This article shows how to write a Python program to print the Alphabet G star pattern using the for loop, while loop, and functions with an example.
The below alphabet G star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The elif condition is to print stars at a few positions to get the Alphabet G pattern of stars and skip others.
rows = int(input("Enter Alphabet G of Stars Rows = ")) print("====The Alphabet G Star Pattern====") for i in range(rows): for j in range(2 * rows): if (i == 0 or i == rows - 1) and (j == 0 or j == (2 * rows) - 2): print(end=" ") elif j == 0 or (i == 0 and j <= rows) or (i == rows // 2 and j > rows // 2) or \ (i > rows // 2 and j == (2 * rows) - 1) or (i == rows - 1 and j < 2 * rows): print("*", end="") else: print(end=" ") print()
Enter Alphabet G of Stars Rows = 9
====The Alphabet G Star Pattern====
*********
*
*
*
* *************
* *
* *
* *
*************** *
The above Python code prints the Alphabet G pattern of stars; the code below is another version with an incline.
rows = int(input("Enter Alphabet G of Stars Rows = ")) print("====The Alphabet G Star Pattern====") for i in range(rows): for j in range((2 * rows) - 1): if (i == 0 or i == rows - 1) and (j == 0 or j == (2 * rows) - 3): print(end=" ") elif j == 0 or (i == 0 and j <= rows) or (i == rows // 2 and j > rows // 2) or \ (i > rows // 2 and j == (2 * rows) - 3) or (i == rows - 1 and j < (2 * rows) - 2): print("*", end="") else: print(end=" ") print()
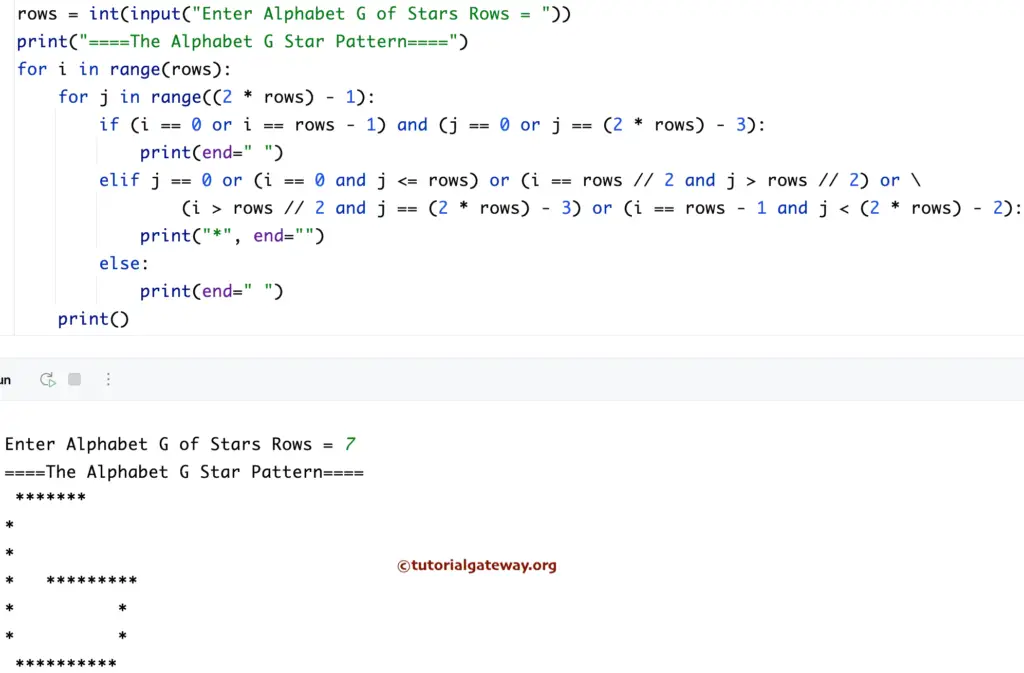
Python program to print the Alphabet G Star pattern using while loop
Instead of a for loop, this program uses the while loop to iterate the alphabetic G pattern rows and prints the stars at each position. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet G of Stars Rows = ")) n = rows // 2 m = 2 * rows i = 0 while i < rows: j = 0 while j < m - 1: if (i == 0 or i == rows - 1) and (j == 0 or j == m - 3): print(end=" ") elif j == 0 or (i == 0 and j <= rows) or (i == n and j > n) or \ (i > n and j == m - 3) or (i == rows - 1 and j < m - 2): print("*", end="") else: print(end=" ") j = j + 1 print() i = i + 1
Enter Alphabet G of Stars Rows = 13
*************
*
*
*
*
*
* ******************
* *
* *
* *
* *
* *
**********************
In this Python example, we created a GPattern function that accepts the rows and the symbol or character to print the Alphabet G pattern of the given symbol.
def GPattern(rows, ch): m = 2 * rows n = rows // 2 for i in range(rows): for j in range(2 * rows): if (i == 0 or i == rows - 1) and (j == 0 or j == m - 2): print(end=" ") elif j == 0 or (i == 0 and j <= rows) or (i == n and j > n) or \ (i > n and j == m - 1) or (i == rows - 1 and j < m): print('%c' %ch, end='') else: print(end=" ") print() row = int(input("Enter Alphabet G of Stars Rows = ")) sy = input("Symbol for G Star Pattern = ") GPattern(row, sy)
Enter Alphabet G of Stars Rows = 11
Symbol for G Star Pattern = $
$$$$$$$$$$$
$
$
$
$
$ $$$$$$$$$$$$$$$$
$ $
$ $
$ $
$ $
$$$$$$$$$$$$$$$$$$$ $