Write a Python Program to Map two lists into a Dictionary with a practical example.
Python Program to Map two lists into a Dictionary Example 1
In this python program, we are using for loop with zip function.
keys = ['name', 'age', 'job'] values = ['John', 25, 'Developer'] myDict = {k: v for k, v in zip(keys, values)} print("Dictionary Items : ", myDict)
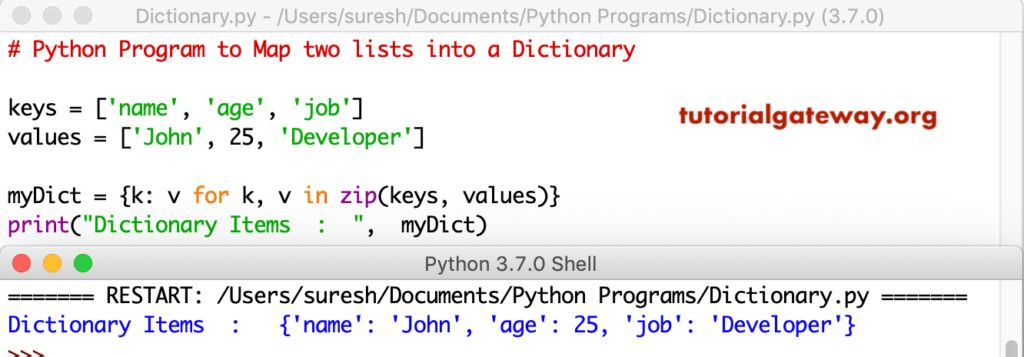
Python Program to insert two lists into a Dictionary Example 2
This code is another approach to inserting lists into a Dictionary. In this Python program, we use a dict keyword and zip function.
keys = ['name', 'age', 'job'] values = ['John', 25, 'Developer'] myDict = dict(zip(keys, values)) print("Dictionary Items : ", myDict)
Map two lists into a Dictionary output
Dictionary Items : {'name': 'John', 'age': 25, 'job': 'Developer'}
>>>
Program to map two lists into a Dictionary Example 3
This map two lists into a Dictionary code is the same as above. However, in this python program, we are allowing the user to insert the keys and values.
keys = [] values = [] num = int(input("Please enter the Number of elements for this Dictionary : ")) print("Integer Values for Keys") for i in range(0, num): x = int(input("Enter Key " + str(i + 1) + " = ")) keys.append(x) print("Integer Values for Values") for i in range(0, num): x = int(input("Enter Value " + str(i + 1) + " = ")) values.append(x) myDict = dict(zip(keys, values)) print("Dictionary Items : ", myDict)
Please enter the Number of elements for this Dictionary : 3
Integer Values for Keys
Enter Key 1 = 2
Enter Key 2 = 4
Enter Key 3 = 6
Integer Values for Values
Enter Value 1 = 50
Enter Value 2 = 100
Enter Value 3 = 150
Dictionary Items : {2: 50, 4: 100, 6: 150}