The Python If statement is one of the most useful decisions making statements in real-time programming. The If statement allows the compiler to test the condition first, and depending upon the result, it executes the code block. While a given test condition is true, then only the code within the if block executes.
The Python If Else Statement is an extension to the If (which we discussed in the earlier post). If condition only executes the code block when the given condition is true and when the condition is false, it will not execute the code.
In the real world, it would be nice to execute something when the expression fails. To do so, the Python If condition is used, and here, the Else block will run some code when the condition fails.
Python If Statement Syntax
The if statement in Python Programming has a simple structure:
if (test condition): Statement2 Statement3 …………. …………. Statementn
When the test condition in the If statement is true, Statement1, Statement2, ……., Statementn will execute. Otherwise, all too them will skip. Let us see the flow chart for a better understanding.
Python If Statement Flow Chart
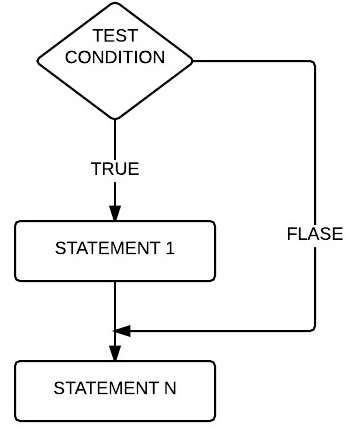
While the test condition is true, STATEMENT1 is executed, followed by STATEMENTN. If it is False, STATEMENTN executes. Because it’s out of the if block and has nothing to do with the result.
Python If Statement Example
This program will check for the positive number using the Python if statement. First, please open your favorite IDLE to write the script, and here we are using 3.5.0. Once you open IDLE, Please select the New File as shown below, or else click Control + N
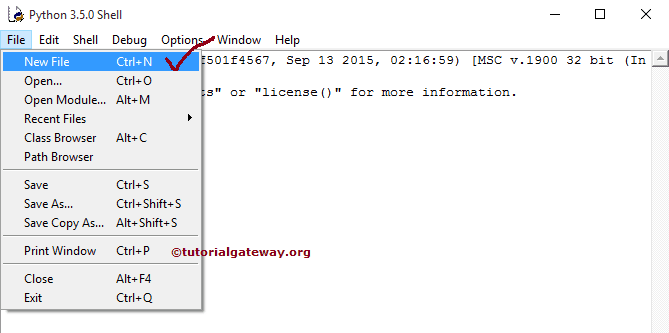
Once you click on the New File, a new file window will open to write a script for Python If statement example. Please add the below script in that new file.
# Example number = int(input(" Please Enter any integer Value: ")) if number >= 1: print(" You Have Entered Positive Integer ")
Once completed, Click on the File, and select the Save option. Please save the file as per you want.
Let’s run the script by selecting Run Menu and clicking the Run Module or pressing F5.
Click the Run Module will pop up a Shell with the message, “Please Enter any integer Value:”. We entered 20 and it is a positive integer.
Please Enter any integer Value: 20
You Have Entered Positive Integer
First, we declared a number variable and asked the user to enter any integer value. int() restricts the user not to enter non-integer values.
number = int(input(" Please Enter any integer Value: "))
When you look at the below Python if statement, the Value stored in the number variable is greater than or equal to 0, then the line will be executed.
if number >= 1: print(" You Have Entered Positive Integer ")
Here we entered 20, which is greater than 0 that’s why it printed the lines inside the If statement block.
Python If Statement Example 2
In this Python if statement example, we will show you what happens to the code outside the If block by altering example 1.
# Example number = int(input(" Please Enter any integer Value: ")) if number >= 1: print(" You Have Entered Positive Integer ") print(" This Message is not coming from IF STATEMENT")
This Python If statement code is the same code that we used in the first example. However, this time, we added one more print outside the If block with the message.
Please Enter any integer Value: 20
You Have Entered Positive Integer
This Message is not coming from IF STATEMENT
We entered 50, which means the condition is TRUE. So, it is displaying the print function inside the If statement and Outside the If block.
Please Enter any integer Value: 50
You Have Entered Positive Integer
This Message is not coming from IF STATEMENT
Let’s try the negative values to intentionally fail the condition. While the condition failed here (number < 1), the compiler prints nothing from the If condition block. So, it printed only one print function, which is outside the block.
