Write a Program to Swap Two Numbers in Python using the Temp variable, Bitwise Operators, and Arithmetic Operators. The standard technique is using temporary variables. However, Python allows you to assign values to multiple variables using a comma, the best alternative method.
Python Program to Swap Two Numbers Using Temp
This program helps the user to enter two numeric values. Next, swap those two values using temp variables.
a = float(input(" Please Enter the First Value a: ")) b = float(input(" Please Enter the Second Value b: ")) print("Before Swapping two Number: a = {0} and b = {1}".format(a, b)) temp = a a = b b = temp print("After Swapping two Number: a = {0} and b = {1}".format(a, b))
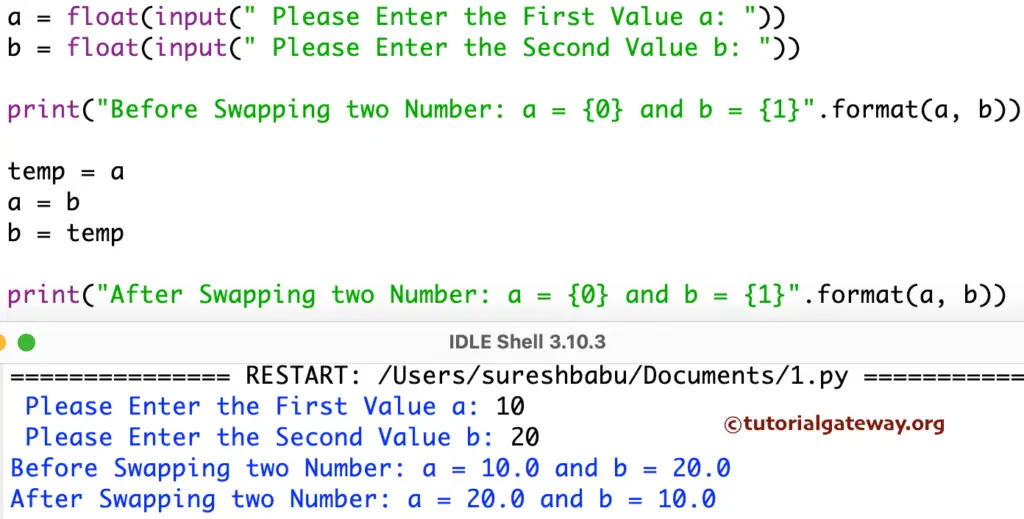
In the above Python program example, we assigned a = 10 and b = 20
Temp = a – assigning a value to the Temp variable.
Temp = 10
a = b – assign b value to variable a
a = 20
b = Temp – Temp value to variable b
b = 10
Swap Two Numbers using Functions
This program for swap numbers is the same as above, but this time, we separated the logic using Functions.
# using functions def swap_numbers(a, b): temp = a a = b b = temp print("After: num1 = {0} and num2 = {1}".format(a, b)) num1 = float(input(" Please Enter the First Value : ")) num2 = float(input(" Please Enter the Second Value : ")) print("Before: num1 = {0} and num2 = {1}".format(num1, num2)) swap_numbers(num1, num2)
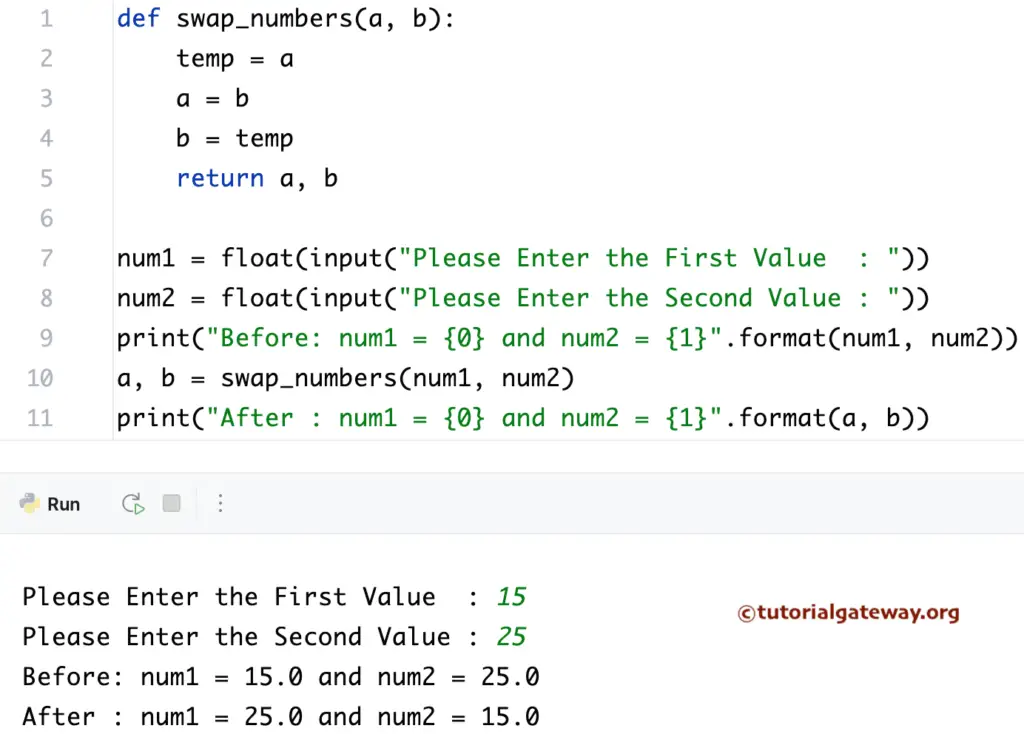
Python Program to swap two numbers without temp variable
This program accepts two floating-point numbers. Next, we performed multiple assignments to swap them.
