Write a Python Program to Perform Arithmetic Operations on Lists using For Loop and While Loop with a practical example.
Python Program to Perform Arithmetic Operations on Lists Example
In this python program, we are using For Loop to iterate each element in a given List. Inside the Python loop, we are performing arithmetic operations on elements of the first and second lists.
# Python Program to Perform List Arithmetic Operations NumList1 = [10, 20, 30] NumList2 = [5, 2, 3] add = [] sub = [] multi = [] div = [] mod = [] expo = [] for j in range(3): add.append( NumList1[j] + NumList2[j]) sub.append( NumList1[j] - NumList2[j]) multi.append( NumList1[j] * NumList2[j]) div.append( NumList1[j] / NumList2[j]) mod.append( NumList1[j] % NumList2[j]) expo.append( NumList1[j] ** NumList2[j]) print("\nThe List Items after Addition = ", add) print("The List Items after Subtraction = ", sub) print("The List Items after Multiplication = ", multi) print("The List Items after Division = ", div) print("The List Items after Modulus = ", mod) print("The List Items after Exponent = ", expo)
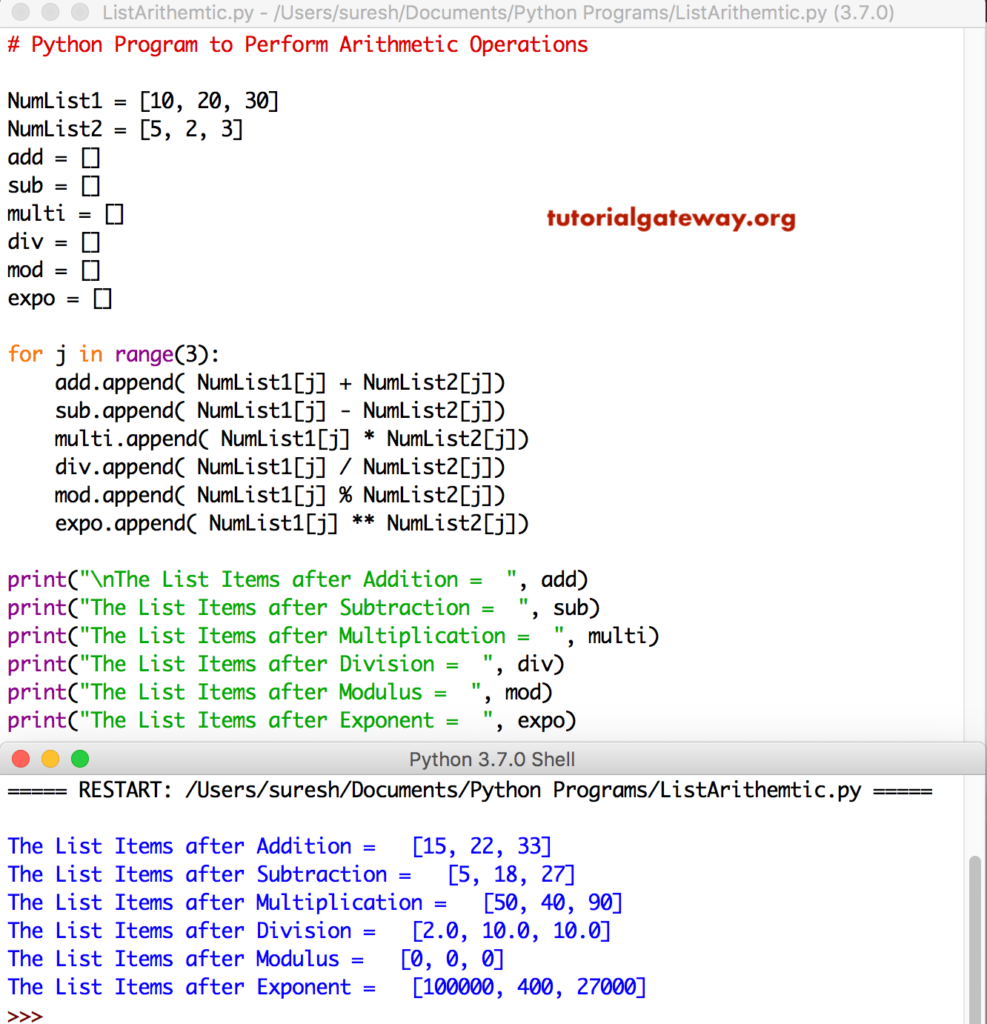
Within this Python Program to Perform Arithmetic Operations on Lists example, NumList1 = [10, 20, 30], NumList2 = [5, 2, 3]. For this, we are using the Python arithmetic Operators
For Loop – First Iteration: for 0 in range(3) – Condition is True
add.append( NumList1[0] + NumList2[0]) => add.append(10 + 5)
add[0] = 15
sub.append( 10 – 5) => sub[0] = 5
multi.append(10 * 5) => multi[0] = 50
div.append( 10 / 5) => div[0] = 2
mod.append( 10 % 5) => sub[0] = 0
expo.append(10 ** 5) => expo[0] = 10000
Second Iteration: for 1 in range(3) – Condition is True
add.append( 20 + 2) => add[1] = 22
sub.append( 20 – 2) => sub[1] = 18
multi.append(20 * 2) => multi[1] = 40
div.append( 20 / 2) => div[1] = 10
mod.append( 20 % 2) => sub[1] = 0
expo.append(20 ** 2) => expo[1] = 400
Third Iteration: for 2 in range(3) – Condition is True
Do the same for this iteration too
Fourth Iteration: for 3 in range(3) – Condition is False
So, it exits from Python For Loop
Perform Arithmetic Operations on Lists using For Loop
In this python program, we are using For Loop to allow users to enter their own number of elements for each List.
