Write a Python Program to print Strong numbers from 1 to 100, or 1 to n, or minimum to maximum with an example.
Python Program to print Strong Numbers from 1 to 100
This python program allows the user to enter the maximum limit value. Next, this program prints strong numbers from 1 to user-entered value. Within this python program, first, we used For Loop to iterate a loop between 1 and maximum value. Within the Python for loop,
- We used While Loop to split the given number. So that we can find the factorial of each Digit in a Number.
- Within the While loop, we used the factorial function to find the factorial.
- The if statement checks whether a given number is Strong Number or Not by comparing the original value with the sum of factorials.
TIP: I suggest you refer Factorial, and Strong Number articles to understand the Python logic.
# Python Program to print Strong Numbers from 1 to N import math maximum = int(input(" Please Enter the Maximum Value: ")) for Number in range(1, maximum): Temp = Number Sum = 0 while(Temp > 0): Reminder = Temp % 10 Factorial = math.factorial(Reminder) Sum = Sum + Factorial Temp = Temp // 10 if (Sum == Number): print(" %d is a Strong Number" %Number)
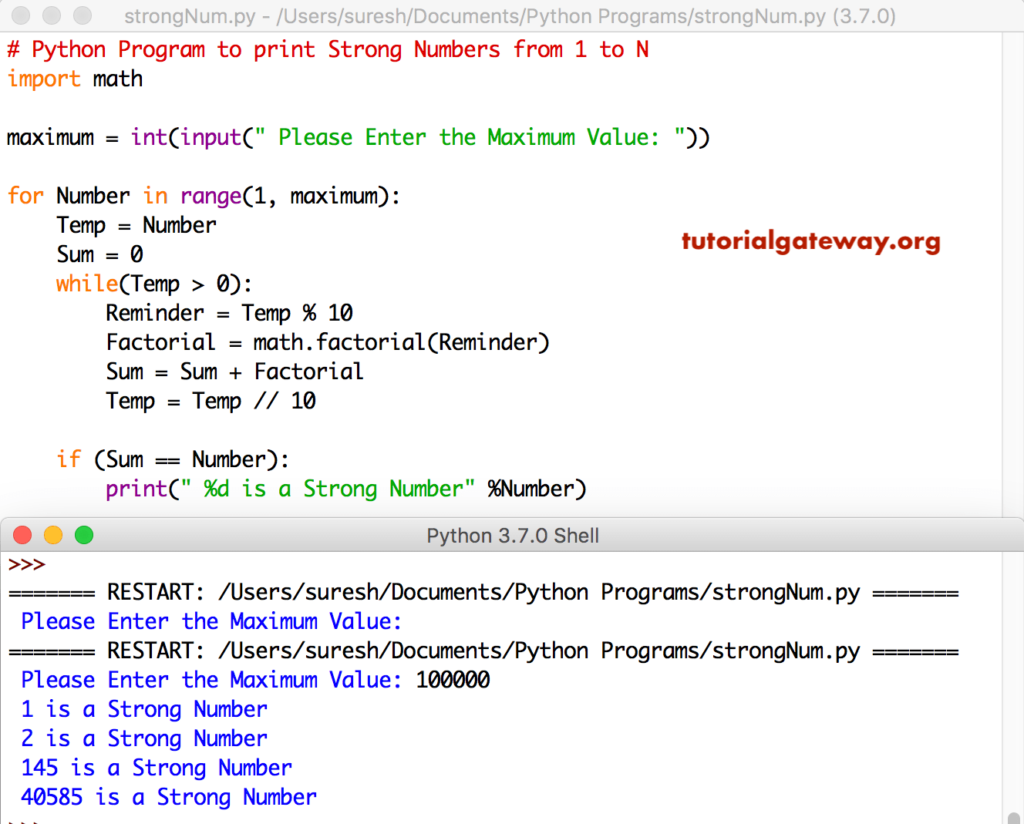
Python Program to print Strong Numbers from 1 to N
In this program, we are allowing the user to enter the minimum and maximum values. Next, this Python program print strong numbers between the minimum and maximum values
import math minimum = int(input(" Please Enter the Minimum Value: ")) maximum = int(input(" Please Enter the Maximum Value: ")) for Number in range(minimum, maximum): Temp = Number Sum = 0 while(Temp > 0): Reminder = Temp % 10 Factorial = math.factorial(Reminder) Sum = Sum + Factorial Temp = Temp // 10 if (Sum == Number): print(" %d is a Strong Number" %Number)
Please Enter the Minimum Value: 10
Please Enter the Maximum Value: 100000
145 is a Strong Number
40585 is a Strong Number