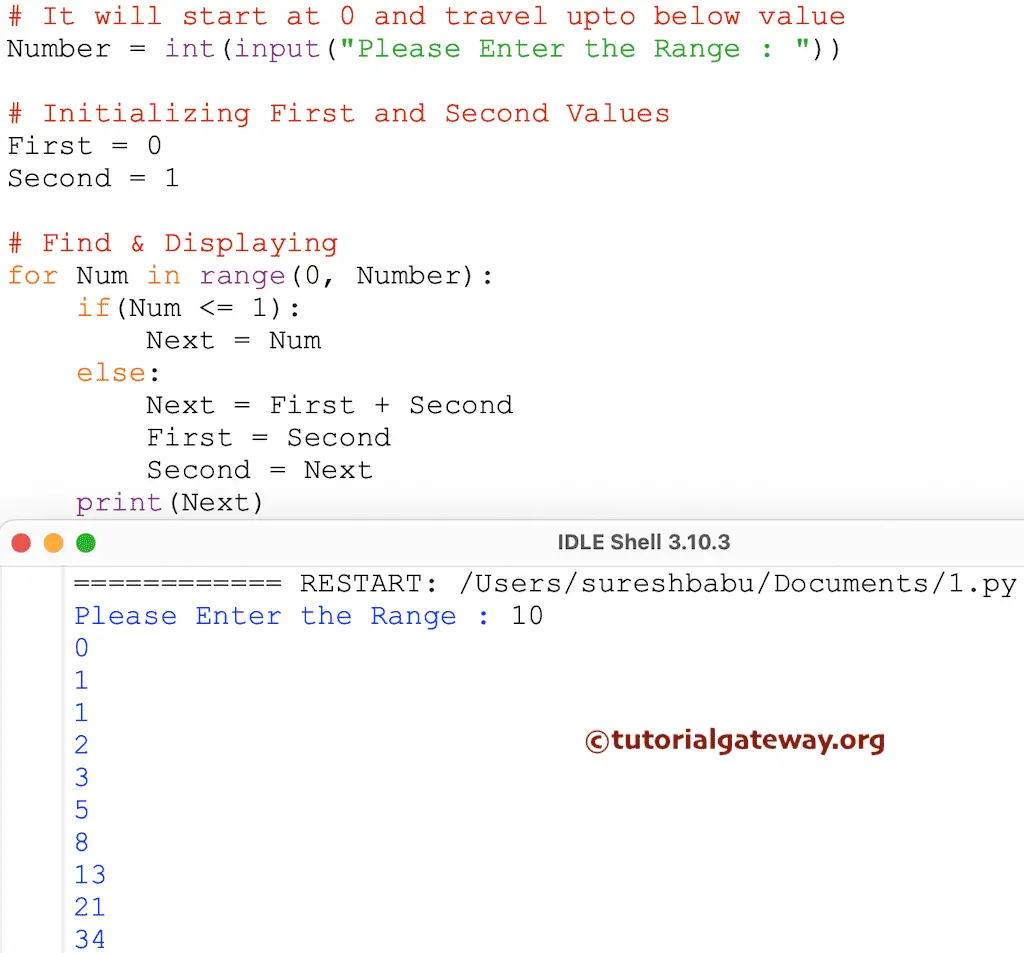
Python Program for Fibonacci Series Numbers using For Loop and List
The previous loop examples use the traditional approach to generate the Fibonacci series. However, you can utilize the Lists and avoid the If else statement. In this example, we initialized the fibSeq list of numbers 0 and 1, and the for loop iterates from 2 to a given number.
The code within the for loop calculates each Fibonacci number by adding the previous two numbers. The list append function adds the Fibonacci numbers to the fibSeq list.
Time and space complexity is O(n).
