This Python string function formats the output as per our requirements. This Python function is one of the ways to format strings in the print function.
In this section, we explain to you how to use the string format function in Python Programming with an example, and its syntax is shown below.
{}.format(value)
The following set of examples helps to understand the Python string Format function.
Python format String {} Example
The Python format string method uses curly brackets {} as the replacement placeholders. It means one {} curly bracket accepts one argument.
We declared a string variable within the third line instead of providing the string value directly inside a print statement. Next, we called that msg.
print('{}'.format(25)) msg = 'Tutorial Gateway' print('{}'.format(msg)
25
Tutorial Gateway
How to use {} format as a Python String literal?
This method uses {} as the placeholder for replacement fields. Anything outside the {} is treated as the Python string literal, which prints as it is.
In this Python format string example, the first statement replaces the {} with Hello and prints the World as it is.
The second format string statement replaces the {} after the Employee’s Age is 25.
print("{} World".format("Hello")) print("Employee Age is {}".format(25))
Hello World
Employee Age is 25
How to format Multiple string arguments in Python?
We are using multiple arguments inside this function. In this situation, the Python print format function uses the order we specified inside the format and the string.
For example, within the first statement, the first curly braces fill or replace with Tutorial, and the second curly braces replace with Gateway. It always follows the same order.
print('{} {}'.format('Tutorial', 'Gateway')) print('{} {}'.format('Tutorial', 25))
Tutorial Gateway
Tutorial 25
If we declare two variables, we can replace the text with those string variables inside this function.
Python format String Index Example
So far, we have used the {}. That’s why those curly braces are replaced by the position of arguments in this method. In this example, we used the index values to change the position.
The second statement prints 2nd argument at {1}, the first argument value at {0}, and the third argument value at {2} placeholder.
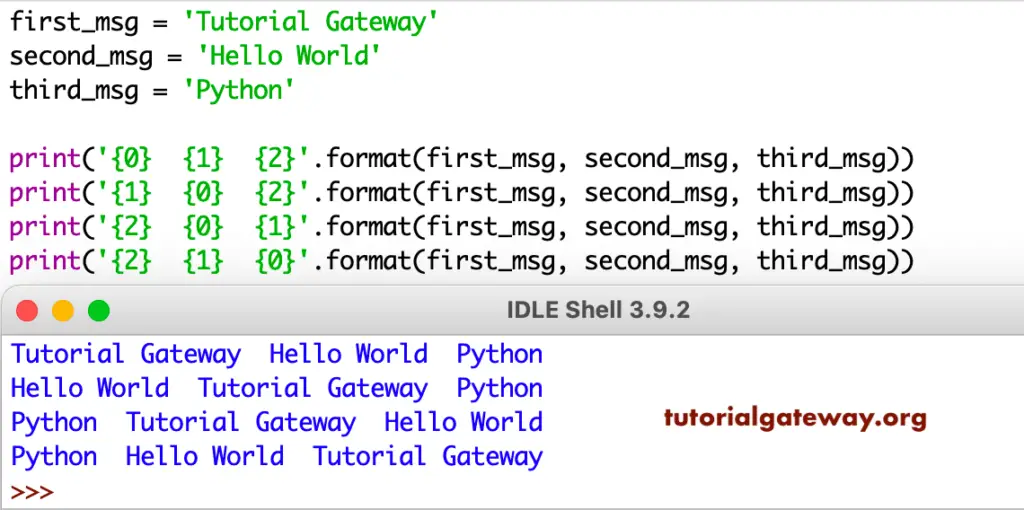
Python format function using Key value
In Python, you can use keyword parameters such as key = value within this string format function.
In this String function example, within the first statement, key1 is lang, and value1 = Py. Next, key2 = com and value2 = Tutorial Gateway. It means the key is replaced by value1 and key2 with value2.
f_msg = 'Tutorial Gateway' s_msg = 'Py' print('Learn {lang} at {com}'.format(lang = s_msg, com = f_msg)) print('{com} Welcomes {lang}'.format(lang = s_msg, com = f_msg))
Learn Py at Tutorial Gateway
Tutorial Gateway Welcomes Py
How to use the Python format function to truncate a string?
You can use this Python format function to truncate the string as well. This example shows how to use the format function to truncate the string value.
The first statement truncates the string to the length of 5. The next statement truncates to length 7, and if we place 10: then it returns the first 10 characters from a string, i.e., Tutorial G.
msg = 'Tutorial Gateway' print('{:.5}'.format(msg)) print('{:.7}'.format(msg))
Tutor
Tutoria
Python format string Options
The following is the list of available Python format options for alignment, signs, binary, etc.
- ^ Aligns the result to the center text.
- < Align the result to the left side. Or left align text.
