The Python union function combines all the items in the original set, and the specified one, and the syntax of it is
set.union(set1, set2,…,setN)
Python set union example
In this example, we declared two sets, and then we combined those two using the union method.
x = {'a', 'b', 'c', 'd'} y = {'e', 'f', 'g', 'h'} print('Items in x = ', x) print('Items in y = ', y) z = x.union(y) print('Items in z = ', z)
Items in x = {'c', 'd', 'b', 'a'}
Items in y = {'f', 'h', 'e', 'g'}
Items in z = {'f', 'h', 'd', 'g', 'c', 'b', 'a', 'e'}
The sets don’t allow duplicate values inside them. So, using the union method to combine the two, removes those duplicate values. If you observe the output, a and c was returned only once (though they are available in both x and y).
TIP: Please refer to the set in Programming.
x = {'a', 'b', 'c', 'd'} y = {'a', 'f', 'g', 'c', 'h', 'j', 'k'} print('x = ', x) print('y = ', y) z = x.union(y) print('z = ', z)
x = {'b', 'a', 'c', 'd'}
y = {'k', 'j', 'g', 'a', 'c', 'h', 'f'}
z = {'b', 'k', 'a', 'd', 'j', 'g', 'c', 'h', 'f'}
Python set union function Example 2
Let me use three of them with the union function. This example combines all three sets. It first combines items in x and then y and z.
x = {'a', 'b' } y = {'e', 'f', 'g', 'h'} z = {'apple', 'cherry', 'kiwi'} print('Items in x = ', x) print('Items in y = ', y) print('Items in z = ', z) new = x.union(y, z) print('Items in new = ', new)
Items in x = {'a', 'b'}
Items in y = {'h', 'g', 'e', 'f'}
Items in z = {'kiwi', 'apple', 'cherry'}
Items in new = {'kiwi', 'g', 'h', 'apple', 'e', 'a', 'f', 'b', 'cherry'}
This example shows how to combine numeric and strings.
x = {'a', 'b' } y = {10, 20, 30, 40} print('Items in x = ', x) print('Items in y = ', y) z = x.union(y) print('Items in z = ', z)
Combine numeric and string output
Items in x = {'b', 'a'}
Items in y = {40, 10, 20, 30}
Items in z = {20, 'b', 40, 10, 30, 'a'}
Here, we declare a numeric, string, and mixed set. Next, we used the method to combine them all and return a new one.
x = {'a', 'b' } y = {10, 20, 30, 40} z = {120, 'cherry', 90, 'kiwi'} print('Set Items in x = ', x) print('Set Items in y = ', y) print('Set Items in z = ', z) new_set = x.union(y, z) print('Set Items in new = ', new_set)
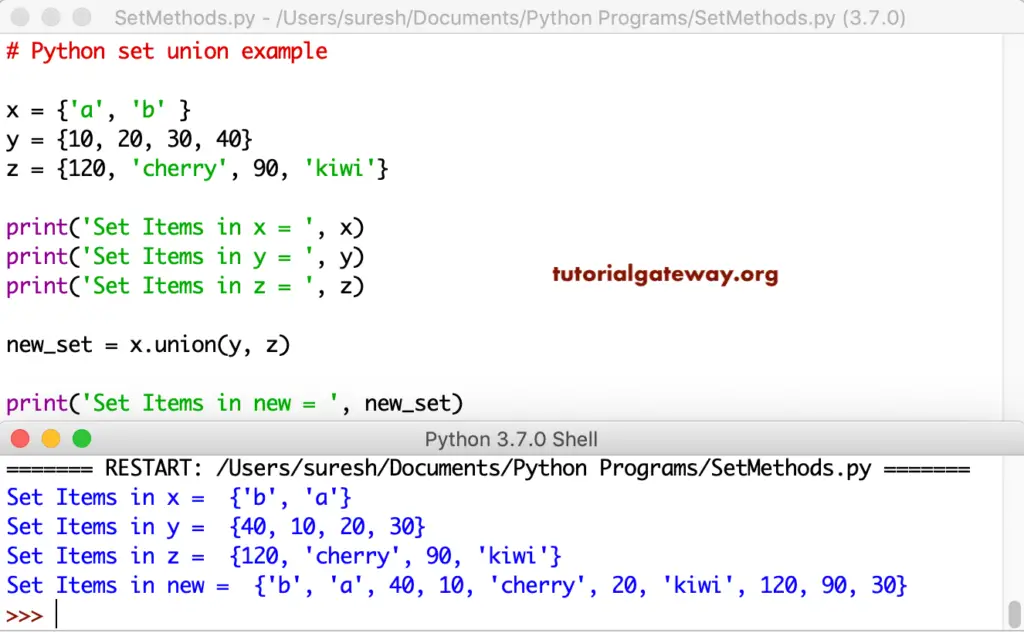