This article shows how to write a Python program to print the Alphabet R star pattern using the for loop, while loop, and functions with an example.
The below alphabet R star pattern example accepts the user-entered rows, and the nested for loop iterates the rows. The elif condition is to print stars at a few required positions to get the Alphabetical R pattern of stars and skip others.
rows = int(input("Enter Alphabet R of Stars Rows = ")) print("====The Alphabet R Star Pattern====") for i in range(rows): for j in range(rows): if i == 0 and 0 < j < rows - 1: print("*", end="") elif i != 0 and j == 0: print("*", end="") elif i == rows // 2 and j != rows - 1: print("*", end="") elif i != 0 and j == rows - 1 and i < rows // 2: print("*", end="") elif i == j and i >= rows // 2: print("*", end="") else: print(end=" ") print()
Enter Alphabet R of Stars Rows = 9
====The Alphabet R Star Pattern====
*******
* *
* *
* *
********
* *
* *
* *
* *
The Python code below reduced the number of else if conditions to print the Alphabet R pattern of stars. We have added extra spaces to make it (R) look better.
rows = int(input("Enter Alphabet R of Stars Rows = ")) print("====The Alphabet R Star Pattern====") for i in range(rows): for j in range(rows): if (i == 0 or i == rows // 2) and 0 < j < rows - 1: print("*", end=" ") elif i != 0 and (j == 0 or (j == rows - 1 and i < rows // 2)): print("*", end=" ") elif i == j and i >= rows // 2: print("*", end=" ") else: print(" ", end=" ") print()
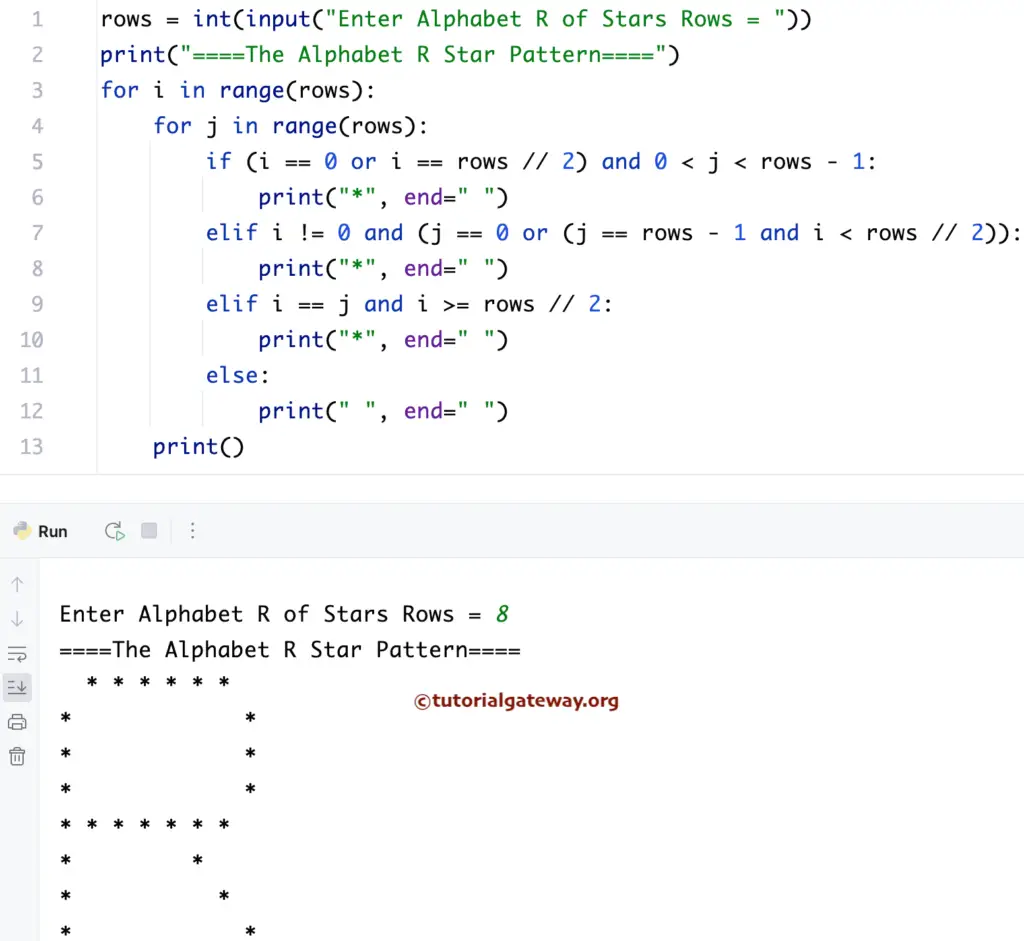
Python program to print the Alphabet R Star pattern using while loop
Instead of a For loop, this program uses the while loop to iterate the Alphabet R pattern rows and prints the stars at the required locations. For more Star Pattern programs >> Click Here.
rows = int(input("Enter Alphabet R of Stars Rows = ")) i = 0 while i < rows: j = 0 while j < rows: if (i == 0 or i == rows // 2) and 0 < j < rows - 1: print("*", end=" ") elif i != 0 and (j == 0 or (j == rows - 1 and i < rows // 2)): print("*", end=" ") elif i == j and i >= rows // 2: print("*", end=" ") else: print(" ", end=" ") j += 1 print() i += 1
Enter Alphabet R of Stars Rows = 12
* * * * * * * * * *
* *
* *
* *
* *
* *
* * * * * * * * * * *
* *
* *
* *
* *
* *
In this Python example, we created an RPattern function that accepts the rows and the symbol or character to print the Alphabet R pattern of the given symbol.
def RPattern(rows, ch): for i in range(rows): for j in range(rows): if (i == 0 or i == rows // 2) and 0 < j < rows - 1: print('%c' %ch, end=' ') elif i != 0 and (j == 0 or (j == rows - 1 and i < rows // 2)): print('%c' %ch, end=' ') elif i == j and i >= rows // 2: print('%c' %ch, end=' ') else: print(" ", end=" ") print() row = int(input("Enter Alphabet R of Stars Rows = ")) sy = input("Symbol for R Star Pattern = ") RPattern(row, sy)
Enter Alphabet R of Stars Rows = 15
Symbol for R Star Pattern = @
@ @ @ @ @ @ @ @ @ @ @ @ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @ @ @ @ @ @ @ @ @ @ @ @ @
@ @
@ @
@ @
@ @
@ @
@ @
@ @