n = int(input("Enter the Range = ")) fibSeq = [0, 1] for i in range(2, n + 1): fibSeq.append(fibSeq[i-1] + fibSeq[i-2]) print(fibSeq)
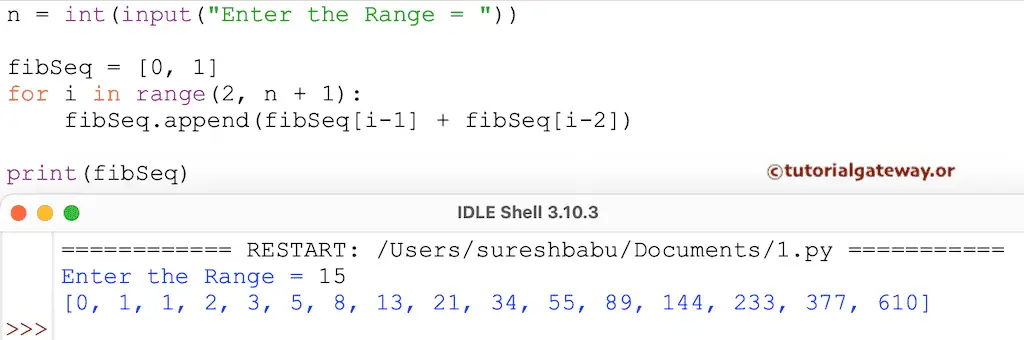
Python Fibonacci Series program using Recursion
This Python program displays the Fibonacci series of numbers from 0 to the user-given value using the Recursion concept. The recursive approach of finding Fibonacci series numbers involves defining a function with if else statements to escape from an infinite loop and call the function itself. This program uses the standard mathematical formula that we mentioned at the beginning, i.e., F(n) = F(n-1) + F(n-2).
# Recursive Function Beginning def fibFind(num): if(num == 0): return 0 elif(num == 1): return 1 else: return (fibFind(num - 2) + fibFind(num - 1)) # End of the Function # It will start at 0 and travel upto below value num = int(input("\nPlease Enter the Range Number: ")) # Find & Displaying Them for Num in range(0, num): print(fibFind(Num))
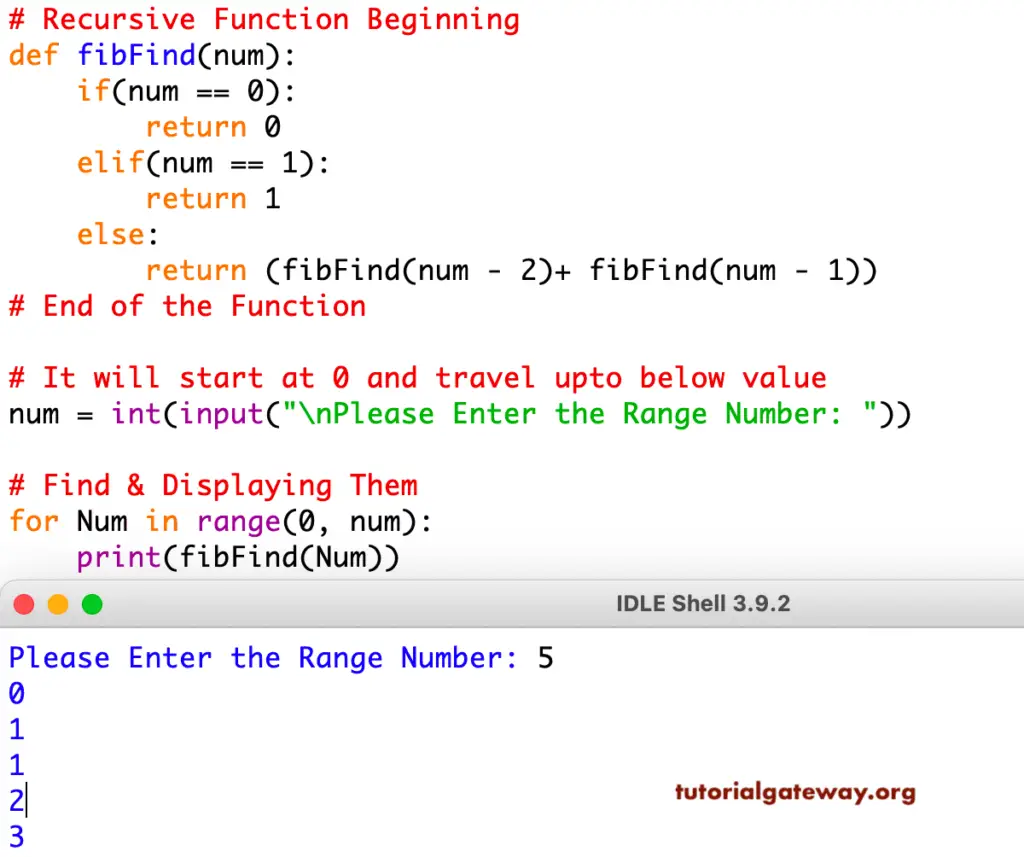
We defined a function using this Python Fibonacci Series program using the recursion example. The following function accepts integer values as parameter values and returns values.
def fibFind(num):
Let’s see the Elif statement inside the above-specified functions.
