Write a Python Program to access the Indexes and Values of a list. In Python, list items are organized based on the index values. So, we can access each list item or value using their indexes. In this Python example, the for loop range (for i in range(len(orgList))) iterates the list items, and the print stamens print the value at each index position.
# Access List Index and Values orgList = [10, 20, 30, 40, 50] print("List Index and Values are") for i in range(len(orgList)): print("Index Number = ", i, " and Value = ", orgList[i])
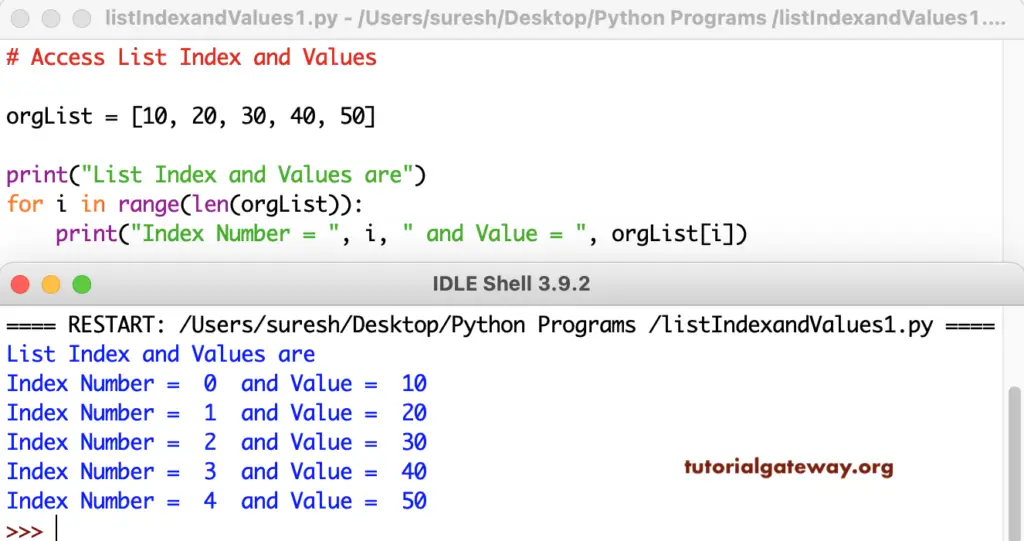
Python Program to access List Index and Values using enumerate
# Access List Index and Values orgList = [2, 4, 6, 8, 10, 12] print("List Index and Values are") for index, value in enumerate(orgList): print("Index Number = ", index, " and Value = ", value)
Python access list index and values using enumerate output
List Index and Values are
Index Number = 0 and Value = 2
Index Number = 1 and Value = 4
Index Number = 2 and Value = 6
Index Number = 3 and Value = 8
Index Number = 4 and Value = 10
Index Number = 5 and Value = 12
In this Python example, we used the list comprehension to access and return the list items or values and the respective index positions.
# Access List Index and Values orgList = [13, 43, 56, 78, 65] list2 = ([list((i, orgList[i])) for i in range(len(orgList))]) print("List Index and Values are") print(list2) list3 = ([(i, orgList[i]) for i in range(len(orgList))]) print("List Index and Values are") print(list3)
Python access list index and values using list comprehension output
List Index and Values are
[[0, 13], [1, 43], [2, 56], [3, 78], [4, 65]]
List Index and Values are
[(0, 13), (1, 43), (2, 56), (3, 78), (4, 65)]
This Python program uses the zip, range, and len functions to return the list indexes and values.
# Access List Index and Values orgList = [22, 44, 66, 88, 122] print("List Index and Values are") for index, value in zip(range(len(orgList)), orgList): print("Index Number = ", index, " and Value = ", value)
Python access list values and index numbers using zip, range and len functions output
List Index and Values are
Index Number = 0 and Value = 22
Index Number = 1 and Value = 44
Index Number = 2 and Value = 66
Index Number = 3 and Value = 88
Index Number = 4 and Value = 122