Write a Python Program to Find the sum of numpy array items. The numpy sum function returns the sum of all the array items. We use this sum function on an integer array.
import numpy as np arr = np.array([10, 20, 40, 70, 90]) total = sum(arr) print("The Sum of Total Array Item = ", total)
The sum of numpy Array items using the sum function output.
The Sum of Total Array Item = 230
It allows the user to enter the numpy ndarray size and the items. Next, we used the numpy sum function to get the sum of those array items.
import numpy as np arrSumList = [] number = int(input("Enter the Total Array Items = ")) for i in range(1, number + 1): value = int(input("Enter the %d Array value = " %i)) arrSumList.append(value) intarrSum = np.array(arrSumList) total = sum(intarrSum) print("The Sum of Total Array Item = ", total)
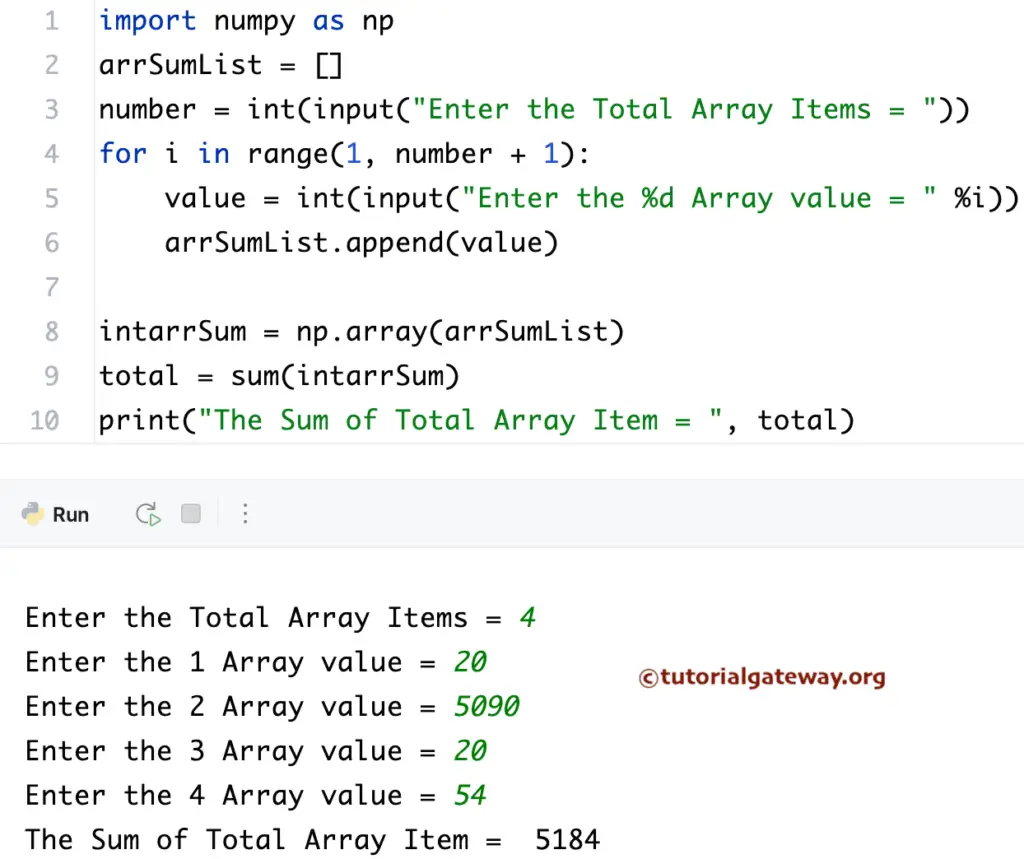
Python Program to Find Sum of Numpy Array items using For Loop range
In this for loop (for i in range(len(sumArr))), i value iterates from array index position 0 to the length of this sumArr. Within this for loop, we are adding each item to the total (total = total + sumArr[I]).
import numpy as np sumArr = np.array([10, 60, 30, 40, 70, 95]) total = 0 for i in range(len(sumArr)): total = total + sumArr[i] print("The Sum of Total Array Item = ", total)
The Sum of Total Array Item = 305
In this numpy example, the for loop (for num in sumArr) iterates the actual array items, not the index position, and adds those items.
