How to use this len built-in function to find the Python list length with a few practical examples? The len function is used to find and returns the length of any collection such as a dictionary, tuple, etc.
The syntax of this len function to find the length of a list in Python or to get the size is:
len(name)
Python List length Example
We can use this Python len function to count the number of elements, length, or size of a list. In this example, we declared an empty one. And we used the len function to calculate for an empty one. Next, we find the size of an integer, and it returns the total number of elements.
emptyLi = [] print(len(emptyLi)) integerLi = [12, 22, 33, 44, 55, 66, 77] print("integerLi) print(len(integerLi))
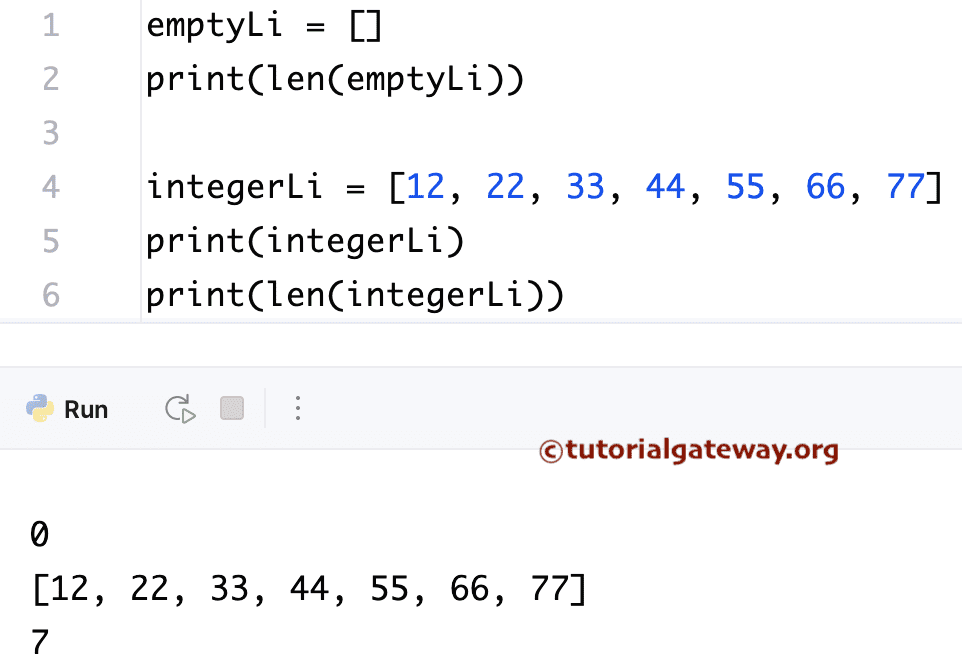
How to use Python len function to find String List Length?
When you use this len function on String items, it returns the total number of words in a string. Or, say, it finds the total items or words in the string. This example shows the total number of string items or the total number of words in the string List.
stringLi = ['Krishna', 'John', 'Yung', 'Ram', 'Steve'] print(stringLi) print( len(stringLi))
['Krishna', 'John', 'Yung', 'Ram', 'Steve']
5
How do Find the Length of a Mixed List using Python len function?
Apart from regular ones, you can also get the length of the mixed list. In the fifth line of code, we declared a Tuple inside it.
Next, we are going to find the size (this includes tuples). Remember, Python len counts the complete Tuple as a single element.