# Python Program for Performing Arithmetic Operations on List NumList1 = [] NumList2 = [] add = [] sub = [] multi = [] div = [] mod = [] expo = [] Number = int(input("Please enter the Total Number of List Elements: ")) print("Please enter the Items of a First and Second List ") for i in range(1, Number + 1): List1value = int(input("Please enter the %d Element of List1 : " %i)) NumList1.append(List1value) List2value = int(input("Please enter the %d Element of List2 : " %i)) NumList2.append(List2value) for j in range(Number): add.append( NumList1[j] + NumList2[j]) sub.append( NumList1[j] - NumList2[j]) multi.append( NumList1[j] * NumList2[j]) div.append( NumList1[j] / NumList2[j]) mod.append( NumList1[j] % NumList2[j]) expo.append( NumList1[j] ** NumList2[j]) print("\nThe List Items after Addition = ", add) print("The List Items after Subtraction = ", sub) print("The List Items after Multiplication = ", multi) print("The List Items after Division = ", div) print("The List Items after Modulus = ", mod) print("The List Items after Exponent = ", expo)
Python List Arithmetic Operations using for loop output
Please enter the Total Number of List Elements: 3
Please enter the Items of a First and Second List
Please enter the 1 Element of List1 : 10
Please enter the 1 Element of List2 : 2
Please enter the 2 Element of List1 : 20
Please enter the 2 Element of List2 : 3
Please enter the 3 Element of List1 : 30
Please enter the 3 Element of List2 : 4
The List Items after Addition = [12, 23, 34]
The List Items after Subtraction = [8, 17, 26]
The List Items after Multiplication = [20, 60, 120]
The List Items after Division = [5.0, 6.666666666666667, 7.5]
The List Items after Modulus = [0, 2, 2]
The List Items after Exponent = [100, 8000, 810000]
Perform Arithmetic Operations on Lists using While Loop
This Python program for arithmetic operations on the list is the same as above. We just replaced the For Loop with While loop.
# Python Program to Perform Arithmetic Operations on Lists NumList1 = []; NumList2 = [] add = [] ; sub = [] ; multi = [] div = []; mod = [] ; expo = [] i = 0 j = 0 Number = int(input("Please enter the Total Number of List Elements: ")) print("Please enter the Items of a First and Second List ") while(i < Number): List1value = int(input("Please enter the %d Element of List1 : " %i)) NumList1.append(List1value) List2value = int(input("Please enter the %d Element of List2 : " %i)) NumList2.append(List2value) i = i + 1 while(j < Number): add.append( NumList1[j] + NumList2[j]) sub.append( NumList1[j] - NumList2[j]) multi.append( NumList1[j] * NumList2[j]) div.append( NumList1[j] / NumList2[j]) mod.append( NumList1[j] % NumList2[j]) expo.append( NumList1[j] ** NumList2[j]) j = j + 1 print("\nThe List Items after Addition = ", add) print("The List Items after Subtraction = ", sub) print("The List Items after Multiplication = ", multi) print("The List Items after Division = ", div) print("The List Items after Modulus = ", mod) print("The List Items after Exponent = ", expo)
Python List Arithmetic Operations using a while loop output
Please enter the Total Number of List Elements: 2
Please enter the Items of a First and Second List
Please enter the 0 Element of List1 : 22
Please enter the 0 Element of List2 : 3
Please enter the 1 Element of List1 : 44
Please enter the 1 Element of List2 : 2
The List Items after Addition = [25, 46]
The List Items after Subtraction = [19, 42]
The List Items after Multiplication = [66, 88]
The List Items after Division = [7.333333333333333, 22.0]
The List Items after Modulus = [1, 0]
The List Items after Exponent = [10648, 1936]