- if (num == 0) checks whether the given number is 0 or not. If it is TRUE, the function returns the value Zero.
- if(num == 1) check whether the given number is 1 or not. If it is TRUE, the function returns the value One.
- And, if the number is greater than 1, the statements inside the else block are executed.
We called the function recursively within the Else block to display the result.
return (fibFind(num-2)+ fibFind(num-1))
For the demonstration of the program using recursion, Number= 2
fibFind(num-2)+ fibFind(num-1)
fibFind(2 – 2)+ fibFind(2 – 1)
It means, (fibFind(0)+ fibFind(1))
return (0 + 1) = return 1.
Time and space complexity is O(2^n) and O(n).
Python Program to Find the Sum of Fibonacci Series Numbers
Write a program to find the sum of Fibonacci numbers using for loop. In this example, we used for loop to iterate from zero to n and find the sum of all the numbers within that range.
Number = int(input("Please Enter the Fibonacci Number Range = ")) First = 0 Second = 1 Sum = 0 for Num in range(0, Number): print(First, end = ' ') Sum = Sum + First Next = First + Second First = Second Second = Next print("\nThe Sum of Fibonacci Series Numbers = %d" %Sum)
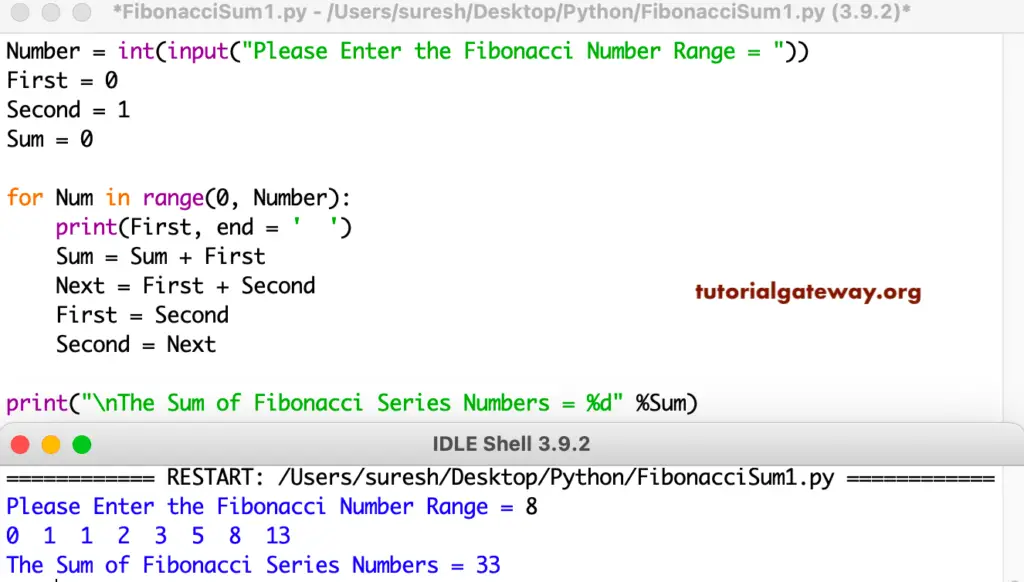
Using while loop
This program finds the sum of Fibonacci Series numbers using a while loop.
Number = int(input("Please Enter the Range = ")) First = 0 Second = 1 Sum = 0 i = 0 while(i < Number): print(First, end = ' ') Sum = Sum + First Next = First + Second First = Second Second = Next i = i + 1 print("\nThe Sum = %d" %Sum)
Please Enter the Range = 24
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987 1597 2584 4181 6765 10946 17711 28657
The Sum = 75024
Using Recursion
This program to find the sum of all the Fibonacci Series numbers using recursion or recursive functions.
def fibonacci(Number): if(Number == 0): return 0 elif Number == 1: return 1 else: return fibonacci(Number - 2) + fibonacci(Number - 1) Number = int(input("Please Enter the Range = ")) Sum = 0 for Num in range(Number): print(fibonacci(Num), end = ' ') Sum = Sum + fibonacci(Num) print("\nThe Sum = %d" %Sum)
Please Enter the Range = 30
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987 1597 2584 4181 6765 10946 17711 28657 46368 75025 121393 196418 317811 514229
The Sum = 1346268
Comments are closed.