Write a Python Program to Print a Hollow Diamond inside a Square Star Pattern using a for loop, while loop, and functions. This example uses the for loop to iterate and print the Hollow Diamond inside a Square Stars pattern.
rows = int(input("Enter Diamond inside a Square Rows = ")) print("Hollow Diamond inside a Square Star Pattern") for i in range(1, rows + 1): for j in range(i, rows + 1): print('*', end = '') for k in range(1, i * 2 ): print(' ', end = '') for l in range(i, rows + 1): print('*', end = '') print() for i in range(1, rows + 1): for j in range(1, i + 1): print('*', end = '') for k in range(i * 2 - 2, 2 * rows - 1): print(' ', end = '') for l in range(1, i + 1): print('*', end = '') print()
Output.
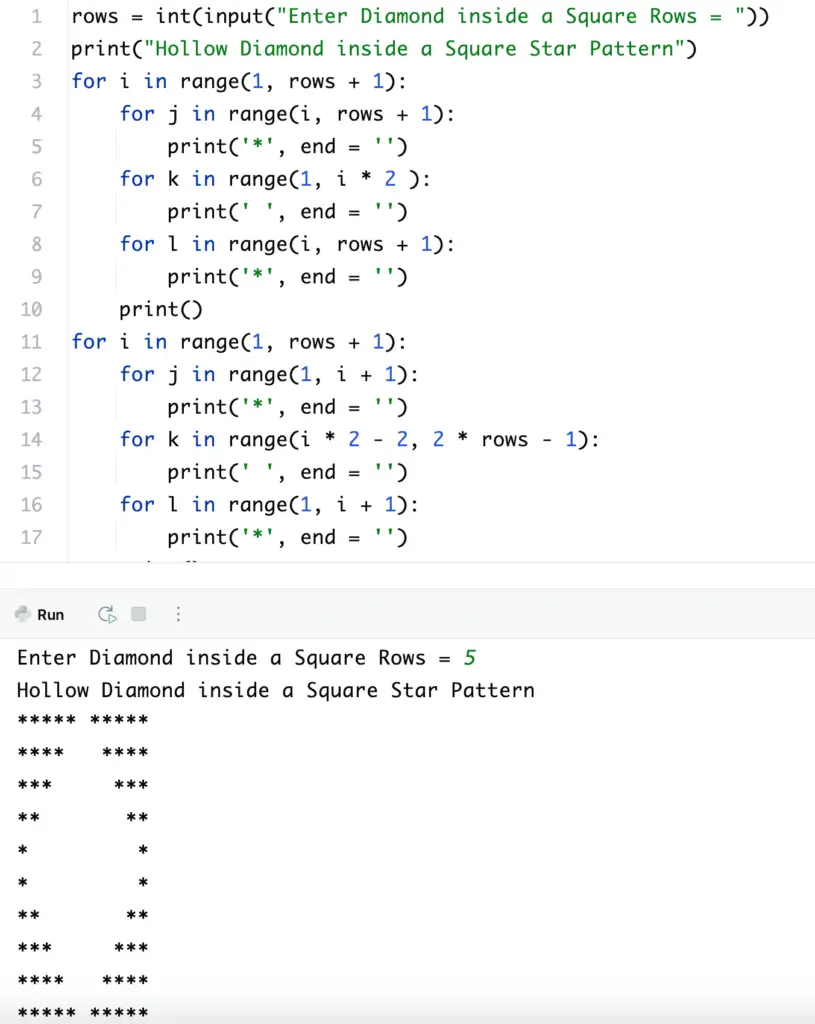
This Python program uses the while loop to print the Hollow Diamond star Pattern inside a Square. We replaced the above for loop with a while loop.
rows = int(input("Enter Diamond inside a Square Rows = ")) print("Hollow Diamond inside a Square Star Pattern") i = 1 while i <= rows: j = i while j <= rows: print('*', end = '') j = j + 1 k = 1 while k < i * 2: print(' ', end = '') k = k + 1 l = i while l <= rows: print('*', end = '') l = l + 1 i = i + 1 print() i = 1 while i <= rows: j = 1 while j <= i: print('*', end = '') j = j + 1 k = i * 2 - 2 while k < 2 * rows - 1: print(' ', end = '') k = k + 1 l = 1 while l <= i: print('*', end = '') l = l + 1 i = i + 1 print()
Output
Enter Diamond inside a Square Rows = 7
Hollow Diamond inside a Square Star Pattern
******* *******
****** ******
***** *****
**** ****
*** ***
** **
* *
* *
** **
*** ***
**** ****
***** *****
****** ******
******* *******
In this Python example, we created firstLoop and secondLoop functions that accept the rows, i value, and characters to Print the Hollow Mirrored Half Diamond Pattern. It replaces the star in the hollow mirrored half diamond pattern with a given symbol.
def firstLoop(rows, i, ch): for j in range(i, rows + 1): print('%c' %ch, end = '') def secondLoop(rows, i, ch): for j in range(1, i + 1): print('%c' %ch, end = '') rows = int(input("Enter Diamond inside a Square Rows = ")) ch = input("Symbol in Diamond inside a Square Pattern = ") print("Hollow Diamond inside a Square Star Pattern") for i in range(1, rows + 1): firstLoop(rows, i, ch) for k in range(1, i * 2 ): print(' ', end = '') firstLoop(rows, i, ch) print() for i in range(1, rows + 1): secondLoop(rows, i, ch) for k in range(i * 2 - 2, 2 * rows - 1): print(' ', end = '') secondLoop(rows, i, ch) print()
Output
Enter Diamond inside a Square Rows = 9
Symbol in Diamond inside a Square Pattern = $
Hollow Diamond inside a Square Star Pattern
$$$$$$$$$ $$$$$$$$$
$$$$$$$$ $$$$$$$$
$$$$$$$ $$$$$$$
$$$$$$ $$$$$$
$$$$$ $$$$$
$$$$ $$$$
$$$ $$$
$$ $$
$ $
$ $
$$ $$
$$$ $$$
$$$$ $$$$
$$$$$ $$$$$
$$$$$$ $$$$$$
$$$$$$$ $$$$$$$
$$$$$$$$ $$$$$$$$
$$$$$$$$$ $$$$$$$$$