The len function finds the length of an object. Or, say, it returns the number of items in an object. If you are finding the string length, then this Python len function returns the total number of characters.
In this section, we discuss how to use this Python len function on String, Tuple, List, Dictionary, Sets, Bytes, and range. to find the length. The syntax of this function is
len(Object_Name)
Python len function to find string length
When you use this Python function on a String object, it counts and returns the total number of characters (including spaces) in a string. Or, say, finds the length of a string. In this example, we are finding the size of an empty string and Tutorial Gateway.
a = '' print(a) print(len(a)) b = 'Tutorial Gateway' print(len(b))
0
6
Python len function to find tuple length
This example finds the length of a Tuple, which means counting the total number of items in this tuple. First, we declared an integer and a string Tuple. Next, it returns the number of items in an integer and string Tuple.
tupleExample = (10, 20, 30, 40, 50, 60, 70, 80, 90) print(len(tupleExample)) tupleExample2 = ('Banana', 'Orange', 'Blackberry', 'Apple') print(len(tupleExample2))
9
4
Python len function to find list length
This function also helps you to find the total number of List items. First, we declared an empty List and found its length. Next, we used it to find the numeric items. Finally, it calculates the number of elements in a string list.
listLe = () print(len(listLe)) listLeExample = [15, 20, 35, 40, 55, 60, 70] print(len(listLeExample)) stringListLe = ['apple', 'mango', 'orange', 'cherry','banana'] print(len(stringListLe))
0
7
5
How to find dictionary length using Python len function?
We can use this function on collections such as dictionaries, sets, or frozen sets. The len function on Dictionary returns the length. Or calculates the total number of available items in a given Dictionary.
DictionaryLe = dict() print(len(DictionaryLe)) print() DictionaryLeExample = {1: 'mango', 2: 'kiwi', 3: 'cherry'} print(len(DictionaryLeExample)) print() mixedDictionaryLe = {'ID': 42, 'name': 'Kevin', 'age': 25, 1: 1200} print(len(mixedDictionaryLe))
0
3
4
Python len function to find set length
The Python len function also finds the total number of items or lengths in a set. Next, we declared a numeric set and a string set. Using this one, we are returning the elements of a numeric set and a string set.
SetExample = {125, 200, 350, 400, 505, 607, 760} print(len(SetExample)) print() stringSet = {'apple', 'mango', 'cherry', 'kiwi', 'orange', 'banana'} print(len(stringSet))
7
6
Python len function to find the length of an array
In this example, we find the size of an array. First, we calculate the length of an empty array. Next, find the total items in an Integer and String Array.
Arr = [] print(len(Arr)) ArrExample = [5, 12, 35, 22, 40, 5] print("\n", ArrExample) print(len(ArrExample)) stringArr = ['apple', 'cherry', 'kiwi', 'mango', 'orange', 'banana'] print("\n", stringArr) print(len(stringArr))
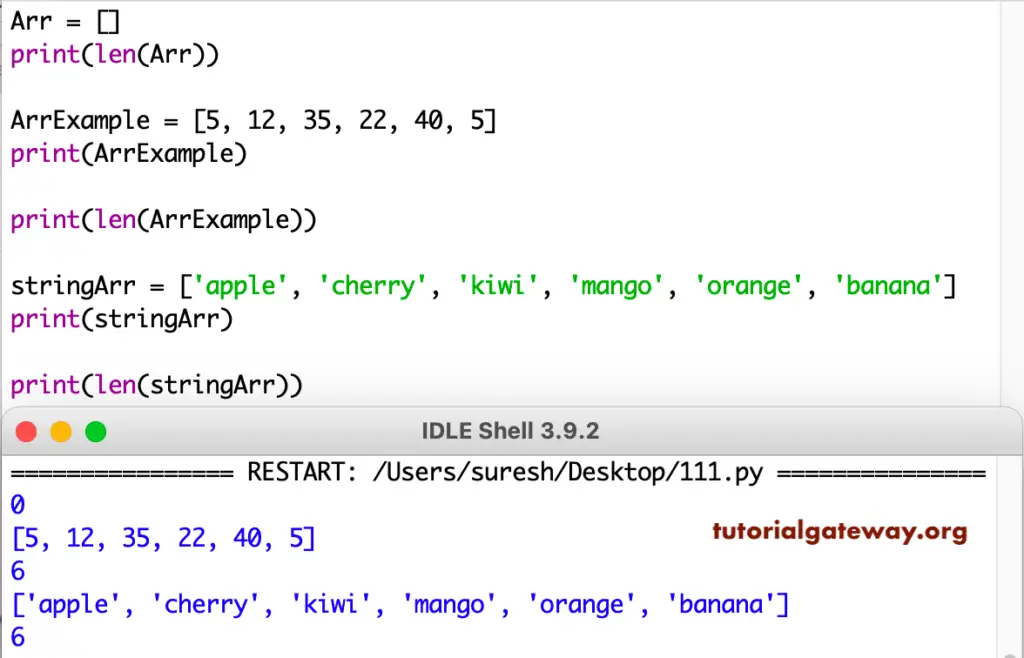
The len function is not only about returning object size, but you can also find the range length. In this example, we declared a range from 4 to 10 and returns the total elements between that range. To understand better, we used another example with a big range.
rangeLe = range(4, 10) print(len(rangeLe)) rangeLe = range(15, 109) print(len(rangeLe))
6
94
Python len function to find the byte length
This function also allows you to find the Byte length, and this example returns the same.
by = b'Simple Example' print("Bytes = ", len(by))
Bytes = 14