mixedLi = ['Krishna', 20, 'John', 40.5, 'Yung', 11.98, 'Ram', 22] print(len(mixedLi)) print() mixedLi2 = ['Krishna', 20, 'John', (40, 50, 65), 'Yung', 11.98, 'Ram'] print(len(mixedLi2))
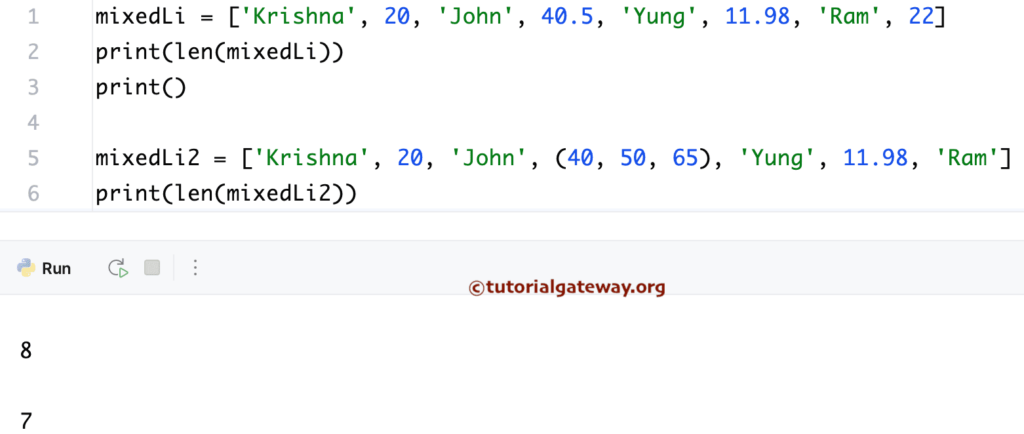
Python len function to find the length of a Nested List
Let us see how to find the length of a nested list input in this programming. For this, we declared a nested one with the combination of a few other items. If you want the complete length, then it considers the Nested object as one element.
However, you can get the Nested size using the index value. For example, the below code is finding the size of a nested one [20, 40, 50, 65, 22].
nestedLi = ['Krishna', 20, 'John', [20, 40, 50, 65, 22], 'Yung', 11.98] print(nestedLi) print(len(nestedLi[3]))
['Krishna', 20, 'John', [20, 40, 50, 65, 22], 'Yung', 11.98]
5
How to Find the Length of a Dynamic List Items?
This program allows the user to enter the total number of elements. Next, using For Loop iterates each position and allows you to enter the individual elements. Within the loop, we used the append function to add items. Once we got the items, we found the size using this len function.
intLi = [] Number = int(input("Please enter the Total Number of Items : ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) intLi.append(value) print("\n Original = ", intLi) print("Size of a Dynamic = ", len(intLi))
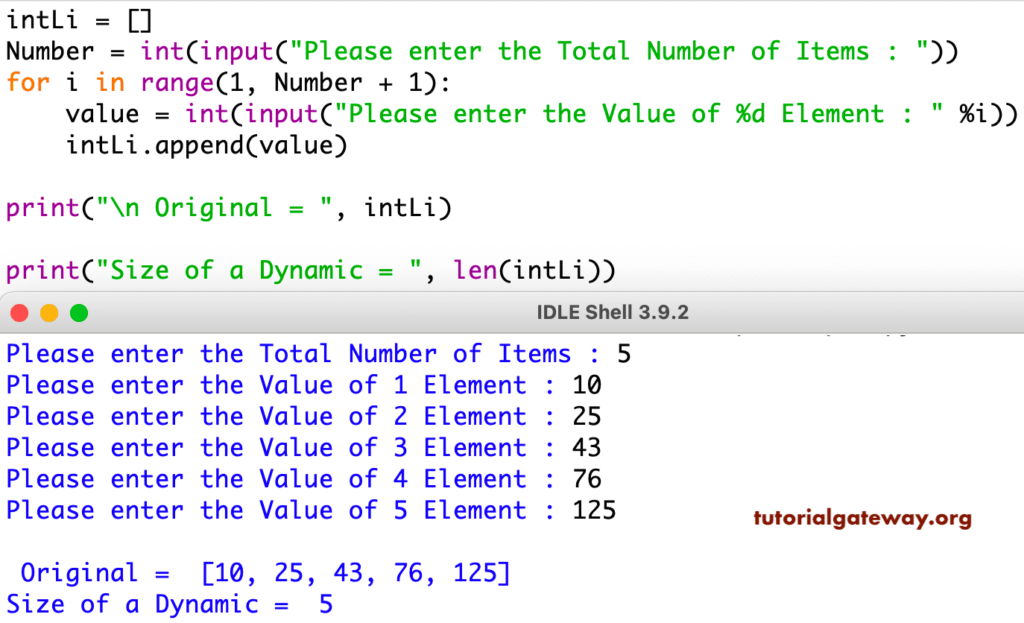