This article shows how to write a Python Program to Print the Right Arrow Star Pattern using a for loop, while loop, and functions. This example uses the nested for loop to iterate and print the Right Arrow Star Pattern.
rows = int(input("Enter Right Arrow Star Pattern Rows = ")) print("====Right Arrow Star Pattern====") for i in range(rows): for j in range(rows): if j < i: print(end = ' ') else: print('*', end = '') print() for i in range(2, rows + 1): for j in range(0, rows): if j < rows - i: print(end = ' ') else: print('*', end = '') print()
Output.
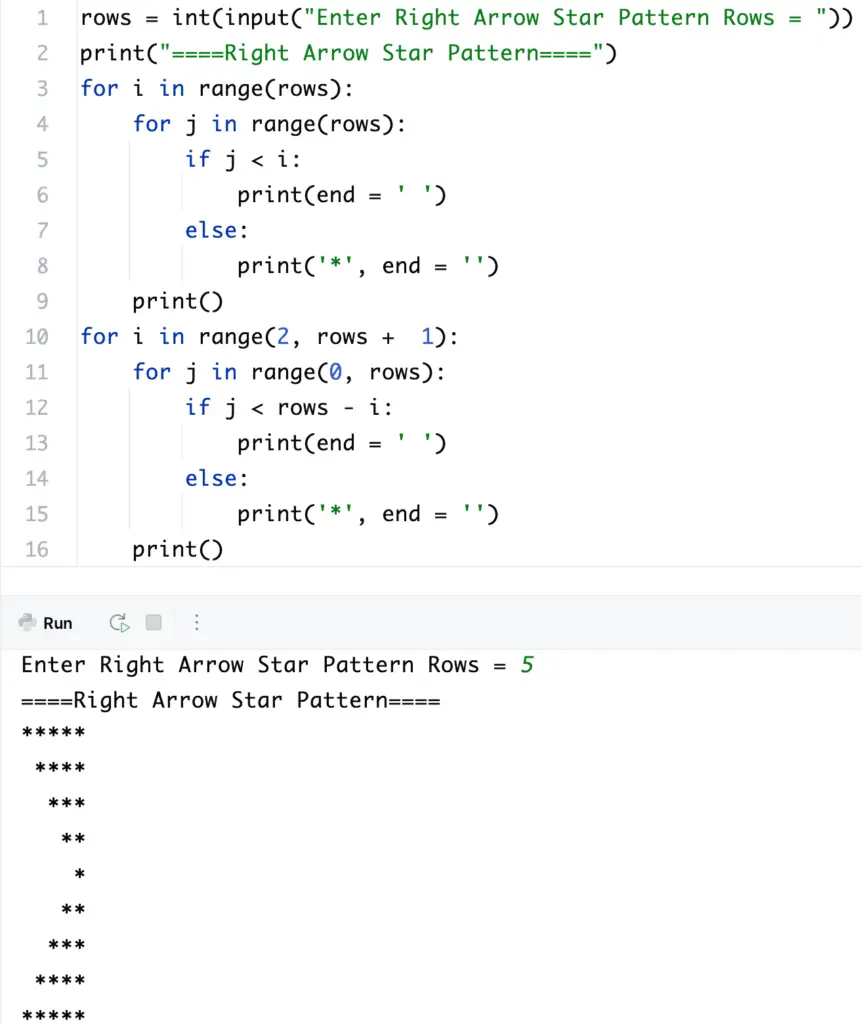
This Python program replaces the above for loop with a while loop to print the Right Arrow Star Pattern.
rows = int(input("Enter Right Arrow Star Pattern Rows = ")) print("====Right Arrow Star Pattern====") i = 0 while i < rows: j =0 while j < rows: if j < i: print(end = ' ') else: print('*', end = '') j = j + 1 i = i + 1 print() i = 2 while i <= rows: j = 0 while j < rows: if j < rows - i: print(end = ' ') else: print('*', end = '') j = j + 1 i = i + 1 print()
Output
Enter Right Arrow Star Pattern Rows = 7
====Right Arrow Star Pattern====
*******
******
*****
****
***
**
*
**
***
****
*****
******
*******
In this Python example, we created a RightArrow function that accepts the rows, i value, and characters to Print the Right Arrow Star Pattern. It replaces the star in the right arrow pattern with a given symbol.
def RightArrow(rows, i, ch): for j in range(i): print(end = ' ') for k in range(i, rows): print('%c' %ch, end = '') print() rows = int(input("Enter Right Arrow Star Pattern Rows = ")) ch = input("Symbol in Right Arrow Pattern = ") for i in range(rows): RightArrow(rows, i, ch) for i in range(rows - 1, -1, -1): RightArrow(rows, i, ch)
Output
Enter Right Arrow Star Pattern Rows = 8
Symbol in Right Arrow Pattern = @
@@@@@@@@
@@@@@@@
@@@@@@
@@@@@
@@@@
@@@
@@
@
@
@@
@@@
@@@@
@@@@@
@@@@@@
@@@@@@@
@@@@@@@@