Write a Python Program to reverse List Items or reverse list elements with a practical example. In this programming language, there is a built-in list method called reverse(), which we can use to get the result.
The syntax of the list reverse method in Python programming is as follows:
list_name.reverse()
Python list reverse() method
This function reverses the given list of items. The below code reverses the elements in an integer list.
rList = [10, 190, 120, 180, 120, 105] rList.reverse() print(rList)
[105, 120, 180, 120, 190, 10]
In this example, we declared a string list. Next, we used the reverse function on it.
TIP: Please refer to the List and methods articles to understand everything about them in Python.
FruitRevList = ['Orange', 'Banana', 'Kiwi', 'Watermelon', 'Grape', 'Blackberry'] FruitRevList.reverse() print(FruitRevList)
['Blackberry', 'Grape', 'Watermelon', 'Kiwi', 'Banana', 'Orange']
List reverse() Function Example
This example method reverses the list of items.
Fruits = ['Apple', 'Orange', 'Banana', 'Kiwi', 'Grape', 'Blackberry'] numbers = [9, 4, -5, 0, 22, -1, 2, 14] print("Original : ", Fruits) print("Original number : ", numbers) Fruits.reverse() print("\nReverse : ", Fruits) numbers.reverse() print("New Reverse Number : ", numbers)
Original : ['Apple', 'Orange', 'Banana', 'Kiwi', 'Grape', 'Blackberry']
Original number : [9, 4, -5, 0, 22, -1, 2, 14]
Reverse : ['Blackberry', 'Grape', 'Kiwi', 'Banana', 'Orange', 'Apple']
New Reverse Number : [14, 2, -1, 22, 0, -5, 4, 9]
Let me use this method on Mixed Items.
MixedRevList = ['apple', 1, 5, 'Kiwi', 'Mango'] MixedRevList.reverse() print(MixedRevList)
['Mango', 'Kiwi', 5, 1, 'apple']
This time, we used it on the Nested list.
MixedRevList = [[171, 222], [32, 13], [14, 15], [99, 76]] MixedRevList.reverse() print(MixedRevList)
[[99, 76], [14, 15], [32, 13], [171, 222]]
How to reverse user entered integer list items?
This program is the same as the first example. However, we are allowing the user to enter the length of it. Next, we used For Loop to add numbers to it.
intRevList = [] number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, number + 1): value = int(input("Please enter the Value of %d Element : " %i)) intRevList.append(value) print("Before is : ", intRevList) intRevList.reverse() print("After is : ", intRevList)
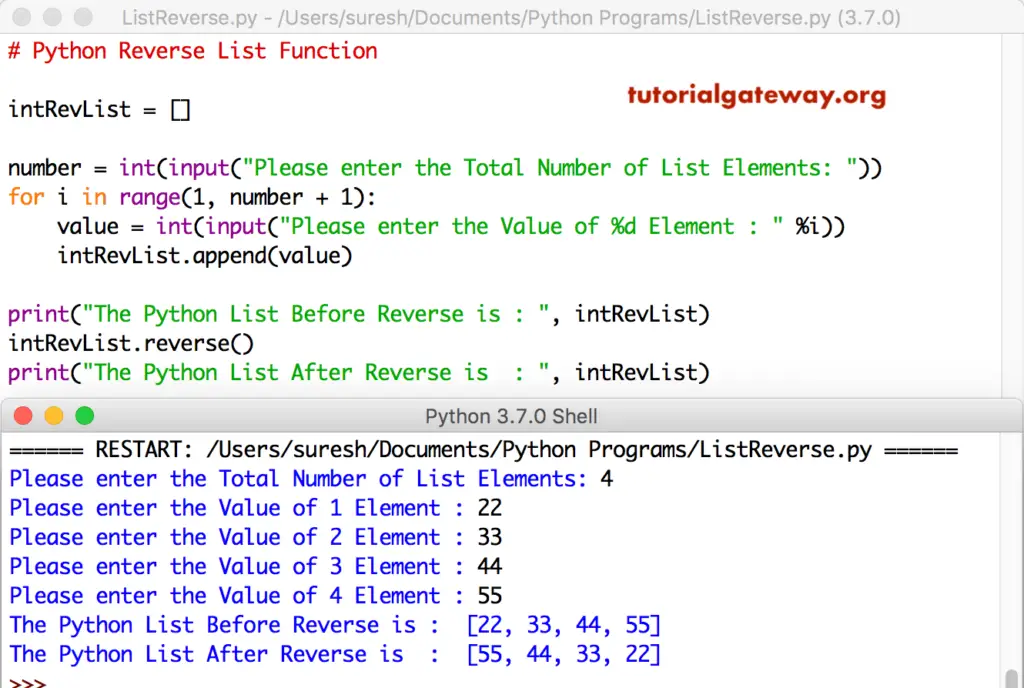
This program allows users to enter their own string or words and then Reverse them.
