Write a Python Program to Find the Smallest or Minimum Item in a Tuple. Here, we use the Tuple min function (min(smTuple))) to return the Smallest Tuple item.
# Tuple Min Item smTuple = (9, 11, 22, 45, 33, 5, 17, 30) print("Tuple Items = ", smTuple) print("Smallest Item in smTuple Tuple = ", min(smTuple))
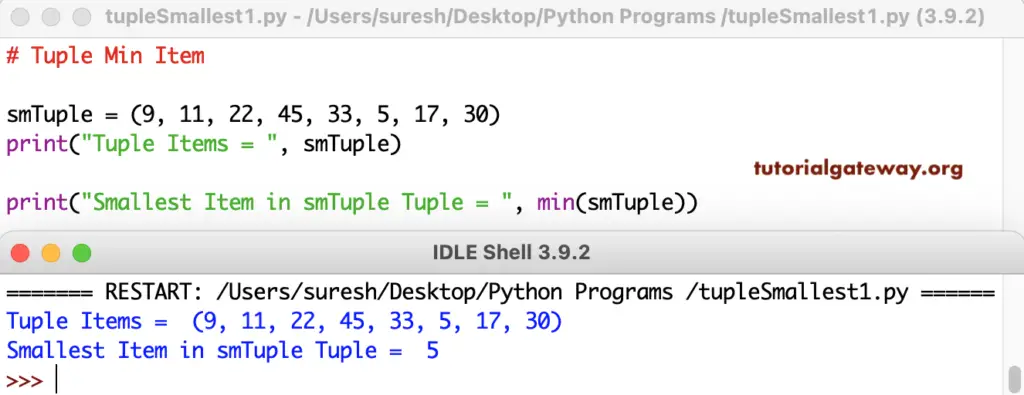
Python Program to Find Smallest Item in a Tuple
We used the Tuple sorted function (sorted(smTuple)) to sort the Tuple in ascending order and print the first item, the Smallest.
# Tuple Min Item smTuple = (14, 19, 11, 25, 7, 22, 30) print("Tuple Items = ", smTuple) smTuple = sorted(smTuple) print("Smallest Item in smTuple Tuple = ", smTuple[0])
Tuple Items = (14, 19, 11, 25, 7, 22, 30)
Smallest Item in smTuple Tuple = 7
In this Python example, we assigned the Tuple first value as the Smallest (tupSmallest = smTuple[0]), and the for loop range starts at one and traverses to smTuple length. The if condition (if(tupSmallest > smTuple[I])) examines whether the current Tuple item is less than the Smallest. If True, assign that Tuple value to the Smallest and the index value to the tupSmallestPos variable.
# Tuple Min Item smTuple = (25, 33, 55, 17, 2, 40, 30, 65, 29) print("Tuple Items = ", smTuple) tupSmallest = smTuple[0] for i in range(len(smTuple)): if(tupSmallest > smTuple[i]): tupSmallest = smTuple[i] tupSmallestPos = i print("Smallest Item in smTuple Tuple = ", tupSmallest) print("Smallest Tuple Item index Position = ", tupSmallestPos)
Tuple Items = (25, 33, 55, 17, 2, 40, 30, 65, 29)
Smallest Item in smTuple Tuple = 2
Smallest Tuple Item index Position = 4
Python Program to return the Tuples Smallest Number using Functions.
# Tuple Max Item def tupleSmallest(smTuple): tupSmallest = smTuple[0] for i in smTuple: if(tupSmallest > i): tupSmallest = i return tupSmallest smTuple = (19, 77, 13, 87, 33, 6, 17, 45, 66) print("Tuple Items = ", smTuple) smt = tupleSmallest(smTuple) print("Smallest Item in smTuple Tuple = ", smt)
Tuple Items = (19, 77, 13, 87, 33, 6, 17, 45, 66)
Smallest Item in smTuple Tuple = 6