import numpy as np sumArr = np.array([10, 30, 50, 70, 90, 120, 150]) total = 0 for num in sumArr: total = total + num print("The Sum of Total Array Item = ", total)
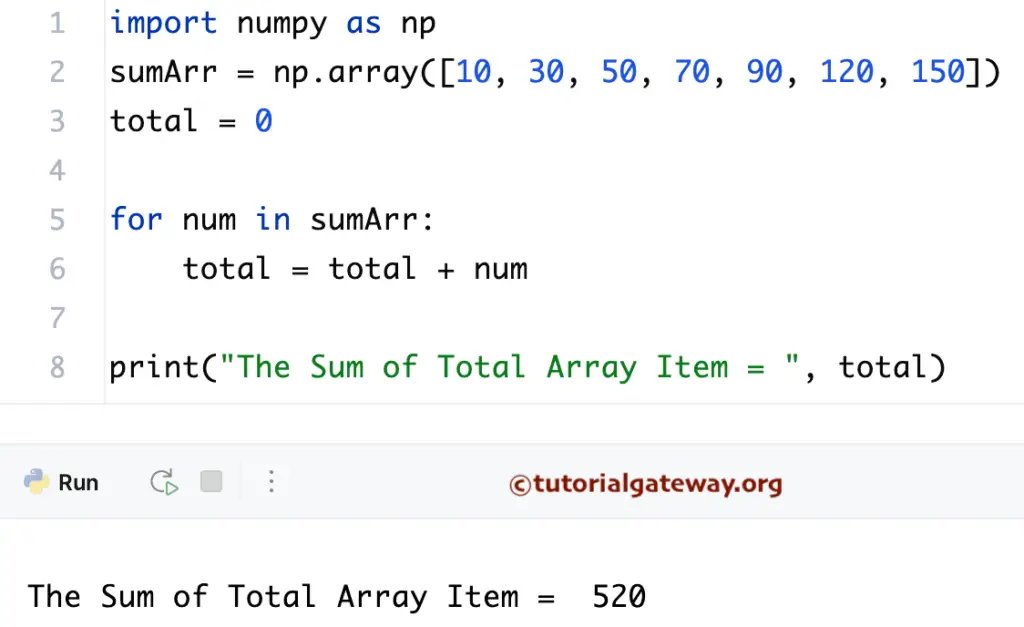
The sum of Array items using while loop
This Program helps to Calculate the Sum of Numpy Array items or elements using a While loop.
import numpy as np sumArr = np.array([15, 66, 125, 30, 50, 95]) total = 0 i = 0 while (i < len(sumArr)): total = total + sumArr[i] i = i + 1 print("The Sum of Total Array Item = ", total)
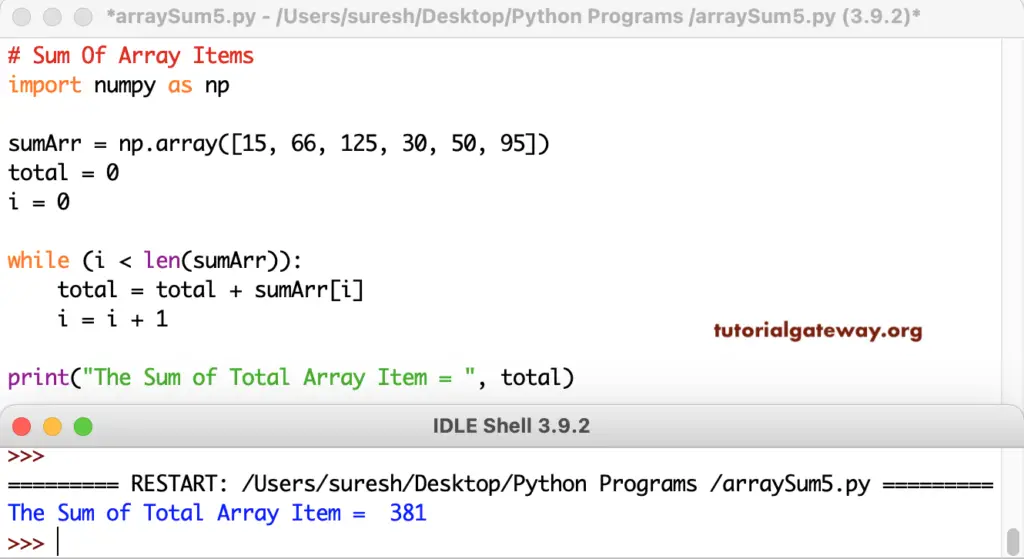