a = float(input("Enter the First Value = ")) b = float(input("Enter the Second Value = ")) a, b = b, a print("a = {0} and b = {1}".format(a, b))
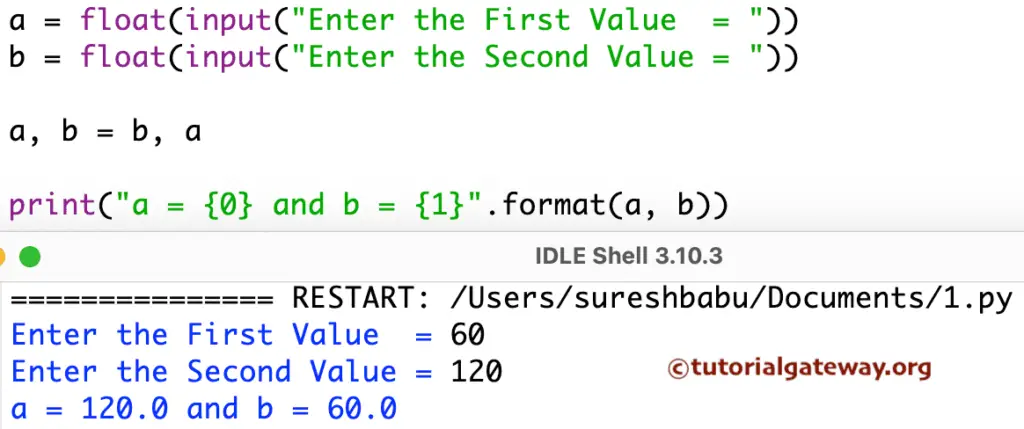
Enter the First Value = 10
Enter the Second Value = 20
a = 20.0 and b = 10.0
Python Program to Swap Two Floating-Point Numbers using Arithmetic Operators
In this example, instead of using the temp or third variable to swap two numbers, we will use Arithmetic Operators. It. is purely an addition and subtraction of two variables.
# using arithmetic + and - operators a = float(input(" Please Enter the First Value a: ")) b = float(input(" Please Enter the Second Value b: ")) print("Before Swapping two Number: a = {0} and b = {1}".format(a, b)) a = a + b b = a - b a = a - b print("After Swapping two Number: a = {0} and b = {1}".format(a, b))
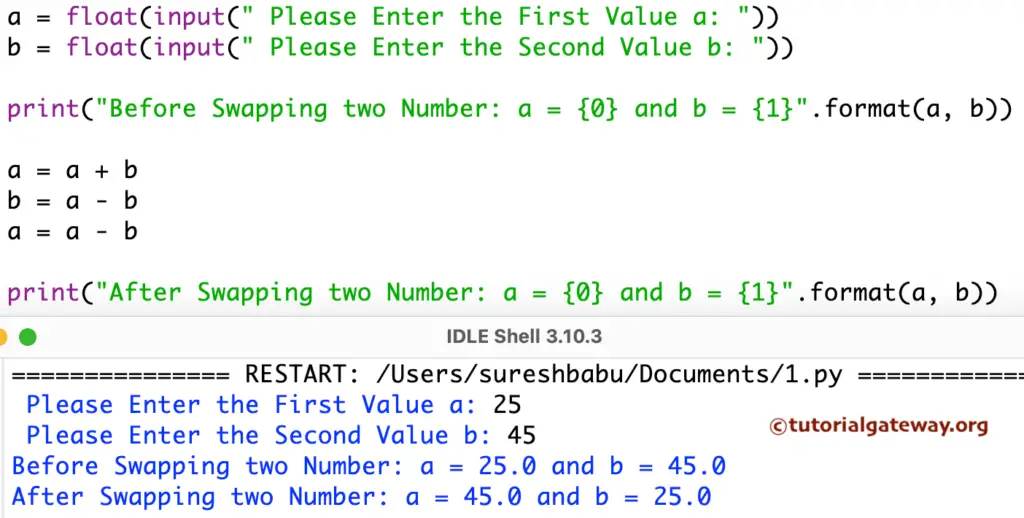
User Entered Values are a = 25 and b = 45
a = a + b = 25 + 45 = 70
b = a – b = 70 – 45 = 25
a = a – b = 70 – 25 = 45
Swap Two Numbers using XOR Bitwise Operator
Here, we are using XOR Bitwise operators to swap two numbers.
a = int(input(" Please Enter the First Value : ")) b = int(input(" Please Enter the Second Value : ")) print("Before: a = {0} and b = {1}".format(a, b)) a = a^b b = a^b a = a^b print("After: a = {0} and b = {1}".format(a, b))
Using Bitwise Operators output
Please Enter the First Value : 111
Please Enter the Second Value : 222
Before: a = 111 and b = 222
After: a = 222 and b = 111