- > Align the result to the right side. Or right justified text.
- = Place the sign in the leftmost position.
- + Uses this sign to indicate if the result is positive or negative.
- – Use this minus sign for Negative values only.
- ‘ ‘ – For positive numbers, it uses a leading space character.
- , – It uses a comma as a thousand separator.
- _ It uses underscore as a thousand separator.
- b – binary.
- c – converts the given value into a Unicode character.
- d – decimal.
- e – Scientific with e (Lowercase).
- E – Scientific with E (Uppercase).
- f – Fixpoint number.
- F – Uppercase Fixpoint number.
- g – General.
- G – General. For scientific notation, it uses E).
- o – Octal integer.
- x – Hexa Lowercase characters.
- X – Uppercase Hexa.
- n – Number.
- % – Percentage.
Python string format padding
As we said earlier, <, > and ^ are used to align the given numbers or text to the left, right, or center. In this example, we use those operators along with this function to perform the alignment.
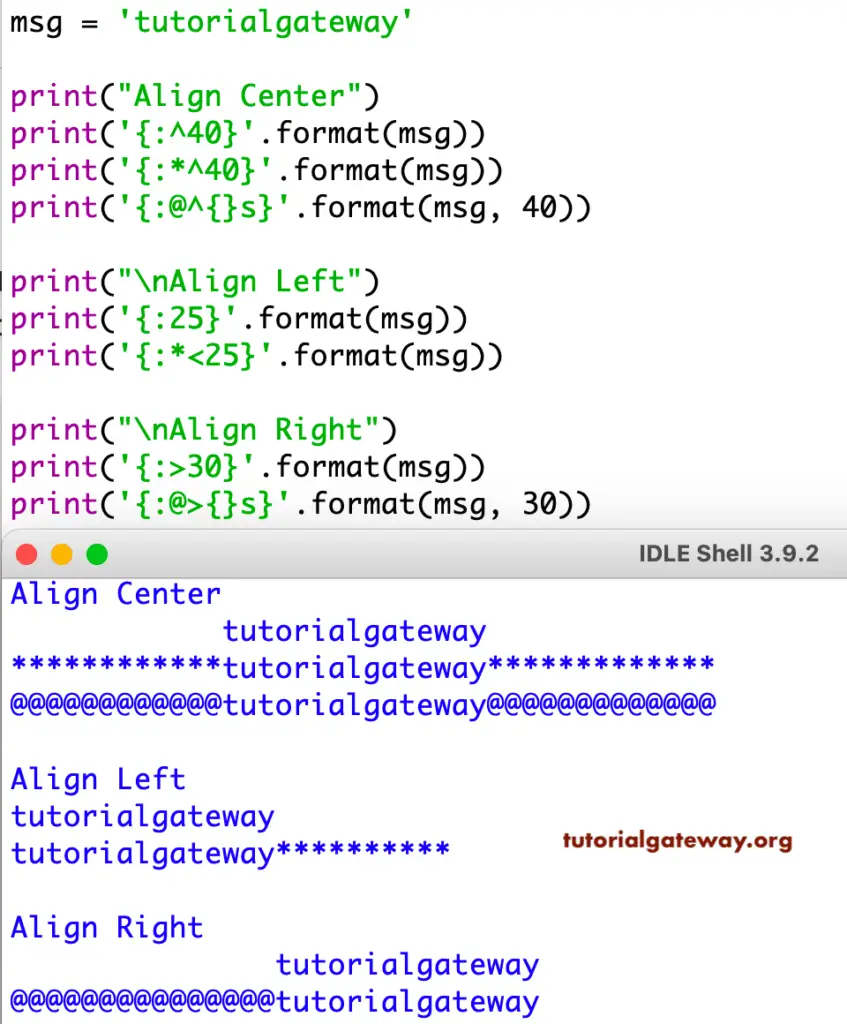
The Python format string padding analysis
- print(‘{:^40}’) returns the message with a width of 40. Here, we used ^ before that 40. It means the message aligns in the center, and the total width is 40 (including spaces and text).
- print(‘{:*^40}’) is the same as the above statement. Instead of showing the empty spaces, we used the * symbol. It means all the empty spaces are filled with * symbols.
- print(‘{:@^{}s}’) – You can also use curly braces as the width value and assign that values as the Python format string function argument. Here, we aligned msg to the right side with a width of 40 and the width filled with @ symbols.
- print(‘{:25}’) or print(‘{:<25}’) returns the message with a width of 25. Here, < aligns the text to the left side and fills the remaining width with empty spaces.
- print(‘{:>30}’) returns the msg with a width of 30. Here, the> symbol aligns the text to the right side and fills the remaining width with empty spaces.
How to format Numbers in Python?
