Write a Python Program to find Sum and Average of N Natural Numbers using While Loop, For Loop, and Functions with an example.
Python Program to find Sum and Average of N Natural Numbers using For Loop
This program allows users to enter any integer value. Next, this program calculates the sum and average of natural numbers from 1 to a user-specified value using For Loop.
number = int(input("Please Enter any Number: ")) total = 0 for value in range(1, number + 1): total = total + value average = total / number print("The Sum of Natural Numbers from 1 to {0} = {1}".format(number, total)) print("Average of Natural Numbers from 1 to {0} = {1}".format(number, average))
The sum and average of natural numbers using for Loop output
Please Enter any Number: 5
The Sum of Natural Numbers from 1 to 5 = 15
Average of Natural Numbers from 1 to 5 = 3.0
Python Program to Calculate Sum and Average of N Natural Numbers using While Loop
In this Program to find the Sum and Average of Natural Numbers, we replaced the For Loop with While Loop. Please refer C example to understand this Python code execution in iteration wise.
number = int(input("Please Enter any Number: ")) total = 0 value = 1 while (value <= number): total = total + value value = value + 1 average = total / number print("The Sum of Natural Numbers from 1 to {0} = {1}".format(number, total)) print("Average of Natural Numbers from 1 to {0} = {1}".format(number, average))
The sum and average of natural numbers using while Loop output
Please Enter any Number: 10
The Sum of Natural Numbers from 1 to 10 = 55
Average of Natural Numbers from 1 to 10 = 5.5
Python Program to calculate Sum and Average of N Natural Numbers using Functions
In this Program, we created a new function to find the sum and average of natural numbers. Inside this function, we used the If Else statement
def sum_and_avg_of_natural_numbers(num): if(num == 0): return num else: return (num * (num + 1) / 2) number = int(input("Please Enter any Number: ")) total = sum_and_avg_of_natural_numbers(number) average = total / number print("The Sum of Natural Numbers from 1 to {0} = {1}".format(number, total)) print("Average of Natural Numbers from 1 to {0} = {1}".format(number, average))
The sum and average of natural numbers using functions output
Please Enter any Number: 100
The Sum of Natural Numbers from 1 to 100 = 5050.0
Average of Natural Numbers from 1 to 100 = 50.5
Python Program for Sum and Average of N Natural Numbers using Recursion
This program to find the Sum and Average of Natural Numbers is the same as the above example, but this time we are using Recursion.
def sum_and_avg_of_natural_numbers(num): if(num == 0): return num else: return (num + sum_and_avg_of_natural_numbers(num - 1)) number = int(input("Please Enter any Number: ")) total = sum_and_avg_of_natural_numbers(number) average = total / number print("The Sum of Natural Numbers from 1 to {0} = {1}".format(number, total)) print("Average of Natural Numbers from 1 to {0} = {1}".format(number, average))
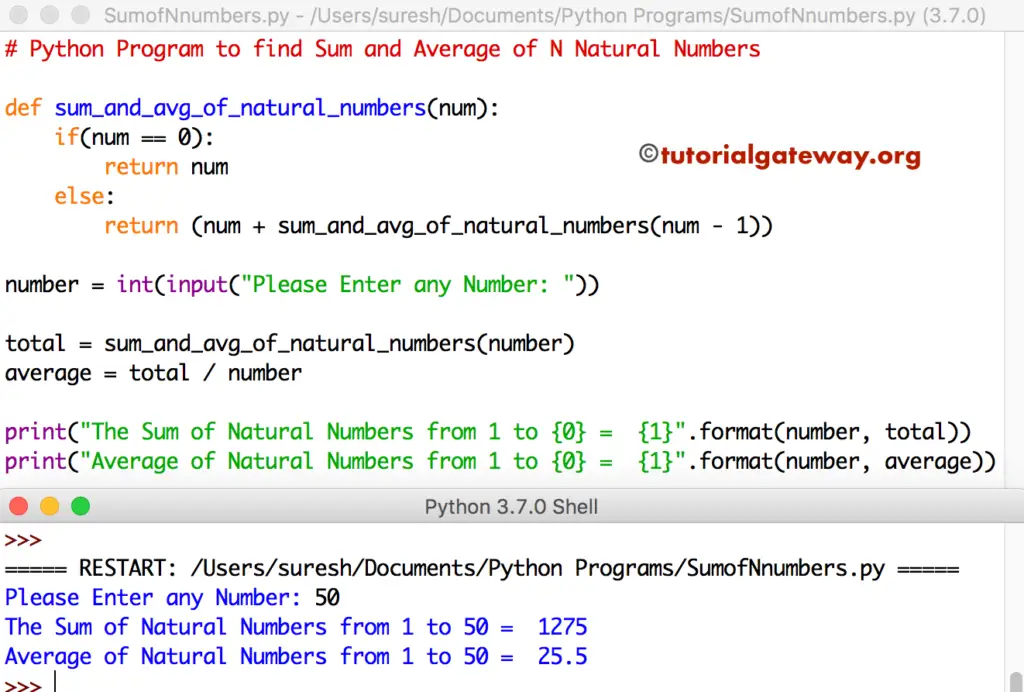