Write a Python Program to Print Even Numbers in a List using For Loop, While Loop, and Functions with a practical example. You can use the loop to iterate the list items and the If statement to check whether the list item is divisible by 2. If True, even number, so, print it.
Python Program to Print Even Numbers in a List using For Loop
In this Python program, we are using For Loop to iterate each element in this List. We are using the If statement inside the loop to check even numbers.
NumList = [] Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) print("\nEven Numbers in this List are : ") for j in range(Number): if(NumList[j] % 2 == 0): print(NumList[j], end = ' ')

In this Python program, the user entered list elements = [22, 56, 7, 87]
For Loop – First Iteration: for 0 in range(0, 4). The For Loop condition is True. So, Python enters into the If Statement.
if(NumList[0] % 2 == 0) => if(22 % 2 == 0) – Condition True. This Number will print.
Second Iteration: for 1 in range(0, 4) – Condition is True
if(NumList[1] % 2 == 0) => if(56 % 2 == 0) – Condition True. So, this number will print.
Third Iteration: for 2 in range(0, 4) – Condition is True
if(NumList[2] % 2 == 0) => if(7 % 2 == 0) – Condition is False. So, this number is skipped.
Fourth Iteration: for 3 in range(0, 4) – Condition is True
if(NumList[3] % 2 == 0) => if(87 % 2 == 0) – Condition is False. So, this number is skipped.
Fifth Iteration: for 4 in range(0, 4) – Condition is False. So, it exits from the For Loop
Python Program to Print Even Numbers in a List using While loop
This example is the same as the above. We just replaced the For Loop with a While loop, and please don’t forget to increment the j value (j = j + 1).
NumList = [] j = 0 Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) print("\nEven Numbers in this List are : ") while(j < Number): if(NumList[j] % 2 == 0): print(NumList[j], end = ' ') j = j + 1

Python Program to Print Even Numbers in a List using Functions
This program is the same as the first example. However, we separated the logic using the Functions.
def even_numbers(NumList): for j in range(Number): if(NumList[j] % 2 == 0): print(NumList[j], end = ' ') NumList = [] Number = int(input("Please enter the Total Number of List Elements: ")) for i in range(1, Number + 1): value = int(input("Please enter the Value of %d Element : " %i)) NumList.append(value) print("\nEven Numbers in this List are : ") even_numbers(NumList)
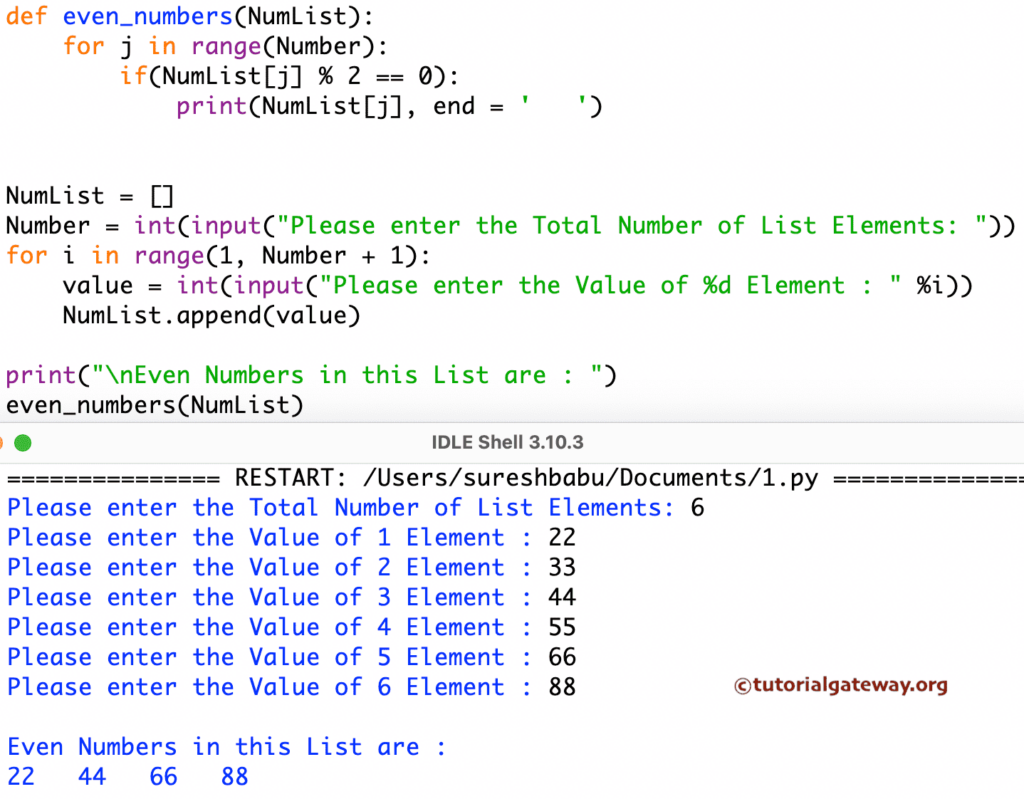