This article shows how to write a Python program to print the Alphabet M star pattern using the for loop, while loop, and functions with an example.
The below alphabet M star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The If else condition is to print stars at the first column and last row to get the Alphabetical M pattern of stars and skip others.
rows = int(input("Enter Alphabet M of Stars Rows = ")) print("====The Alphabet M Star Pattern====") for i in range(rows): print("*", end="") for j in range(rows + 1): if j == rows or (j == i - 1 and j < rows // 2) or (j == rows - i and j > rows // 2): print("*", end="") else: print(end=" ") print()
Enter Alphabet M of Stars Rows = 9
====The Alphabet M Star Pattern====
* *
** **
* * * *
* * * *
* * * *
* *
* *
* *
* *
If you want the extra * for the odd numbers of the M pattern, use the code below on top of the for loop.
if rows % 2 != 0: rows += 1
This Python code is another version of writing the Alphabetical M pattern of stars.
rows = int(input("Enter Alphabet M of Stars Rows = ")) print("====The Alphabet M Star Pattern====") for i in range(rows): print("*", end="") for j in range(rows + 1): if j == rows or (j == i and j < rows // 2) or (j == rows - i - 1 and j > rows // 2): print("*", end="") else: print(end=" ") print()
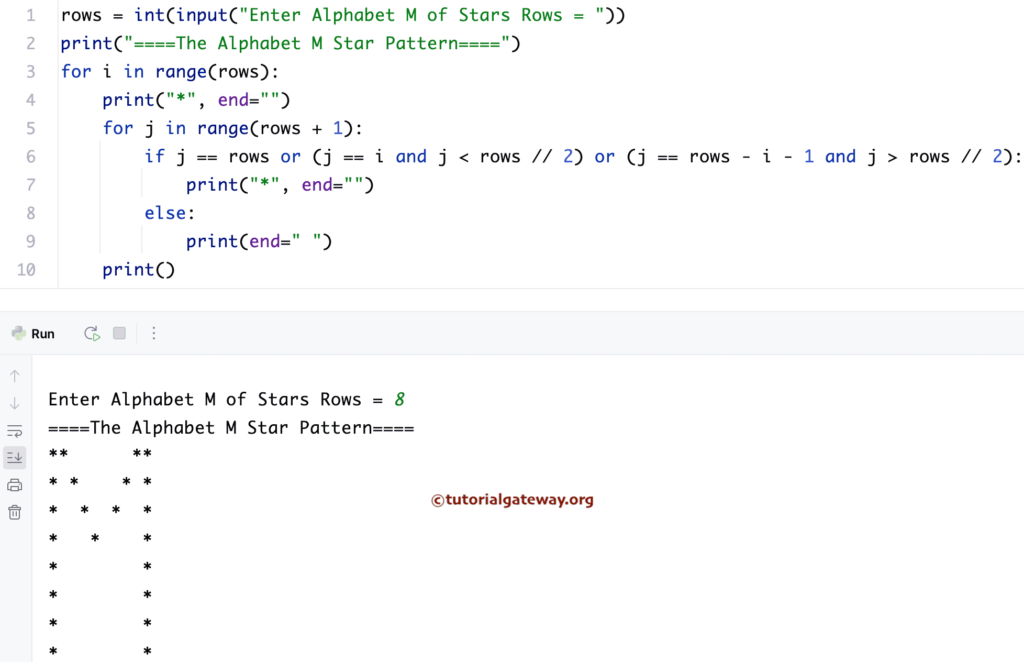
Python program to print the Alphabet M Star pattern using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet M pattern rows and prints the stars at the required positions. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet M of Stars Rows = ")) i = 0 while i < rows: print("*", end="") j = 0 while j < rows + 1: if j == rows or (j == i and j < rows // 2) or (j == rows - i - 1 and j > rows // 2): print("*", end="") else: print(end=" ") j = j + 1 print() i = i + 1
Enter Alphabet M of Stars Rows = 11
** **
* * * *
* * * *
* * * *
* * * *
* *
* *
* *
* *
* *
* *
In this Python example, we created an MPattern function that accepts the rows and the symbol or character to print the Alphabet M pattern of the given symbol.
def MPattern(rows, ch): for i in range(rows): print('%c' %ch, end='') for j in range(rows + 1): if j == rows or (j == i and j < rows // 2) or (j == rows - i - 1 and j > rows // 2): print('%c' %ch, end='') else: print(end=" ") print() row = int(input("Enter Alphabet M of Stars Rows = ")) sy = input("Symbol for M Star Pattern = ") MPattern(row, sy)
Enter Alphabet M of Stars Rows = 14
Symbol for M Star Pattern = $
$$ $$
$ $ $ $
$ $ $ $
$ $ $ $
$ $ $ $
$ $ $ $
$ $ $
$ $
$ $
$ $
$ $
$ $
$ $
$ $