Write a Python Program to Multiply All Items in a Dictionary with a practical example.
Python Program to Multiply All Items in a Dictionary Example 1
In this Python program, we are using For Loop to iterate each element in this Dictionary. Inside the for loop, we are multiplying those values by the total variable.
myDict = {'x': 20, 'y':5, 'z':60} print("Dictionary: ", myDict) total = 1 # Multiply Items for key in myDict: total = total * myDict[key] print("\nAfter Multiplying Items in this Dictionary: ", total)
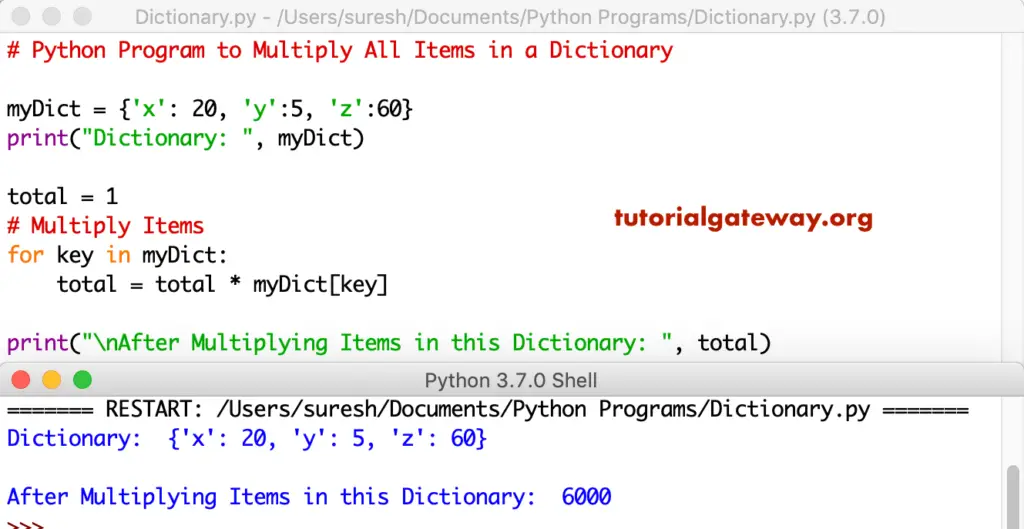
Python Program to Multiply Items in a Dictionary Example 2
This program uses For Loop along with the values function to multiply values in a Dictionary.
myDict = {'x': 2, 'y':50, 'z':70} print("Dictionary: ", myDict) total = 1 # Multiply Items for i in myDict.values(): total = total * i print("\nAfter Multiplying Items in this Dictionary: ", total)
Multiply dictionary items output
Dictionary: {'x': 2, 'y': 50, 'z': 70}
After Multiplying Items in this Dictionary: 7000