This article shows how to write a Python program to print the Alphabet W star pattern using the for loop, while loop, and functions with an example.
The below alphabet W star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The If else condition is to print stars at the required positions to get the Alphabetical W pattern of stars and skip others.
rows = int(input("Enter Alphabet W of Stars Rows = ")) print("====The Alphabetical W Star Pattern====") for i in range(rows): for j in range(rows): if j == 0 or j == rows - 1: print("*", end=" ") elif (i + j == rows - 1 or i == j) and i >= rows // 2: print("*", end=" ") else: print(" ", end=" ") print()
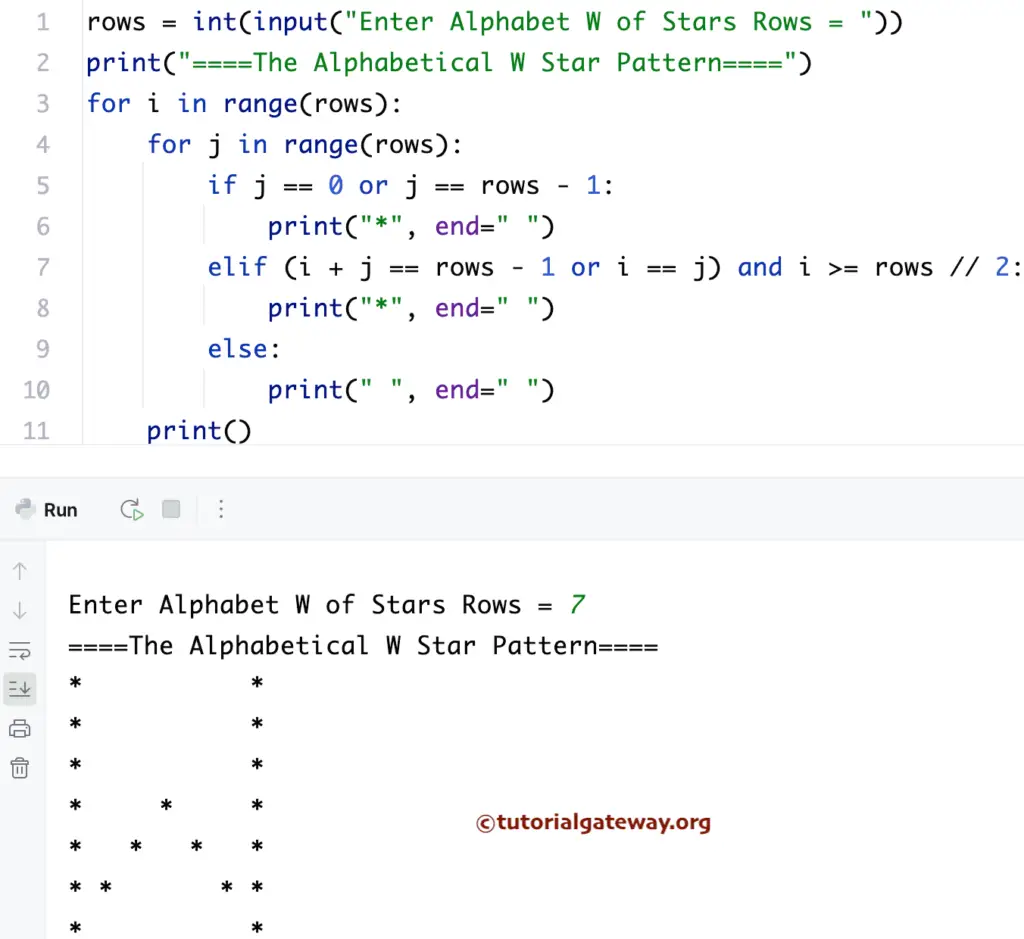
Python program to print the Alphabet W pattern of Stars using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet W pattern rows and prints the stars at a few positions. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet W of Stars Rows = ")) i = 0 while i < rows: j = 0 while j < rows: if j == 0 or j == rows - 1: print("*", end="") elif (i + j == rows - 1 or i == j) and i >= rows // 2: print("*", end="") else: print("", end=" ") j += 1 print() i += 1
Enter Alphabet W of Stars Rows = 10
* *
* *
* *
* *
* *
* ** *
* * * *
* * * *
** **
* *
In this Python example, we created a WPattern function that accepts the rows and the symbol or character to print the Alphabet V pattern of the given symbol.
def WPattern(rows, ch): for i in range(rows): for j in range(rows): if j == 0 or j == rows - 1: print('%c' %ch, end=' ') elif (i + j == rows - 1 or i == j) and i >= rows // 2: print('%c' %ch, end=' ') else: print(" ", end=" ") print() row = int(input("Enter Alphabet W of Stars Rows = ")) sy = input("Symbol for W Star Pattern = ") WPattern(row * 2 + 1, sy)
Enter Alphabet W of Stars Rows = 8
Symbol for W Star Pattern = @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @ @
@ @ @ @
@ @ @ @
@ @ @ @
@ @ @ @
@ @ @ @
@ @ @ @
@ @ @ @
@ @