Write a Python Program to Find the Largest or Maximum Item in a Tuple. Here, we use the Tuple max function to return the largest Tuple item.
# Tuple Max Item mxTuple = (3, 78, 67, 34, 88, 11, 23, 19) print("Tuple Items = ", mxTuple) print("Largest Item in mxTuple Tuple = ", max(mxTuple))
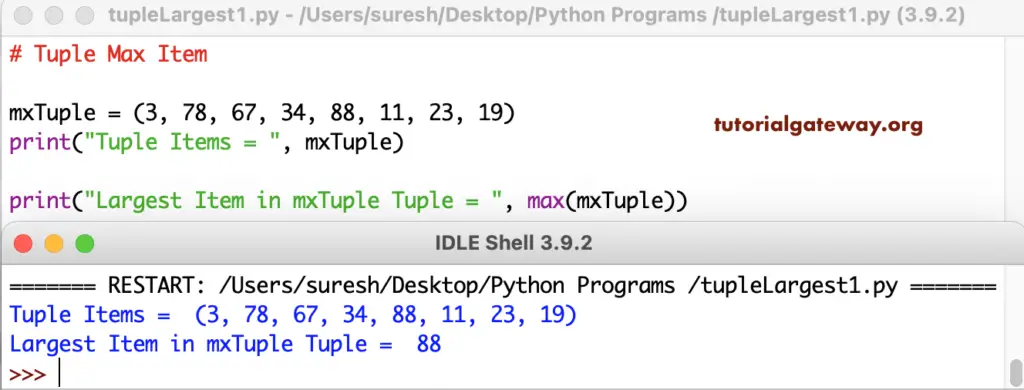
Python Program to Find Largest Item in a Tuple.
We used the Tuple sorted function (sorted(mxTuple)) to sort the Tuple in ascending order. Next, we are printing the last index position item, which is the largest.
# Tuple Max Item mxTuple = (2, 14, 11, 19, 25, 17, 33, 22, 30) print("Tuple Items = ", mxTuple) mxTuple = sorted(mxTuple) print("Largest Item in mxTuple Tuple = ", mxTuple[len(mxTuple) - 1])
Tuple Items = (2, 14, 11, 19, 25, 17, 33, 22, 30)
Largest Item in mxTuple Tuple = 33
In this Python example, we assigned the Tuple first value as the largest (tupLargest = mxTuple[0]), and the for loop range starts at one and traverses to mxTuple length. The if condition (if(tupLargest < mxTuple[I])) examines whether the current Tuple item is greater than the largest. If True, assign that Tuple value to the largest and the index value to the tupLargestPos variable.
# Tuple Max Item mxTuple = (25, 17, 19, 33, 55, 22, 40, 30, 50, 29) print("Tuple Items = ", mxTuple) tupLargest = mxTuple[0] for i in range(1, len(mxTuple)): if(tupLargest < mxTuple[i]): tupLargest = mxTuple[i] tupLargestPos = i print("Largest Item in mxTuple Tuple = ", tupLargest) print("Largest Tuple Item index Position = ", tupLargestPos)
Tuple Items = (25, 17, 19, 33, 55, 22, 40, 30, 50, 29)
Largest Item in mxTuple Tuple = 55
Largest Tuple Item index Position = 4
Python Program to Print the Tuples Largest Number using Functions.
# Tuple Max Item def tupleLargest(mxTuple): tupLargest = mxTuple[0] for i in mxTuple: if(tupLargest < i): tupLargest = i return tupLargest mxTuple = (19, 25, 77, 56, 89, 45, 55, 12) print("Tuple Items = ", mxTuple) lar = tupleLargest(mxTuple) print("Largest Item in mxTuple Tuple = ", lar)
Tuple Items = (19, 25, 77, 56, 89, 45, 55, 12)
Largest Item in mxTuple Tuple = 89