strRevList = [] number = int(input("Please enter the Total Number of Elements: ")) for i in range(1, number + 1): item = input("Please enter the Value of %d Element : " %i) strRevList.append(item) print("Before. : ", strRevList) strRevList.reverse() print("After : ", strRevList)
Please enter the Total Number of Elements: 4
Please enter the Value of 1 Element : Apple
Please enter the Value of 2 Element : Kiwi
Please enter the Value of 3 Element : Orange
Please enter the Value of 4 Element : Banana
Before : ['Apple', 'Kiwi', 'Orange', 'Banana']
After : ['Banana', 'Orange', 'Kiwi', 'Apple']
It allows the user to enter the length of a List. Next, we used For Loop and the append function to add numbers to the list. And then, we used the built-in list reverse() method to invert the list items.
NumList = [] Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) NumList.reverse() print("\nThe Result of a Reverse List = ", NumList)
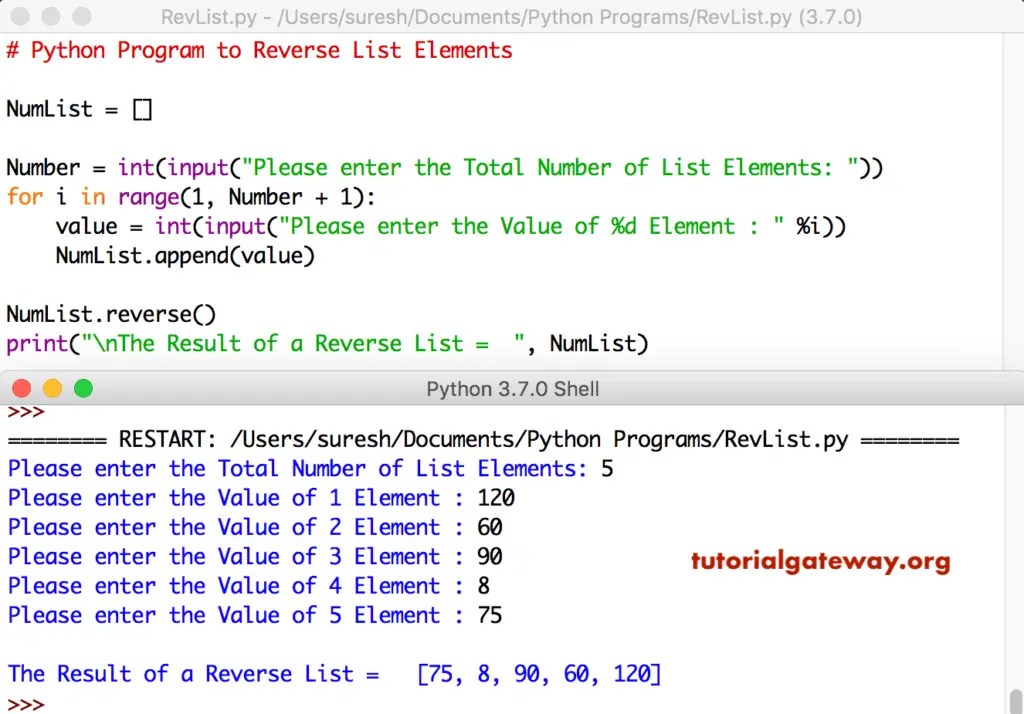
TIP: Function is used to invert the elements in a List.
