This article shows how to write a Python program to print the Alphabet L star pattern using the for loop, while loop, and functions with an example.
The below alphabet L star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The If else condition is to print stars at the first column and last row to get the Alphabetical L pattern of stars and skip others.
rows = int(input("Enter Alphabet L of Stars Rows = ")) print("====The Alphabet L Star Pattern====") for i in range(rows): print("*", end="") for j in range(rows + 1): if i == rows - 1: print("*", end="") else: print(end=" ") print()
Enter Alphabet L of Stars Rows = 8
====The Alphabet L Star Pattern====
*
*
*
*
*
*
*
**********
This Python code is another version of writing the Alphabetical L pattern of stars.
rows = int(input("Enter Alphabet L of Stars Rows = ")) print("====The Alphabet L Star Pattern====") for i in range(rows): for j in range(rows + 1): if i == rows - 1 or j == 0: print("*", end="") else: print(end=" ") print()
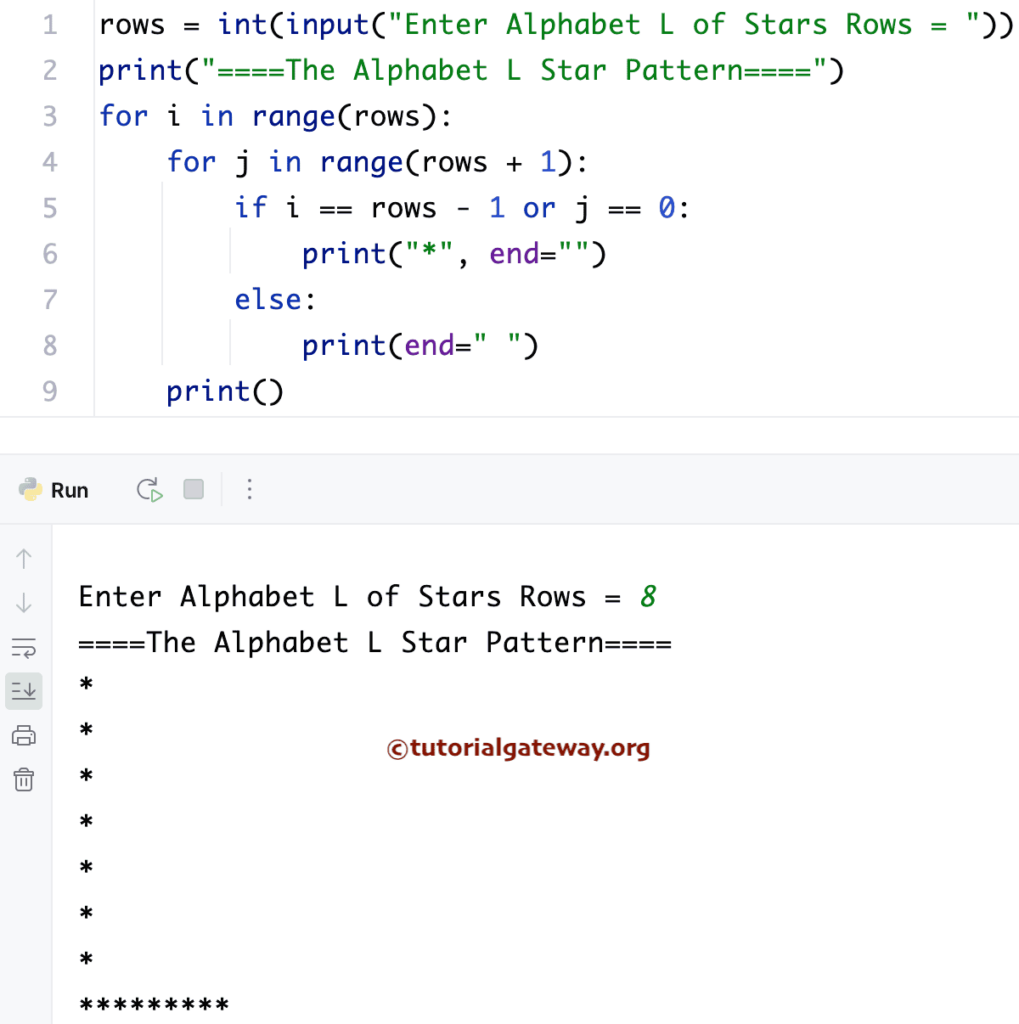
Python program to print the Alphabet L Star pattern using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet L pattern rows and prints the stars at the first column and last row position. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet L of Stars Rows = ")) i = 0 while i < rows: j = 0 while j < rows + 1: if i == rows - 1 or j == 0: print("*", end="") else: print(end=" ") j = j + 1 print() i += 1
Enter Alphabet L of Stars Rows = 13
*
*
*
*
*
*
*
*
*
*
*
*
**************
In this Python example, we created an LPattern function that accepts the rows and the symbol or character to print the Alphabet L pattern of the given symbol.
def LPattern(rows, ch): for i in range(rows): for j in range(rows + 1): if i == rows - 1 or j == 0: print('%c' %ch, end='') else: print(end=" ") print() row = int(input("Enter Alphabet L of Stars Rows = ")) sy = input("Symbol for L Star Pattern = ") LPattern(row, sy)
Enter Alphabet L of Stars Rows = 11
Symbol for L Star Pattern = ^
^
^
^
^
^
^
^
^
^
^
^^^^^^^^^^^^