We used the print format method on numbers. The first statement places a space before 95, and the second statement returns a positive sign before 95. Similarly, if we replace + with – to return the negative sign for the negative numbers.
print('{: d}'.format(95)) print('{:+d}'.format(95))
95
+95
Python format or formatting Separators
Use a thousand separators or change the separator. This statement uses a comma as a thousand separator. If we replace {:,} with {:_}, it returns the _ underscore as a thousand separator, i.e., 100_000.
value = 100000 print('{:,}'.format(value))
100,000
You can use this to apply padding to the values. First, we declared positive and negative integers. Next, we used the integer values for padding.
value = 100 value2 = -200 print('{:10d}'.format(value)) print('{:<10d}'.format(value)) print('{:^10d}'.format(value)) print('{:=10d}'.format(value2))
100
100
100
- 200
Padding analysis
- {:10d} means it takes 100 and assigns the width as 10. Here, we haven’t specified the alignment <, >, or ^, so it aligns 10 to the right side (default) with a width of 10.
- {:<10d} means 100 aligns to the left side, and the width = 10.
- {:^10d} align 100 to the center, and the width is 10.
- {:=10d} keeps the negative sign on the left side and 200 on the right side. Here, the total width is 10.
Python format float or floating-point values
You can use the string format built-in function on the float values. I mean, you can restrict the number of decimals, or you can increase the decimal positions.
For example, .2f means only 2 decimal positions. And if we mention .4f ({:4f}, it returns the value with 4 decimal positions by rounding the remaining values to 10.9824.
value = 10.9823764989 print('{:.2f}'.format(value))
10.98
Python format Integers and Decimals Example
Styling the numeric values and float numbers using this string function.
value = 100 f_value = 15.957639 print('{:5d}'.format(value)) print('{:07d}'.format(value)) print('{:6.2f}'.format(f_value)) print('{:012.4f}'.format(f_value))
100
0000100
15.96
0000015.9576
In this format print example,
- {:5d} means it returns 100 with a width of 5. If you specify any alignment, then 100 aligns to that side. Otherwise, by default, it aligns to the right side
- {:07d} means 100 returned with a width of 7. Here, all the empty spaces are filled with 0 because of 07d.
- {:6.2f} returns f_value with two decimal places (.2f). Next, we specified the width as 6. So, it returns f_value with a width of 6.
- {:012.4f} takes 15.957639 and returns it with four decimal places. Here, the width value is 12; we used 0 before that width value. So, 15.9576 return with a width of 12, and the remaining empty spaces are filled with 0’s.
Python format Binary Values
In this section, we are using all the existing style options. First, we declared a value of 10000. Here, we use that value to perform binary, octal, hexadecimal, and numeric functions.
Next, we used different values as Python format string arguments to return the Scientific, general, percentages, and fixed-point numbers.
value = 100000
{:.2f} – 100000.00
{:b} – 11000011010100000
{:o} – 303240
{:x} – 186a0
{:X} – 186A0
{:n} – 100000
{:c} of 120 = x
{:e} of 5.00023455341 = 5.000235e+00
{:E} of 5.00023455341 = 5.000235E+00
{:f} of 25.98723453422 = 25.987235
{:F} of 25.98723453422 = 25.987235
{:g} of 15.987234534 = 15.9872
{:G} of 0.987234534 = 0.987235
{:.2} of 0.987234 = 0.99
{:%} of 0.87 = 87.000000%
Python format List of Strings Example
Using this function to style the List items.
fruits = ['apple', 'mango', 'banana', 'cherry','kiwi'] print('Fruits List = {}'.format(fruits)) print('Fruit Item = {0[1]}'.format(fruits)) print('Fruit Item = {f[1]}'.format(f = fruits)) print('Fruit Item = {}'.format(*fruits))
Fruits List = ['apple', 'mango', 'banana', 'cherry', 'kiwi']
Fruit Item = mango
Fruit Item = mango
Fruit Item = apple
Python format Dictionary items
Use the print function on the dictionary elements.
employee = {'name': 'John', 'age': 25, 'salary': 120000} print('{} is {} years Old'.format(employee['name'], employee['age'])) print('At {1}, {0} is earning {2} income'.format( employee['name'], employee['age'], employee['salary'])) print('-------------') print('{name} is {age} Years Old'.format(**employee)) print('At {age},{name} is earning {salary} income'.format(**employee)) print('-------------') print('{emp[name]} is {emp[age]} Years Old'.format(emp = employee)) print('At {emp[age]}, {emp[name]} is earning {emp[salary]} income'.format(emp = employee))
John is 25 years Old
At 25, John is earning 120000 income
-------------
John is 25 Years Old
At 25,John is earning 120000 income
-------------
John is 25 Years Old
At 25, John is earning 120000 income