Write a Python Program to find the Smallest Number in a List using the min function, for loop, and sort function with a practical example. The min() function returns the least value, the sort() function sorts the list in ascending order, and the first value will be the least.
Python Program to find the Smallest Number in a List using min
In this example, we declared a list of random integer values. The min function returns the minimum value in a List.
a = [10, 50, 60, 80, 20, 15] print(min(a))
10
This Python program is the same as above. But this time, we are allowing the user to enter the length of a List. Next, we used For Loop to add numbers to the Python list.
NumList = [] Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) print("The Smallest Element in this List is : ", min(NumList))

Python Program to find the Smallest Number in a List using the sort function
The sort function sorts List elements in ascending order. Next, we are using Index position 0 to print the first element in a List.
a = [100, 50, 60, 80, 20, 15] a.sort() print(a[0])
20
This list’s smallest number is the same as above. But this time, we allow users to enter their list items.
NumList = [] Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) NumList.sort() print("The Smallest Element in this List is : ", NumList[0])
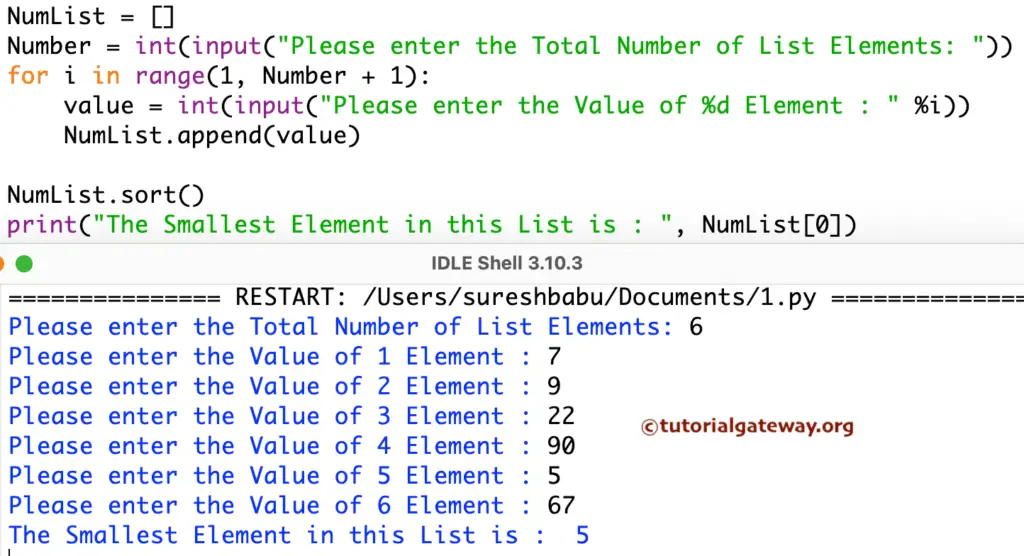
Python Program to find the Smallest Number in a List using for loop
In this program, we are not using any built-in function such as the sort or min function.
NumList = [] Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) smallest = NumList[0] for j in range(1, Number): if(smallest > NumList[j]): smallest = NumList[j] position = j print("The Smallest Element in this List is : ", smallest) print("The Index position of the Smallest Element is : ", position)
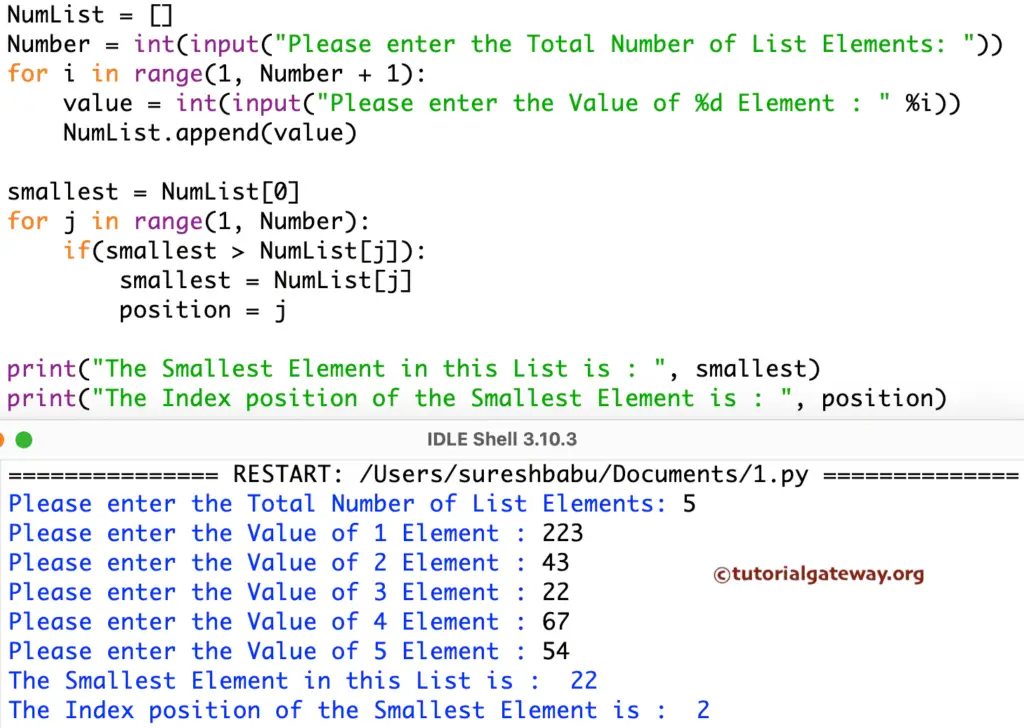
From the above Python Program to find the Smallest Number in a List example, the User inserted values are
NumList[5] = {223, 43, 22, 67, 54}
smallest = NumList[0] = 223
First Iteration – for 1 in range(1, 5) – Condition is true. So, it executes the If statement inside the loop until the condition fails.
if (smallest > NumList[j]) inside the for loop is True because (223 > 43)
smallest = NumList[1] => 43
position = 1
Second Iteration: for 2 in range(1, 5) – Condition is true
If (smallest > NumList[2]) = (43 > 22) – Condition True
smallest = NumList[2] => 22
Position = 2
Third Iteration: for 3 in range(1, 5) – Condition is true
If (smallest > NumList[3]) = (22 > 67) – Condition False
smallest = 22
Position = 2
Fourth Iteration: for 4 in range(1, 5) – Condition is true
If (22 > 54) – Condition False
smallest = 22
Position = 2
Fifth Iteration: for 5 in range(1, 5) – Condition is False. So, it exits from the loop.
If you want the while loop code, replace the for loop with the below code.
j = 1 while j < Number: if(smallest > NumList[j]): smallest = NumList[j] position = j j = j + 1