Using reversed function
By default, the reversed() function returns the iterator object, so we have to convert it back to a list.
nums = [11, 25, 90, 70, 50, 60] rev = list(reversed(nums)) print(rev)
[60, 50, 70, 90, 25, 11]
Python Program to Reverse List using map and lambda functions
numLst = [10, 20, 30, 40, 50, 60] revLst = list(map(lambda x: numLst[-x], range(1, len(numLst) + 1))) print(revLst)
[60, 50, 40, 30, 20, 10]
Using slicing technique
It is one of the best approaches to invert the list elements.
nums = [10, 20, 30, 40, 50, 60] rev = nums[::-1] print(rev)
[60, 50, 40, 30, 20, 10]
Python Program to Reverse List items using for loop
We can call it the extension of the above slicing technique. This example code uses for loop and range function to iterate list elements from last to first and append them to the new list.
nums = [10, 20, 90, 40, 50, 60] rev = [] for i in range(len(nums) - 1, -1, -1): rev.append(nums[i]) print(rev)
[60, 50, 40, 90, 20, 10]
We can also use the for loop, and the list insert() function to reverse the elements.
nums = [10, 20, 30, 40, 50, 60] rev = [] for i in nums: rev.insert(0, i) print(rev)
[60, 50, 40, 30, 20, 10]
Python Program to Reverse List Items using while loop
In this Python program, we are using a While loop. Inside the while loop, we performed the Swapping with the help of the third variable. I suggest you refer Swap two Numbers article to understand the Python logic.
NumList = [] Number = int(input("Please enter the Total Numbers : ")) for i in range(1, Number + 1): value = int(input("%d Element : " %i)) NumList.append(value) j = Number - 1 i = 0 while(i < j): temp = NumList[i] NumList[i] = NumList[j] NumList[j] = temp i = i + 1 j = j - 1 print("\nThe Result = ", NumList)
Please enter the Total Numbers : 3
1 Element : 1
2 Element : 2
3 Element : 3
The Result = [3, 2, 1]
This example also uses the while loop, but we used the list pop() method to reverse the items this time. The pop() function returns the last value, and the append() method will add that number to a new list.
nums = [10, 20, 30, 40, 50, 60] rev = [] while nums: rev.append(nums.pop()) print(rev)
[60, 50, 40, 30, 20, 10]
Python Program to Reverse List elements using Functions
This List items program is the same as the above. However, we separated the logic using Functions.
def reverseList(NumList, num): j = Number - 1 i = 0 while(i < j): temp = NumList[i] NumList[i] = NumList[j] NumList[j] = temp i = i + 1 j = j - 1 NumList = [] Number = int(input("Please enter the Total Number of Elements: ")) for i in range(1, Number + 1): value = int(input("%d Element : " %i)) NumList.append(value) reverseList(NumList, Number) print("\nThe Result = ", NumList)
Please enter the Total Number of Elements: 5
1 Element : 10
2 Element : 20
3 Element : 30
4 Element : 40
5 Element : 50
The Result = [50, 40, 30, 20, 10]
Python Program to Reverse List elements using Recursion
This program reverses the List items by calling functions recursively.
def reverseList(NumList, i, j): if(i < j): temp = NumList[i] NumList[i] = NumList[j] NumList[j] = temp reverse_list(NumList, i + 1, j-1) NumList = [] Number = int(input("Please enter the Total Number of Elements: ")) for i in range(1, Number + 1): value = int(input("%d Element : " %i)) NumList.append(value) reverseList(NumList, 0, Number - 1) print("\nThe Result = ", NumList)
Please enter the Total Number of Elements: 6
1 Element : 12
2 Element : 13
3 Element : 14
4 Element : 15
5 Element : 16
6 Element : 27
The Result = [27, 16, 15, 14, 13, 12]
A better approach to reverse the list items using recursion is shown below.
def reverseList(n): if len(n) == 0: return [] else: return [n[-1]] + reverseList(n[:-1]) nums = [25, 77, 90, 80, 150, 60, 180] rev = reverseList(nums) print(rev)
[180, 60, 150, 80, 90, 77, 25]