Write a Python program to make a simple calculator that can perform addition, subtraction, multiplication, and division based on the user selected option.
Python Program to Make a Simple Calculator
The below program prints a message about each option and their respective numbers. Here, option 1 = Add, 2 = Subtract, 3 = Multiply, and 4 = Divide. Next, it asks the user to choose the operation they want. Users who enter options 1, 2, 3, and 4 are valid; anything other than those is invalid input.
In the following line, we used two input() statements of integer type to allow users to enter two numbers. Next, the elif statement performs simple calculations based on the user option.
print('''please Select the Operation you want to Perfom 1 = Add 2 = Subtract 3 = Multiply 4 = Divide''') opt = int(input("Choose Operation from 1, 2, 3, 4 = ")) n1 = int(input("First Number = ")) n2 = int(input("Second Number = ")) if opt == 1: print(n1, ' + ', n2, ' = ', n1 + n2) elif opt == 2: print(n1, ' - ', n2, ' = ', n1 - n2) elif opt == 3: print(n1, ' * ', n2, ' = ', n1 * n2) elif opt == 4: print(n1, ' / ', n2, ' = ', n1 / n2) else: print('Invalid Input')
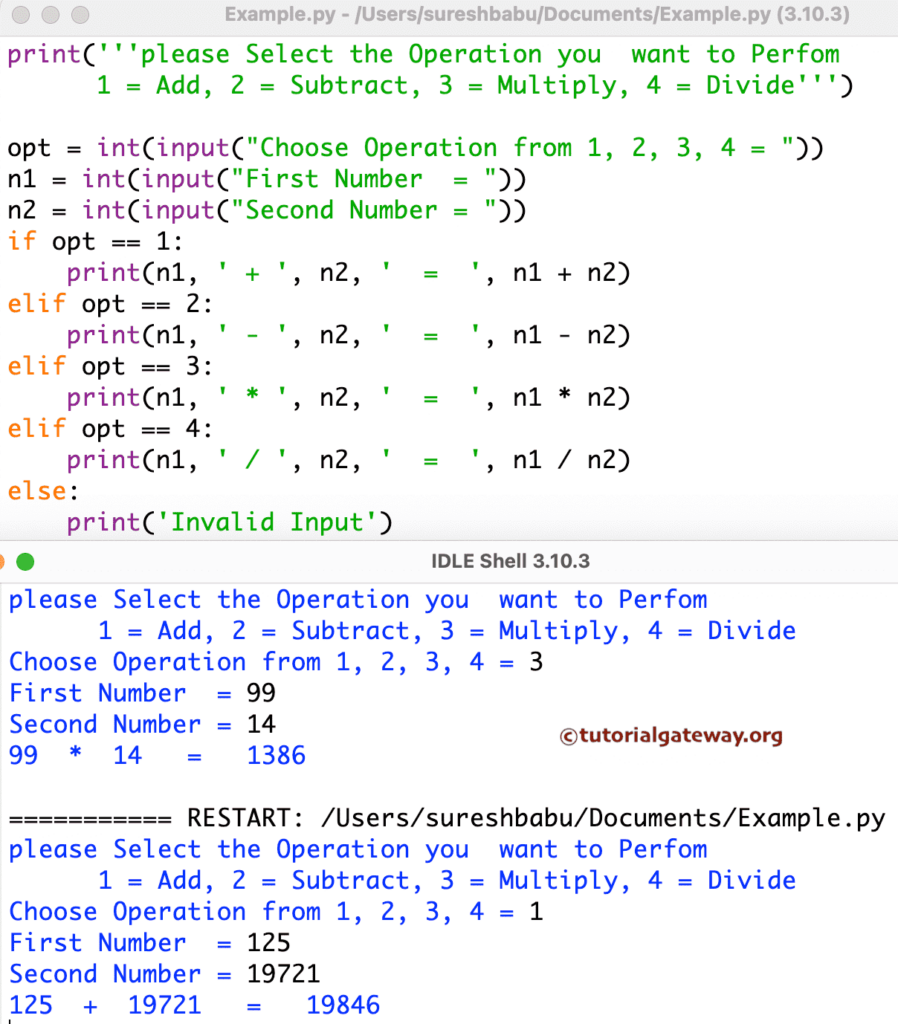
Simple Calculator using Functions
In this Python calculator program, we created addition(x, y), subtraction(x, y), multiplication(x, y), and division(x, y) functions to do calculations. Within the print, we are directly calling those functions.
def addition(x, y): return x + y def subtraction(x, y): return x - y def multiplication(x, y): return x * y def division(x, y): return x / y opt = int(input("Choose Operation from 1(Add), 2(Sub), 3(Multi), 4(Div) = ")) n1 = int(input("First Number = ")) n2 = int(input("Second Number = ")) if opt == 1: print(n1, ' + ', n2, ' = ', addition(n1, n2)) elif opt == 2: print(n1, ' - ', n2, ' = ', subtraction(n1, n2)) elif opt == 3: print(n1, ' * ', n2, ' = ', multiplication(n1, n2)) elif opt == 4: print(n1, ' / ', n2, ' = ', divison(n1, n2)) else: print('Invalid Input')
