The Python static method or @staticmethod can call without creating an instance or object. Although Python staticmethod belongs to a class, you can call it directly by the static method name. If you want a function that doesn’t need any class variables or instance variables to operate, then you can create it as a static method.
For example, if you want to print a welcome message, then you can create it as a staticmethod because it won’t change. Or it won’t need any class variable to display that message. We can create a static method in Python by using @staticmethod decorator or staticmethod() function. Although we don’t recommend the second option, we are showing the possibilities for your understanding.
In this section, we explain how to create a static method in Python programming language and call the staticmethod. In most of the examples, we combine static methods with non-static ones. At first, it might confuse you, but this allows you to differentiate between static and non-static.
Python static method Example
You can write both static and non-static methods with a single class. Let me create a Pythonnonstatic func_message() and one static method func_msg() using @staticmethod decorator.
TIP: Refer to the Functions and class article to create a Python class for staticmethod.
class Employee: def func_message(self): print('Welcome to Programming') @staticmethod def func_msg(): print("Welcome to Tutorial Gateway")
To display something from a class, you need to create an object or instance from that class.
Calling @staticmethod in Python
This Python example shows how to call a staticmethod or @staticmethod and a non-static method. As we said earlier, we don’t need to create an instance to call the staticmethod.
If you look into the example, Employee. func_msg() means classname. staicmethod_name. It automatically calls the func_msg function in the employee class.
Next, we created an instance of the Employee class to call another function, which is a non-static function.
class Employee: def func_message(self): print('Welcome to Programming') @staticmethod def func_msg(): print("Welcome to Tutorial Gateway") Employee.func_msg() emp = Employee() emp.func_message()
Welcome to Tutorial Gateway
Welcome to Programming
Python static method approach 2
In this example, we create a Python static method using staticmethod() function. Within the class, first, we created two regular functions. Next, we used the below statement to convert the regular function to a staticmethod.
Employee.func_addition = staticmethod (Employee. func_addition). It means class_name. method_name = staticmethod (class_name. method_name)
class Employee: def func_message(self): print('Welcome to The World') def func_addition(a, b): return a + b Employee.func_addition = staticmethod(Employee.func_addition) print('Total = ', Employee.func_addition(25, 50)) emp = Employee() emp.func_message()
Total = 75
Welcome to the World
It is another example of creating a static method using the staticmethod() function. Here, we created two func_addition() and func_multiply()
class Employee: def func_message(self): print('Welcome to this World') def func_addition(a, b): return a + b def func_multiply(a, b): return a * b emp = Employee() emp.func_message() Employee.func_addition = staticmethod(Employee.func_addition) print('Total = ', Employee.func_addition(25, 50)) Employee.func_multiply = staticmethod(Employee.func_multiply) print('Multiplication = ', Employee.func_multiply(25, 50))
Welcome to this World
Total = 75
Multiplication = 1250
Python @staticmethod with Arguments example
In Python, you don’t have to use self or cls attributes to create a static method or staticmethod. Like a function, you can create it and add the arguments as per your requirements.
In this example, we created two of them. The func_msg() function prints a welcome message. And split_string (message) function accepts a string and splits that string by comma delimiter. I suggest you refer to the String split article.
class Employee: def func_message(self): print('Welcome to Programming') @staticmethod def func_msg(): print("Welcome to Tutorial Gateway") @staticmethod def split_string(message): return message.split(",") Employee.func_msg() countries = 'India, China, Japan, USA, UK, Australia, Canada' print(Employee.split_string(countries))
Python @staticmethod definition with arguments output
Welcome to Tutorial Gateway
['India', ' China', ' Japan', ' USA', ' UK', ' Australia', ' Canada']
It is another example of a Python static method with arguments. In this example, we used functions to split the message using a comma and used the replace function to replace the comma with an underscore. I suggest you refer String replace article.