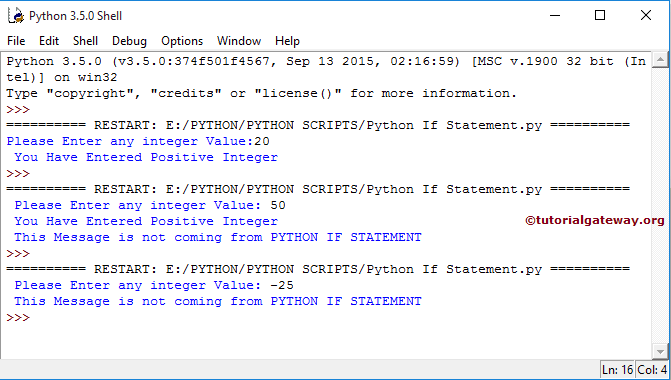
Python If Else Syntax
The syntax of the Python If Else Statement is
if (Test condition): # The condition is TRUE then these lines will be printed True codes else # When the condition evaluates to FALSE then these lines will print False codes
When the test condition present in the above Python If else structure is evaluated to true, True statements are executed. When it returns false, a False code is executed.
If Else Statement Example
In this Python If Else statement program, we will place 4 different lines. When the condition is met true, it will display 2 distinct lines. When the conditional expression evaluates to false, we will show the other 2 statements using this else block code.
# Example marks = int(input(" Please Enter Your Subject Marks: ")) if marks >= 50: print(" Congratulations ") #s1 print(" You cleared the subject ") #s2 else: print(" You Failed") #s3 print(" Better Luck Next Time") #s4
Please save this file and run the script file by pressing F5.
We enter 60 as marks for the demo purpose, which is greater than 50. That’s why the Python if else program printed (s1 and s2 statements) inside the If block.
Please Enter Your Subject Marks: 60
Congratulations
You cleared the subject
We entered 30 as marks. It means the Condition is FALSE, so s3 and s4 inside the else block will print.
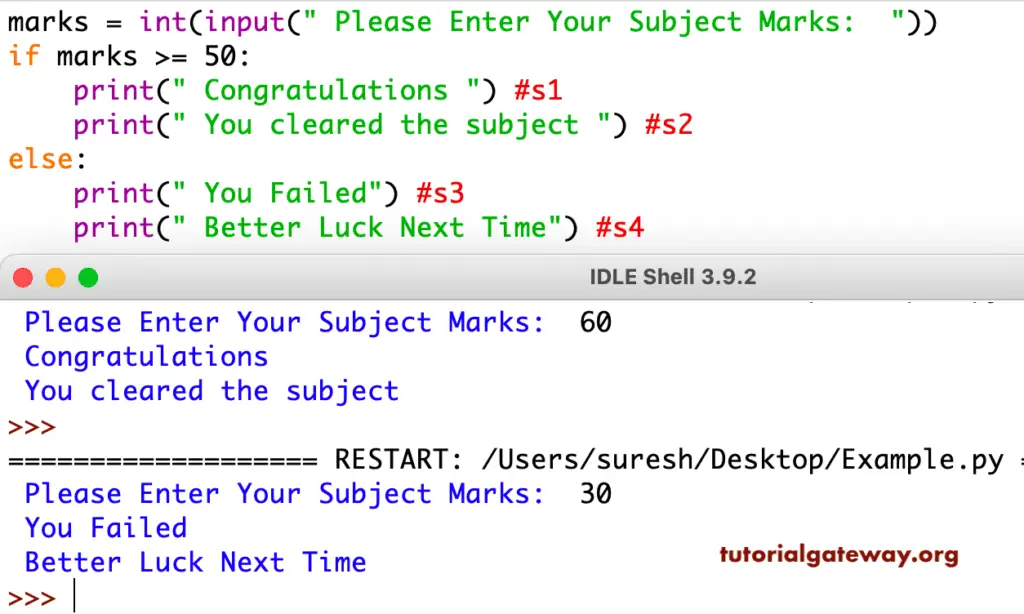
First, we ask the user to enter marks. int() restricts the user not to enter non-integer values.
When you look at the Python if else statement example, the Value stored in the marks variable is greater than or equal to 50, then the following print lines will execute. If it is less than 50, the below code inside Else will execute.