Write a Python Program to Print a Hollow Inverted Pyramid Star Pattern using a for loop, while loop, and functions. This example uses the for loop to iterate and print the Hollow Inverted Pyramid Star Pattern.
rows = int(input("Enter Hollow Inverted Pyramid Pattern Rows = ")) for i in range(rows, 0, -1): for j in range(rows - i): print(end = ' ') for k in range(i): if (i == 1 or i == rows or k == 0 or k == i - 1): print('*', end = ' ') else: print(' ', end = ' ') print()
Output.
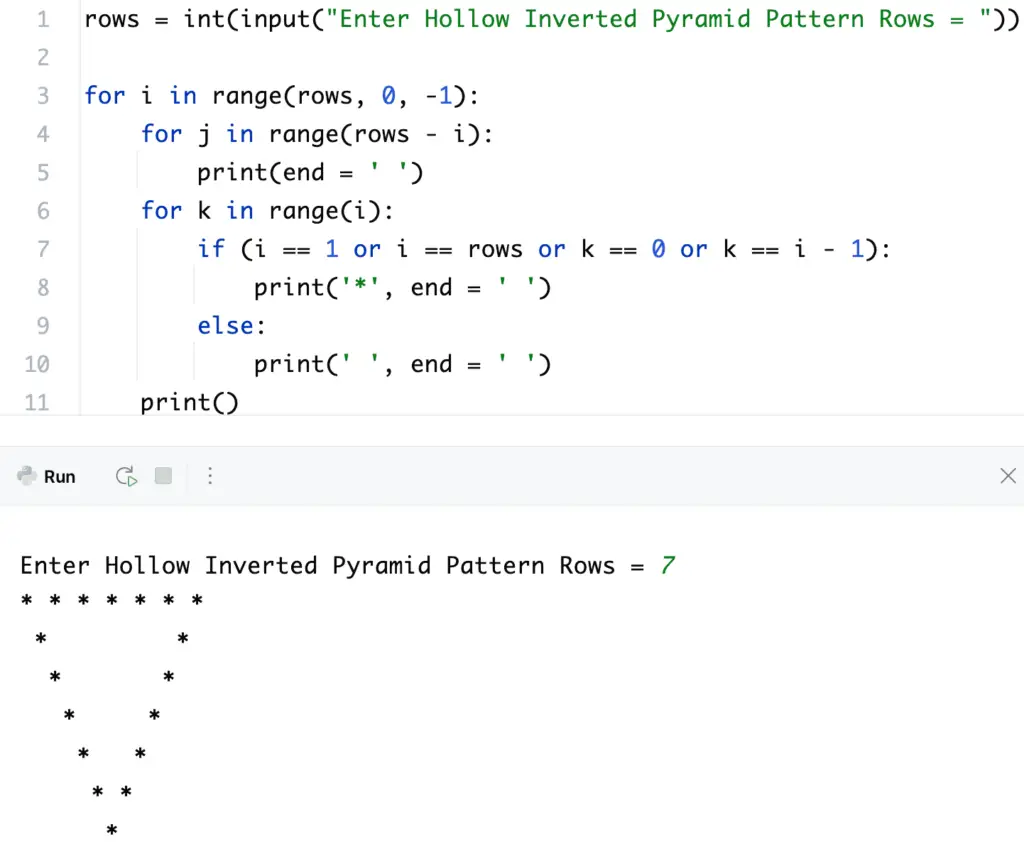
This Python program replaces the above for loop with a while loop to print the Hollow Inverted Pyramid star Pattern.
rows = int(input("Enter Hollow Inverted Pyramid Pattern Rows = ")) i = rows while i > 0: j = 0 while j < rows - i: print(end = ' ') j = j + 1 k = 0 while k < i: if (i == 1 or i == rows or k == 0 or k == i - 1): print('*', end = ' ') else: print(' ', end = ' ') k = k + 1 i = i - 1 print()
Output
Enter Hollow Inverted Pyramid Pattern Rows = 9
* * * * * * * * *
* *
* *
* *
* *
* *
* *
* *
*
In this Python example, we created a HollowInvertedPyramid function that accepts the rows and characters to Print the Hollow Inverted Pyramid Pattern. It replaces the star within the If else statement in the hollow inverted pyramid pattern with a given symbol.
def HollowPyramid(rows, ch): for i in range(rows, 0, -1): for j in range(rows - i): print(end = ' ') for k in range(i): if (i == 1 or i == rows or k == 0 or k == i - 1): print('%c' %ch, end = ' ') else: print(' ', end = ' ') print() rows = int(input("Enter Hollow Inverted Pyramid Pattern Rows = ")) ch = input("Symbol in Hollow Inverted Pyramid Pattern = ") HollowPyramid(rows, ch)
Output
Enter Hollow Inverted Pyramid Pattern Rows = 13
Symbol in Hollow Inverted Pyramid Pattern = #
# # # # # # # # # # # # #
# #
# #
# #
# #
# #
# #
# #
# #
# #
# #
# #
#