class Employee: def func_message(self): print('Welcome to Programming') @staticmethod def func_msg(): print("Welcome to Tutorial Gateway") @staticmethod def split_string(message): return message.split(",") @staticmethod def replace_string(message): return message.replace(",", "_") Employee.func_msg() countries = 'India, China, Japan, USA, UK, Australia, Canada' print(Employee.split_string(countries)) print(Employee.replace_string(countries))
@staticmethod with arguments output
Welcome to Tutorial Gateway
['India', ' China', ' Japan', ' USA', ' UK', ' Australia', ' Canada']
India_ China_ Japan_ USA_ UK_ Australia_ Canada
Arithmetic Operations
Here, we create three Python staticmethod to perform addition, subtraction, and multiplication.
class Employee: def func_message(self): print('Welcome to Programming Language') @staticmethod def func_addition(a, b): return a + b @staticmethod def func_multiply(a, b): return a * b @staticmethod def func_subtract(a, b): return a - b print(Employee.func_addition(10, 20)) print(Employee.func_addition(15, 28)) print(Employee.func_multiply(2, 3)) print(Employee.func_multiply(5, 7)) print(Employee.func_subtract(20, 75)) print(Employee.func_subtract(1600, 249))
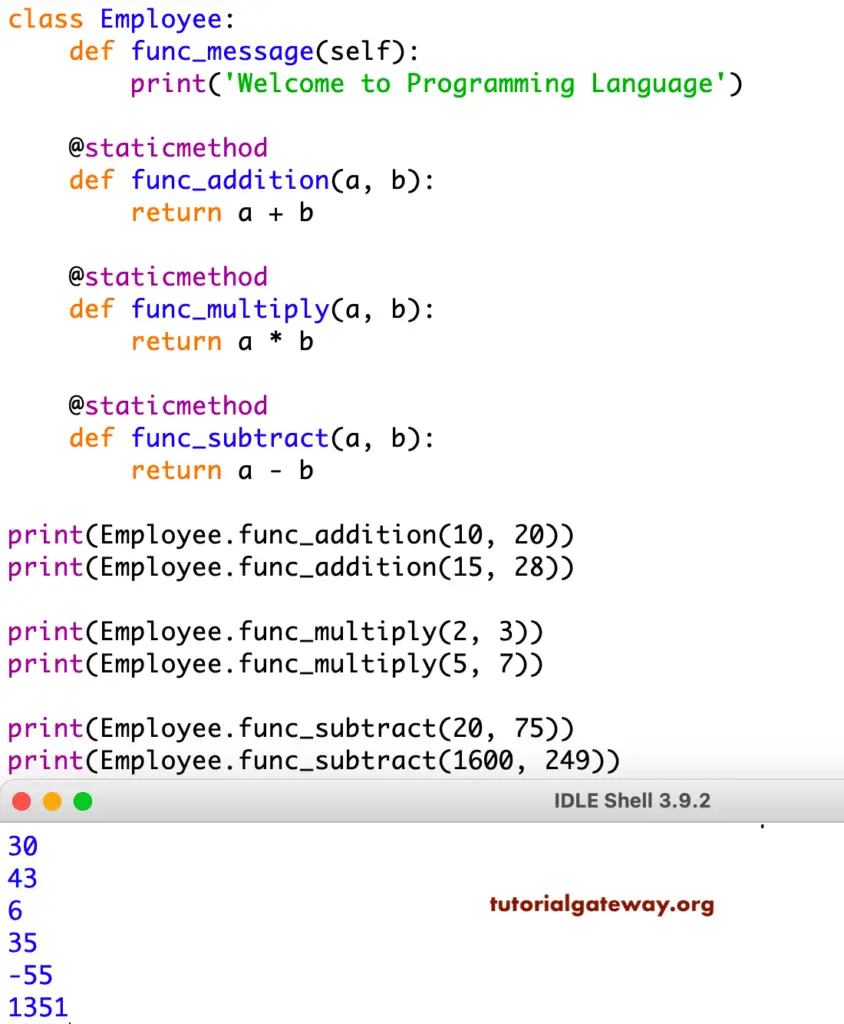