This article shows how to write a Python program to print the Alphabet A star pattern using the for loop, while loop, and functions with an example.
The below alphabet A star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The If else condition is to print stars at a few positions, get the Alphabet A pattern of stars, and skip others.
rows = int(input("Enter Alphabet A of Stars Rows = ")) for i in range(rows): for j in range(rows // 2 + 1): if (i == 0 and j != 0 and j != rows // 2) or i == rows // 2 or (j == 0 or j == rows // 2) and i != 0: print('*', end='') else: print(end=' ') print()
Enter Alphabet A of Stars Rows = 9
***
* *
* *
* *
*****
* *
* *
* *
* *
The above example looks good; however, we must modify the program slightly to get the perfect Alphabet A star pattern.
rows = int(input("Enter Alphabet A of Stars Rows = ")) print("====The Alphabet A Star Pattern====") n = rows for i in range(rows): for j in range(2 * rows): if j == n or j == ((2 * rows) - n) or (i == (rows // 2) and n < j < ((2 * rows) - n)): print('*', end='') else: print(end=' ') print() n = n - 1
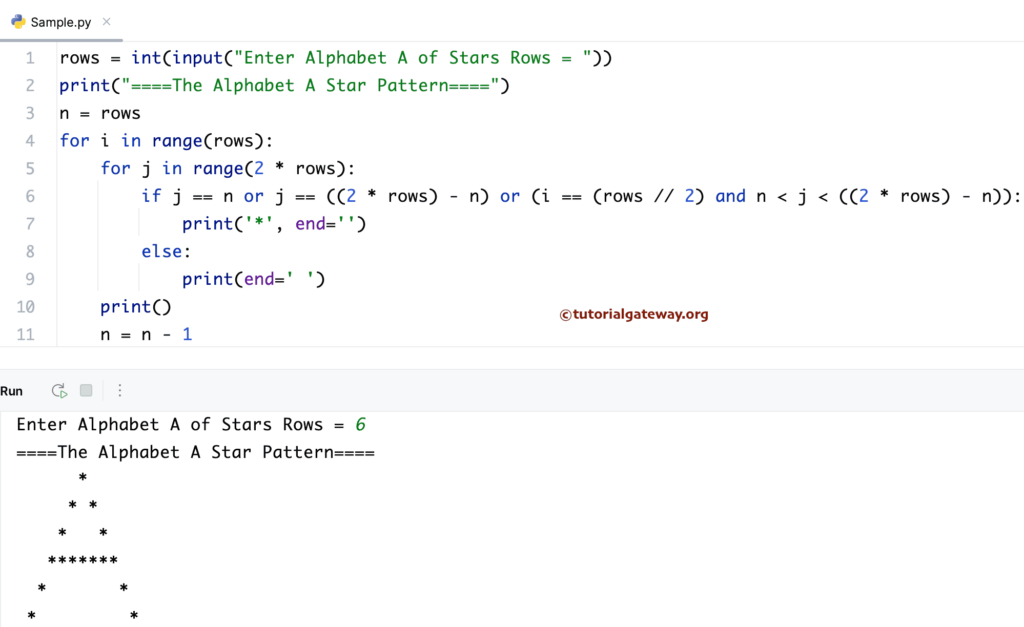
Python Program to print the Alphabet A Star pattern using a while loop
Instead of a For loop, this program uses the while loop to iterate the A pattern rows and prints the stars at each position. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet A of Stars Rows = ")) n = rows i = 0 while i < rows: j = 0 while j < 2 * rows: if j == n or j == ((2 * rows) - n) or (i == (rows // 2) and n < j < ((2 * rows) - n)): print('*', end='') else: print(end=' ') j = j + 1 print() i = i + 1 n = n - 1
Enter Alphabet A of Stars Rows = 10
*
* *
* *
* *
* *
***********
* *
* *
* *
* *
In this Python example, we created an APattern function that accepts the rows and the symbol or character to print the Alphabet A pattern of the given symbol.
def APattern(rows, ch): n = rows for i in range(rows): for j in range(2 * rows): if j == n or j == ((2 * rows) - n) or (i == (rows // 2) and n < j < ((2 * rows) - n)): print('%c' %ch, end='') else: print(end=' ') print() n = n - 1 row = int(input("Enter Alphabet A of Stars Rows = ")) sym = input("Symbol for A Pattern = ") APattern(row, sym)
Enter Alphabet A of Stars Rows = 9
Symbol for A Pattern = $
$
$ $
$ $
$ $
$$$$$$$$$
$ $
$ $
$ $
$ $