Write a Python program to print a Pascal triangle number pattern using for loop. To print the Pascal triangle, we must use the nested for loop to iterate multi-level numbers and find the factorial of each number in an iteration.
In this example, we use the built-in math factorial function. Please refer to For loop and while loop to understand the iterations.
from math import factorial rows = int(input("Enter Pascals Triangle Number Pattern Rows = ")) print("====Pascals Triangle Number Pattern====") for i in range(0, rows): for j in range(rows - i + 1): print(end = ' ') for k in range(0, i + 1): print(factorial(i)//(factorial(k) * factorial(i - k)), end = ' ') print()
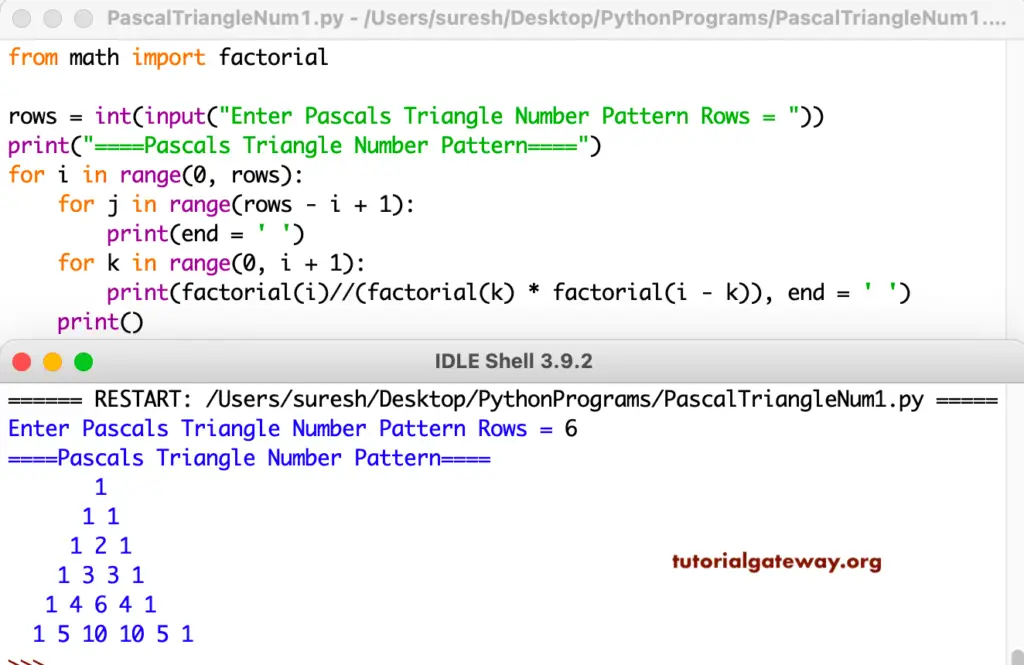
This Python example program prints the Pascal triangle of numbers using a while loop.
# using while loop from math import factorial rows = int(input("Enter Rows = ")) print("====Pattern====") i = 0 while(i < rows): j = 0 while(j <= rows - i): print(end = ' ') j = j + 1 k = 0 while(k <= i): print(factorial(i)//(factorial(k) * factorial(i - k)), end = ' ') k = k + 1 print() i = i + 1
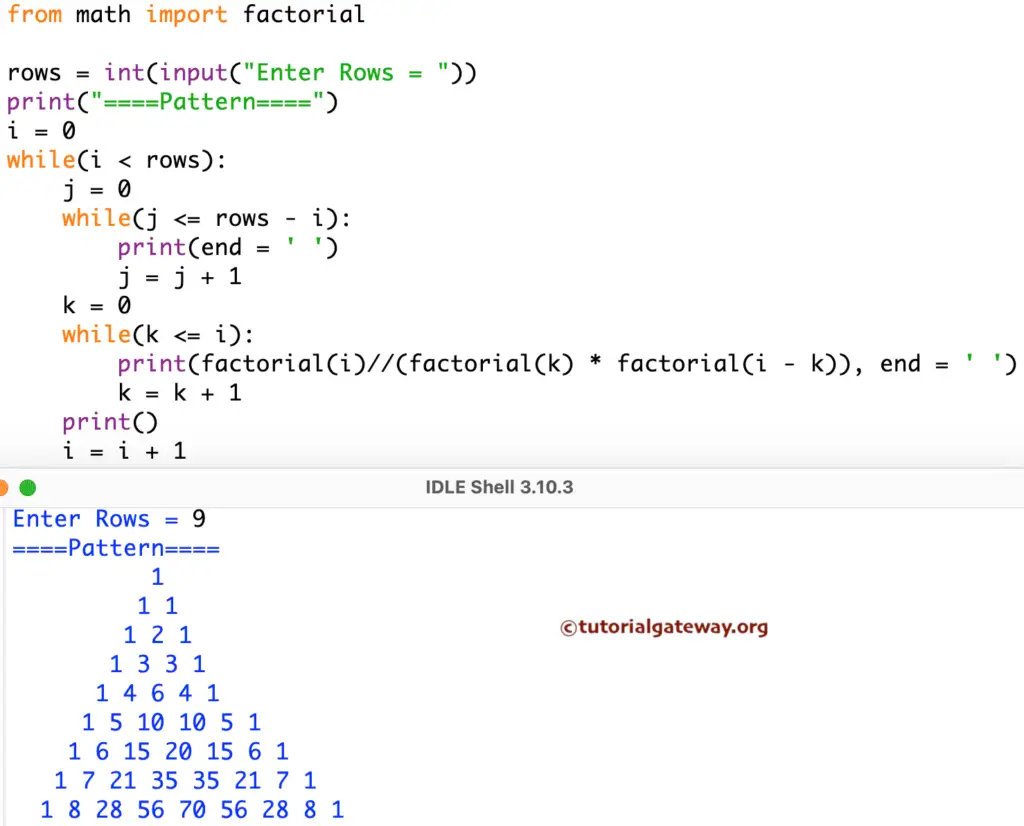