Write a Python program to find the largest of Two Numbers using Elif Statement and Nested If statement with an example.
Python Program to find Largest of Two Numbers using Elif Statement
Although there are many approaches to find the largest number among the two numbers, we discuss a few of them. This python program for the largest of two numbers, helps the user to enter two different values. Next, the Python program finds the largest number among those two numbers using Elif Statement.
# Python Program to find Largest of Two Numbers a = float(input(" Please Enter the First Value a: ")) b = float(input(" Please Enter the Second Value b: ")) if(a > b): print("{0} is Greater than {1}".format(a, b)) elif(b > a): print("{0} is Greater than {1}".format(b, a)) else: print("Both a and b are Equal")
In this Python Program to find Largest of Two Numbers output, First, we entered the values a = 10, b = 20
Please Enter the First Value a: 10
Please Enter the Second Value b: 20
20.0 is Greater than 10.0
Next, we entered the values a = 10, and b = 10
Please Enter the First Value a: 10
Please Enter the Second Value b: 10
Both a and b are Equal
At last, we entered the values a = 25, b = 15
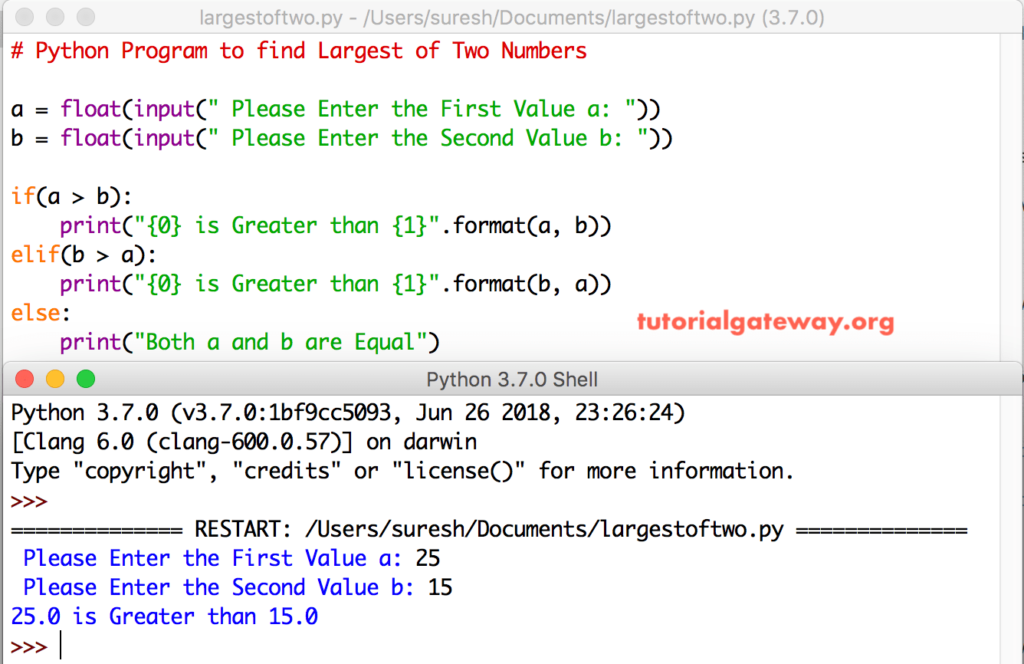
Within this python program to find Largest of Two Numbers example, the following statements ask users to enter two numbers and stores them in variables a, and b
a = float(input(" Please Enter the First Value a: ")) b = float(input(" Please Enter the Second Value b: "))
The Elif Statement is
if(a > b): print("{0} is Greater than {1}".format(a, b)) elif(b > a): print("{0} is Greater than {1}".format(b, a)) else: print("Both a and b are Equal")
- The first if condition checks whether a is greater than b. If True, then a is greater than b printed
- Elif statement checks whether b is greater than a. If True, then b is higher than a printed
- If all the above conditions fail, they are equal.
Python Program to find Largest of Two Numbers using Nested If Statement
In this Python program, it finds the largest number among the two using Nested If.
# Python Program to find Largest of Two Numbers a = float(input(" Please Enter the First Value a: ")) b = float(input(" Please Enter the Second Value b: ")) if(a == b): print("Both a and b are Equal") else: largest = a if a > b else b print("{0} is the Largest Value".format(largest))
Largest of Two numbers using Nested If output 1
Please Enter the First Value a: 89
Please Enter the Second Value b: 78
89.0 is the Largest Value
Largest of Two numbers using Nested If output 2
Please Enter the First Value a: 12
Please Enter the Second Value b: 24
24.0 is the Largest Value
Nested If output to find largest of two numbers 3
Please Enter the First Value a: 3
Please Enter the Second Value b: 3
Both a and b are Equal
Within the Python Program to return the Largest of Two Numbers example, the first if condition checks whether a is equal to b. Inside the Else block, we are using another if statement to check whether a is greater than b or not.
Python Program for Largest of Two Numbers using Arithmetic Operator
In this Python Largest of Two Numbers example, we are using a Minus operator.
# Python Program to find Largest of Two Numbers a = float(input(" Please Enter the First Value a: ")) b = float(input(" Please Enter the Second Value b: ")) if(a - b > 0): print("{0} is Greater than {1}".format(a, b)) elif(b - a > 0): print("{0} is Greater than {1}".format(b, a)) else: print("Both a and b are Equal")
Largest of Two Numbers using Arithmetic Operators output 1
Please Enter the First Value a: 5
Please Enter the Second Value b: 3
5.0 is Greater than 3.0
output 2
Please Enter the First Value a: 9
Please Enter the Second Value b: 15
15.0 is Greater than 9.0
The Largest of Two Numbers using Arithmetic Operators output 3.
Please Enter the First Value a: 20
Please Enter the Second Value b: 20
Both a and b are Equal