The python math floor function is one of the Mathematical Functions available in the math library.
This python math floor function is used to return the closest integer value which is less than or equal to the specified expression or Value.
Syntax
In this article, we will show you, How to write the math floor in Python programming with examples, and the syntax of it in the math library is:
math.FLOOR (Expression)
Floor Function Example
The floor Function in Python returns the closest integer value which is less than or equal to the given numeric value. Let me show you a simple example of a floor function that returns the closest value of 12.95.
import math val = math.floor(12.95) print(val)
12
In this floor example, it accepts the argument and returns the smallest integer value of both positive and negative values.
import math print('The final result of math.floor(10.45) = ', math.floor(10.45)) print('The final result of math.floor(20.99) = ', math.floor(20.99)) print() print('The final result of math.floor(-120.49) = ', math.floor(-120.49)) print('The final result of math.floor(-160.99) = ', math.floor(-160.99))
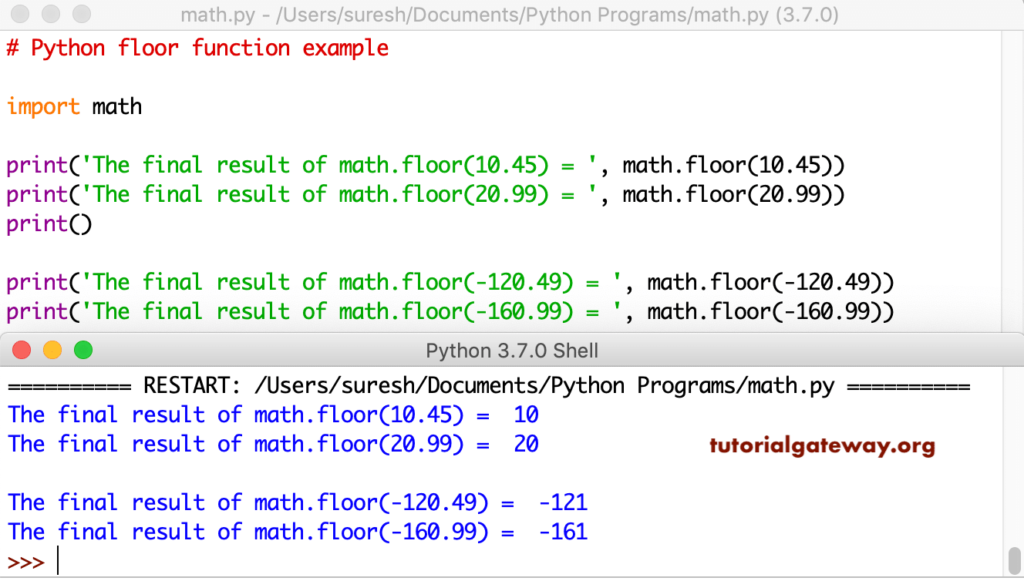
Python math floor Function Example
The following query will show you the multiple ways to use this math floor function.
import math # This allows us to use the floor function a = 0.24 b = -90.98 c = 45.05 d = 45.98 e = math.floor(2.45 + 7.55 - 14.88) pi = math.floor(math.pi) x = [-22.45, 2.40, 9.65] # List Example y = (-2.45, 22.10, 22.95) # Tuple Example print(" The Value of 'a' after the floor function is: ", math.floor(a)) print(" The Value of %.2f after the floor function is: %d" %(b, math.floor(b))) print(" The Value of %.2f after the floor function is: %d" %(c, math.floor(c))) print(" The Value of %.2f after the floor function is: %d" %(d, math.floor(d))) print(" The Value of 'e' after the floor function is: ", e) print(" The Value of 'PI' after the floor function is: ", pi) print(" ") # printing List values print(" First Value from List is: ", math.floor(x[0])) print(" Second Value from List is: ", math.floor(x[1])) print(" Third Value from List is: ", math.floor(x[2])) print(" ") # printing Tuple values print(" First Value from List is: ", math.floor(y[0])) print(" Second Value from List is: ", math.floor(y[1])) print(" Third Value from List is: ", math.floor(y[2]))
The Value of 'a' after the floor function is: 0
The Value of -90.98 after the floor function is: -91
The Value of 45.05 after the floor function is: 45
The Value of 45.98 after the floor function is: 45
The Value of 'e' after the floor function is: -5
The Value of 'PI' after the floor function is: 3
First Value from List is: -23
Second Value from List is: 2
Third Value from List is: 9
First Value from List is: -3
Second Value from List is: 22
Third Value from List is: 22
First, We imported the math library using the following statement. This will allow us to use mathematical functions like floor.
import math
Below lines of Python code are used to declare variables and assign the value.
a = 0.24 b = -90.98 c = 45.05 d = 45.98
In the next line, we applied the math floor function in python directly on the multiple values (some calculations)
e = math.floor(2.45 + 7.55 - 14.88)
It means
floor (2.45 + 7.55 – 14.88)
floor (-4.88) = – 5
In the next line, we declared the List and Tuple. Next, we used the floor function inside the print statements to find the closest integer value of the above-mentioned variable.
Difference between int and floor
This is a frequently asked question because both the int and floor functions return the same result for positive values. However, if you check with negative values as an argument, then you can see the difference.
import math print('math.floor(100.45) Result = ', math.floor(100.45)) print('math.floor(200.99) Result = ', math.floor(200.99)) print('int(100.45) Result = ', int(100.45)) print('int(200.99) Result = ', int(200.99)) print() print('math.floor(-150.49) result = ', math.floor(-150.49)) print('math.floor(-260.99) result = ', math.floor(-260.99)) print('int(-150.49) result = ', int(-150.49)) print('int(-260.99) result = ', int(-260.99))
math.floor(100.45) Result = 100
math.floor(200.99) Result = 200
int(100.45) Result = 100
int(200.99) Result = 200
math.floor(-150.49) result = -151
math.floor(-260.99) result = -261
int(-150.49) result = -150
int(-260.99) result = -260
floor Division Example
This Mathematical operator returns the floored result of the division.
# floor Division example a = 10 b = 3 x = a / b print(x) y = a // b print(y)
3.3333333333333335
3
List Example
Let me use this math floor function on List items. Here, we are using the For Loop to iterate the list item and then apply the floor function for each item.
import math numbers = [-10.89, 20.22, 40.67, -350.11, -450.91] for num in numbers: print(math.floor(num))
-11
20
40
-351
-451
This Python math.floor function example is the same as above. However, this time we are using the Map and Lambda functions to iterate items.
import math numbers = [-10.89, 20.22, 40.67, -350.11, -450.91] print(numbers) print() floor_result = map(lambda num: math.floor(num), numbers) print('The final result = ', list(floor_result))
floor function along with map and lambda functions output
[-10.89, 20.22, 40.67, -350.11, -450.91]
The final result = [-11, 20, 40, -351, -451]