This article shows how to write a Python program to print the Alphabet C star pattern using the for loop, while loop, and functions with an example.
The below alphabet C star pattern example accepts the user-entered rows and the nested for loop iterates the rows. The If else condition is to print stars at the first and last positions to get the Alphabet C pattern of stars and skip others.
rows = int(input("Enter Alphabet C of Stars Rows = ")) print("====The Alphabet C Star Pattern====") for i in range(rows): print('*', end='') for j in range(rows - 1): if i == 0 or i == rows - 1: print('*', end='') else: continue print()
Enter Alphabet C of Stars Rows = 8
====The Alphabet C Star Pattern====
********
*
*
*
*
*
*
********
The above generates the Alphabet C, but you can also try the code below; it looks better than the above.
rows = int(input("Enter Alphabet C of Stars Rows = ")) print("====The Alphabet C Star Pattern====") if rows < 3: print("The letter C is Not visible for this Number.") n = rows // 2 + 1 print(" " + "*" * n) for i in range(rows // 2): print("*" + " " * (n - 1)) print(" " + "*" * n)
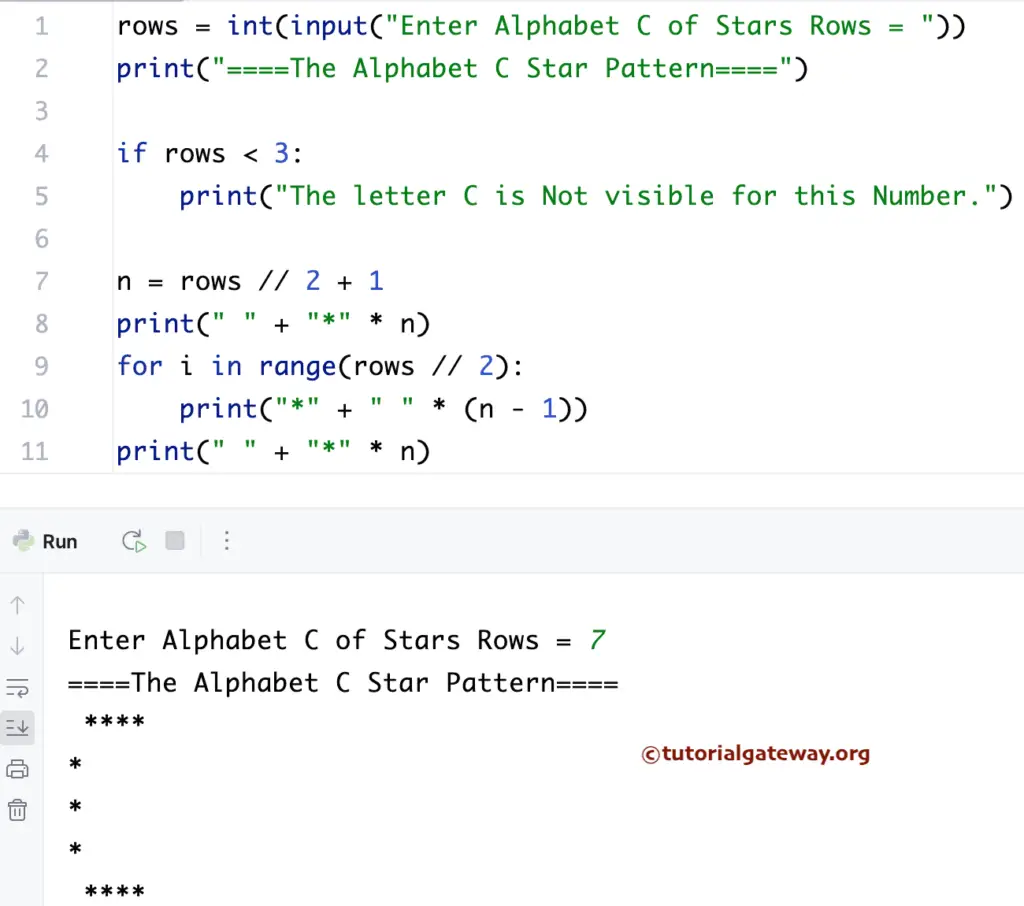
Python program to print the Alphabet C Star pattern using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet C pattern rows. Next, it prints the stars at each position. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet C of Stars Rows = ")) if rows < 3: print("The letter C is Not visible for this Number.") n = rows // 2 + 1 print(" " + "*" * n) i = 0 while i < (rows // 2): print("*" + " " * (n - 1)) i = i + 1 print(" " + "*" * n)
Enter Alphabet C of Stars Rows = 12
*******
*
*
*
*
*
*
*******
In this example, we created a CPattern function that accepts the rows and the symbol or character. Next, it print the Alphabet C pattern of the given symbol. It is a slightly modified version of the above for loop example.
def CPattern(rows, ch): if rows < 3: print("The letter C is Not visible for this Number.") n = rows - 1 print(" " + '%c' %ch * n) for _ in range(rows // 2): print('%c' %ch) print(" " + '%c' %ch * n) row = int(input("Enter Alphabet C of Stars Rows = ")) sym = input("Symbol for C Star Pattern = ") CPattern(row, sym)
Enter Alphabet C of Stars Rows = 10
Symbol for C Star Pattern = #
#########
#
#
#
#
#
#########