Write a Python program to Convert String to Uppercase using the built in function, For Loop, while loop, and ASCII with an example.
Python Program to Convert String using upper Function
This python program allows the user to enter a string. Next, we used a built-in string function to convert lowercase characters in a string to uppercase.
TIP: Please refer String article to understand everything about them in Python.
tx1 = input("Please Enter your Own Text : ") tx2 = tx1.upper() print("\nOriginal = ", tx1) print("Result = ", tx2)
Output of converting Python string to uppercase
Please Enter your Own Text : Code Example
Original = Code Example
Result = CODE EXAMPLE
Python Convert String to Uppercase using For Loop
This python program allows the user to enter a string. Next, it finds lowercase letters and converts them to uppercase.
First, we used For Loop to iterate characters in a String. Inside the For Loop, we are using If Else Statement to check whether the character is between a and z or not. If true, we are subtracting 32 from its ASCII value. Otherwise, we are coping that character to string 1.
TIP: Please refer ASCII Value of Total Characters article and ASCII table to understand the ASCII values.
tsm = input("Please Enter your Own Text : ") tsm1 = '' for i in range(len(tsm)): if(tsm[i] >= 'a' and tsm[i] <= 'z'): tsm1 = tsm1 + chr((ord(tsm[i]) - 32)) else: tsm1 = tsm1 + tsm[i] print("\nOriginal Words = ", tsm) print("The Result of them = ", tsm1)
Please Enter your Own Text : Learn
Original Words = Learn
The Result of them = LEARN
Using While Loop
This python lowercase to uppercase conversion program is the same as above. However, we just replaced the For Loop with While Loop.
txt = input("Please Enter your Own Text : ") txt1 = '' i = 0 while(i < len(txt)): if(txt[i] >= 'a' and txt[i] <= 'z'): txt1 = txt1 + chr((ord(txt[i]) - 32)) else: txt1 = txt1 + txt[i] i = i + 1 print("\nActual Word = ", txt) print("The Result = ", txt1)
Please Enter your Own Text : Tutorial GAtewAy
Actual Word = Tutorial GAtewAy
The Result = TUTORIAL GATEWAY
Python Program to Convert Lowercase String to Uppercase Example 4
This python string uppercase program is the same as the second example. However, we are using For Loop with Object
smp = input("Please Enter your Own Words : ") smp1 = '' for i in smp: if(i >= 'a' and i <= 'z'): smp1 = smp1 + chr((ord(i) - 32)) else: smp1 = smp1 + i print("\nOriginal = ", smp) print("The Result = ", smp1)
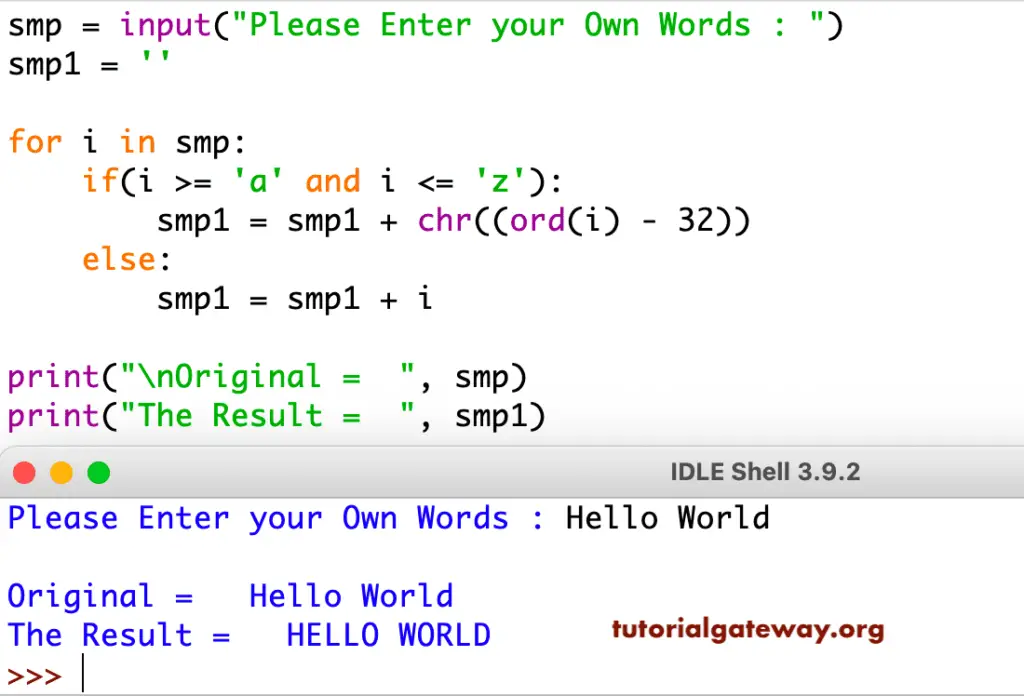
Python Program to Convert Uppercase using ASCII Values
In this program, we are comparing the ASCII values to check whether there are any lowercase characters in this string. If true, we are converting them to uppercase.
stp = input("Please Enter your Own Text : ") stp1 = '' for i in stp: if(ord(i) >= 97 and ord(i) <= 122): stp1 = stp1 + chr((ord(i) - 32)) else: stp1 = stp1 + i print("\nActual = ", stp) print("The Result = ", stp1)
Please Enter your Own Text : Sample InformatIon
Actual = Sample InformatIon
The Result = SAMPLE INFORMATION