Write a Python Program to Count Positive and Negative Numbers in Tuple using the for loop range. The if condition (if (posngTuple[i] >= 0)) checks whether the Tuple item is greater than or equals to zero. If True, we add one to the Positive Tuple count; otherwise (tNegativeCount = tNegativeCount + 1), add one to the Negative Tuple count value.
# Count Positive and Negative Numbers posngTuple = (3, -22, -44, 19, -99, -37, 4, 11, -89) print("Positive and Negative Tuple Items = ", posngTuple) tPositiveCount = tNegativeCount = 0 for i in range(len(posngTuple)): if (posngTuple[i] >= 0): tPositiveCount = tPositiveCount + 1 else: tNegativeCount = tNegativeCount + 1 print("The Count of Positive Numbers in posngTuple = ", tPositiveCount) print("The Count of Negative Numbers in posngTuple = ", tNegativeCount)
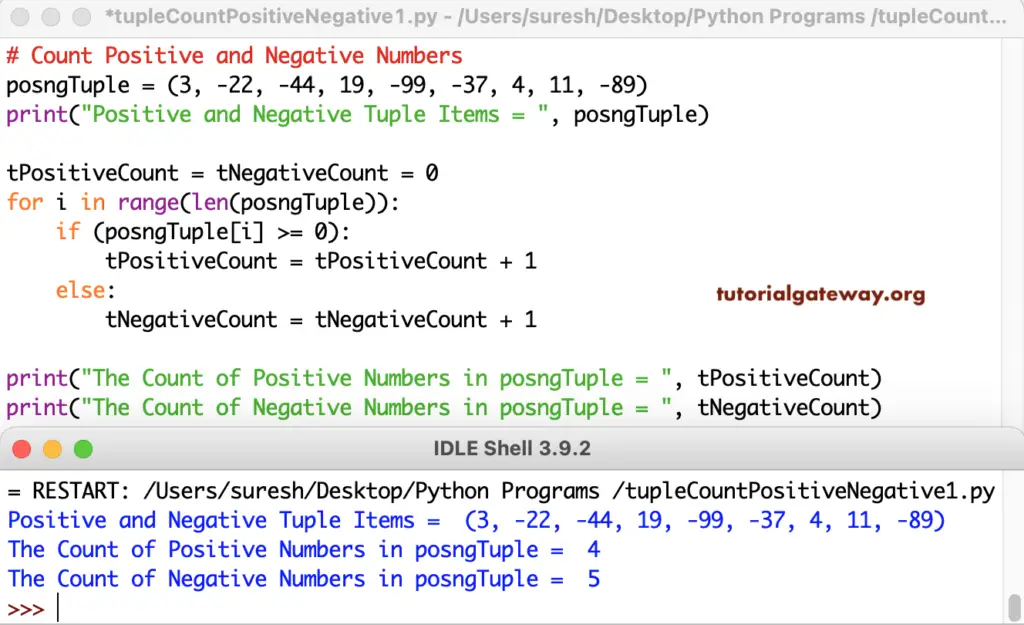
Python Program to Count Positive and Negative Numbers in Tuple
In this Python Positive and Negative example, we used the for loop (for pntup in posngTuple) to iterate the actual tuple values and inspect whether they are greater than or equal to zero.
# Count Positive and Negative Numbers posngTuple = (55, -99, -88, 0, -78, 22, 4, -66, 21, 33) print("Positive and Negative Tuple Items = ", posngTuple) tPositiveCount = tNegativeCount = 0 for pntup in posngTuple: if(pntup >= 0): tPositiveCount = tPositiveCount + 1 else: tNegativeCount = tNegativeCount + 1 print("The Count of Positive Numbers in posngTuple = ", tPositiveCount) print("The Count of Negative Numbers in posngTuple = ", tNegativeCount)
Positive and Negative Tuple Items = (55, -99, -88, 0, -78, 22, 4, -66, 21, 33)
The Count of Positive Numbers in posngTuple = 6
The Count of Negative Numbers in posngTuple = 4
Python Program to Count the Positive and Negatives in Tuple using the While Loop.
# Count of Tuple Positive and Negative Numbers posngTuple = (11, -22, -33, 44, 55, -66, -77, 0, -99) print("Positive and Negative Tuple Items = ", posngTuple) tPositiveCount = tNegativeCount = 0 i = 0 while (i < len(posngTuple)): if(posngTuple[i] >= 0): tPositiveCount = tPositiveCount + 1 else: tNegativeCount = tNegativeCount + 1 i = i + 1 print("The Count of Positive Numbers in posngTuple = ", tPositiveCount) print("The Count of Negative Numbers in posngTuple = ", tNegativeCount)
Positive and Negative Tuple Items = (11, -22, -33, 44, 55, -66, -77, 0, -99)
The Count of Positive Numbers in posngTuple = 4
The Count of Negative Numbers in posngTuple = 5
In this Python Tuple example, we created a function that returns the Positive and Negative numbers count.
# Count of Tuple Positive and Negative Numbers def CountOfPositiveNegativeNumbers(evodTuple): tPositiveCount = tNegativeCount = 0 for pntup in evodTuple: if(pntup >= 0): tPositiveCount = tPositiveCount + 1 else: tNegativeCount = tNegativeCount + 1 return tPositiveCount, tNegativeCount evodTuple = (26, -77, -99, 75, 14, -56, 19, 81, -1, 33) print("Positive and Negative Tuple Items = ", evodTuple) PositiveCount, NegativeCount = CountOfPositiveNegativeNumbers(evodTuple) print("The Count of Positive Numbers in evodTuple = ", PositiveCount) print("The Count of Negative Numbers in evodTuple = ", NegativeCount)
Positive and Negative Tuple Items = (26, -77, -99, 75, 14, -56, 19, 81, -1, 33)
The Count of Positive Numbers in evodTuple = 6
The Count of Negative Numbers in evodTuple = 4