This article shows how to write a Python program to print the Alphabet S star pattern using the for loop, while loop, and functions with an example.
The below alphabet S star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The elif condition is to print stars at the first, middle, and last rows, half of the first and last columns to get the alphabetical S pattern of stars, and skip others.
rows = int(input("Enter Alphabet S of Stars Rows = ")) print("====The Alphabet S Star Pattern====") for i in range(rows): for j in range(rows): if i == 0 or i == rows // 2 or i == rows - 1: print("*", end=" ") elif (j == 0 and i < rows // 2) or (j == rows - 1 and i > rows // 2): print("*", end=" ") else: print(" ",end=" ") print()
Enter Alphabet S of Stars Rows = 9
====The Alphabet S Star Pattern====
* * * * * * * * *
*
*
*
* * * * * * * * *
*
*
*
* * * * * * * * *
The above code prints the boxy S. However the below Python code will print more circular style of Alphabet S pattern of stars.
rows = int(input("Enter Alphabet S of Stars Rows = ")) print("====The Alphabet S Star Pattern====") for i in range(rows): for j in range(rows): if (i == 0 or i == rows // 2 or i == rows - 1) and j != 0 and j != rows - 1: print("*", end=" ") elif i != 0 and j == 0 and i < rows // 2: print("*", end=" ") elif j == rows - 1 and i > rows // 2 and i != rows - 1: print("*", end=" ") else: print(" ", end=" ") print()
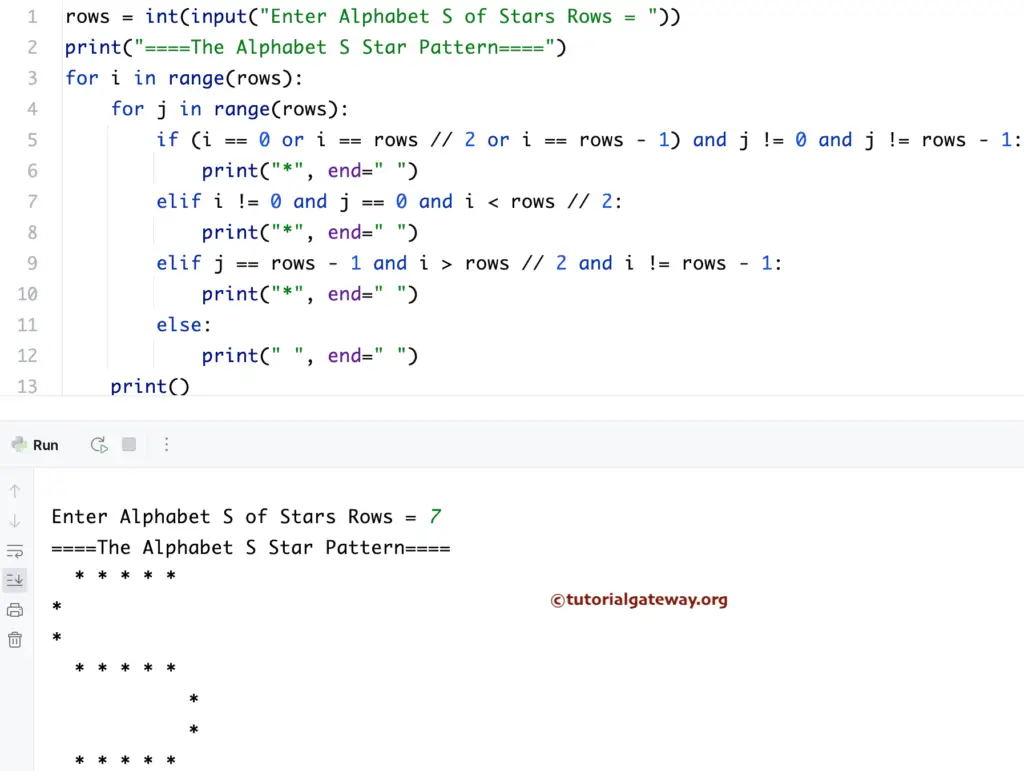
Python program to print the Alphabet S Star pattern using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet S pattern rows and prints the stars at the required locations. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet S of Stars Rows = ")) i = 0 while i < rows: j = 0 while j < rows: if i == 0 or i == rows // 2 or i == rows - 1: print("*", end=" ") elif (j == 0 and i < rows // 2) or (j == rows - 1 and i > rows // 2): print("*", end=" ") else: print(" ",end=" ") j += 1 print() i += 1
Enter Alphabet S of Stars Rows = 11
* * * * * * * * * * *
*
*
*
*
* * * * * * * * * * *
*
*
*
*
* * * * * * * * * * *
In this Python example, we created a SPattern function that accepts the rows and the symbol or character to print the Alphabet S pattern of the given symbol. It is same as the second example but we replaced the j != rows – 1 with j != rows and j != 0 with j != -1 to see the S with proper endings.
def SPattern(rows, ch): for i in range(rows): for j in range(rows): if i == 0 and j != 0 and j != rows: print('%c' % ch, end=" ") elif i != 0 and j == 0 and i < rows // 2: print('%c' %ch, end=" ") elif i == rows // 2 and j != 0 and j != rows - 1: print('%c' %ch, end=" ") elif j == rows - 1 and i > rows // 2 and i != rows - 1: print('%c' %ch, end=" ") elif i == rows - 1 and j != -1 and j != rows - 1: print('%c' %ch, end=" ") else: print(" ", end=" ") print() row = int(input("Enter Alphabet S of Stars Rows = ")) sy = input("Symbol for S Star Pattern = ") SPattern(row, sy)
Enter Alphabet S of Stars Rows = 12
Symbol for S Star Pattern = #
# # # # # # # # # # #
#
#
#
#
#
# # # # # # # # # #
#
#
#
#
# # # # # # # # # # #