The Python String is nothing but a sequence of characters. This section discusses how to create a String, access the individual characters (items), and iterate the characters. Also, show you slicing String in Python programming language.
Create a Python String
There are multiple ways to create a string in Python. This gives you an idea to create it.
# Create using Single Quote Str1 = 'This is a message' print(Str1) # Create using Double Quote Str2 = "This is another with Double Quotes" print(Str2) # Create using Multiple Single Quotes Str3 = '''This also Work''' print(Str3) # Create it using Triple Quotes # This is Very Useful to create Multiline Text Str4 = """Learn Programming at Tutorial Gateway""" print(Str4)
This is a message
This is another with Double Quotes
This also Work
Learn Programming
at Tutorial Gateway
Access Python String items
We can access the elements in a String using indexes. Using an index, we can access each item separately. The index value starts at 0 and ends at n-1, where n is the length. For example, if the length is 5, the index starts at 0 and ends at 4. To access the 1st value, use the name[0], and to access the 5th value, then use the name[4].
x = 'Tutorial Gateway' # Positive Indexing print(x[0]) print(x[4]) print(x[9]) print('=======\n') # Negative print(x[-3]) print(x[-7]) print(x[-16]) print('=======\n')
If you are using the Negative index numbers, it starts looking for items from right to left (Here, -1 is the Last Item, -2 is Last but one, etc.).
T
r
G
=======
w
G
T
=======
Iterate String
The Python For Loop is the most common way to traverse the characters or items in a string. This code helps us to iterate the str, and prints each character present in the str.
TIP: Please refer to For Loop and Functions.
Str = "Python" for word in Str: print("Letters are: ", word)
The above-specified code works nicely to print the characters inside it. However, to change the individual item, we need the Python index position also. To fix this, we have to use the range function along with for loop
for word in range(len(Str)): print("Letters at index {0} is = {1}".format(word, Str[word]))
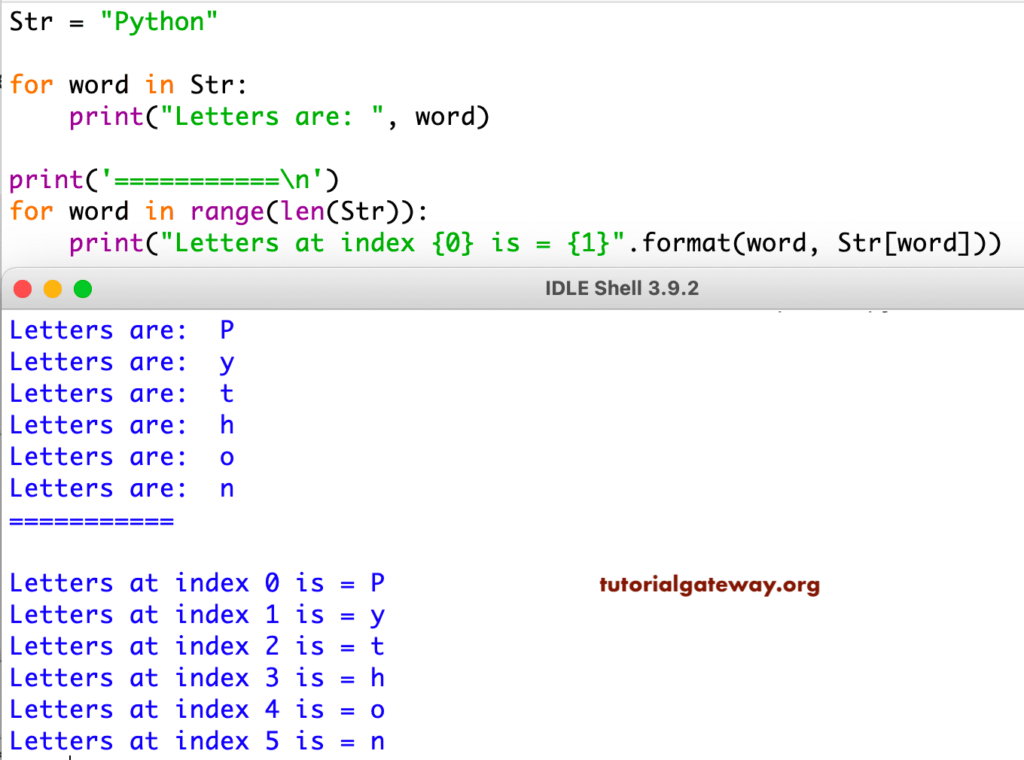
string concatenation
Joining or combining more than one is called the Python string concatenation or concat. There are multiple ways to concat.
- We can use the + operator to join more than one.
- Placing more than one literal joins them automatically.
- Placing more than one inside the one and closed parentheses ()
- * operator is repeating the sentence for a given number of times. Here it is three times.
In this Python example, we show you how to use Arithmetic Operators on String to perform arithmetic Operations.
X = 'Tutorial ' Y = 'Gateway' # Using + Operator Concat = X + Y Concat1 = 'This ' + X # Placing two Literals Together Concat2 = 'Tutorial ' 'Gateway' #Using Parentheses Concat3 = ('Tutorial ' 'Gateway') print(Concat) print(Concat1) print(Concat2) print(Concat3) # Using * Operator Z = X * 3 print(Z)
Tutorial Gateway
This Tutorial
Tutorial Gateway
Tutorial Gateway
Tutorial Tutorial Tutorial
Python String Slice
In String Slice, the first integer value is the index position where the slicing start, and the second is where the slicing end, but it does not include the content at this index position. For instance, if we define stritem[1:4], then string slicing starts at index position 1 and ends at index position 3 (not 4).
x = 'Tutorial Gateway' # Using two a = x[2:13] print(a) # Using Second b = x[:8] print(b) # Slice using First c = x[4:] print(c) # without using two d = x[:] print(d) # Slice using Negative first e = x[-3:] print(e) # Slice using Negative second f = x[:-2] print(f)
torial Gate
Tutorial
rial Gateway
Tutorial Gateway
way
Tutorial Gatew
Python string slice analysis
- If you omit the first index, the slicing starts from the beginning.
- If you omit the second argument, Slicing starts from the first position and continues to the last.
- And, If you are using the Negative numbers as an index, the slicing starts from right to left.