Write a Python Program to Count Occurrence of a Character in a String with a practical example. This python program allows you to enter a string and a character.
# Python Program to Count Occurrence of a Character in a String string = input("Please enter your own String : ") char = input("Please enter your own Character : ") count = 0 for i in range(len(string)): if(string[i] == char): count = count + 1 print("The total Number of Times ", char, " has Occurred = " , count)
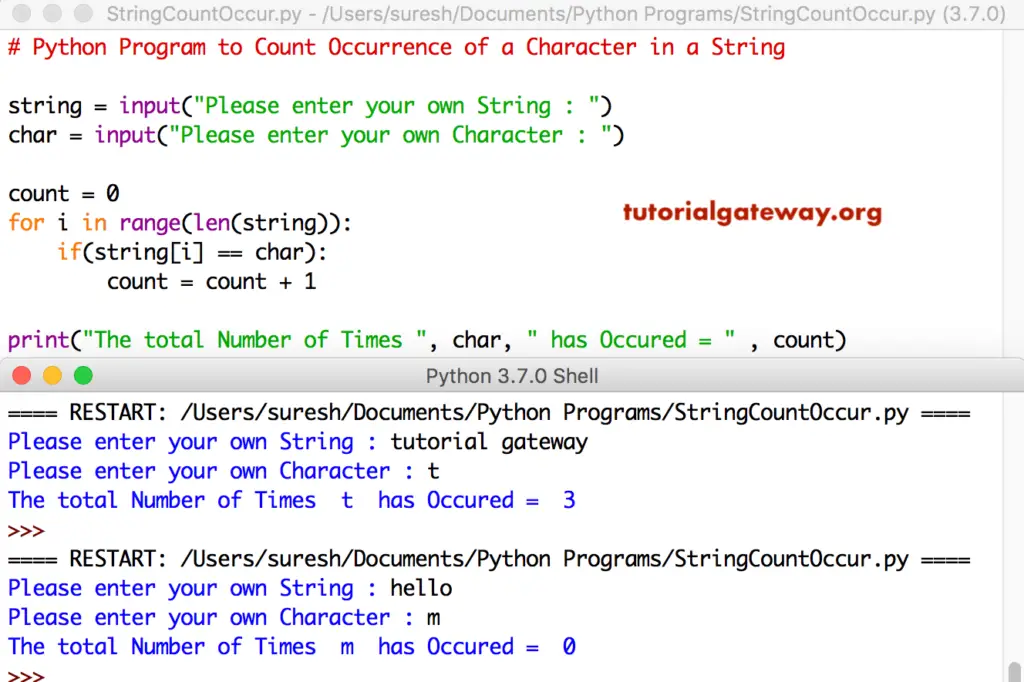
Here, we used For Loop to iterate each character in a String. Inside the Python For Loop, we used the If statement to check whether any character in a string is equal to the given character or not. If true, then count = count + 1.
string = tutorial gateway
ch = t
count = 0
For Loop First Iteration: for i in range(11)
if(string[i] == char)
if(t == t) – Condition is True.
count = 0 + 1 => 1
Second Iteration: for 1 in range(11)
if(u == l) – Condition is false.
Third Iteration: for 2 in range(11)
if(string[2] == char) => if(t == t) – Condition is True.
count = 1 + 1 => 2
Do the same for the remaining Program iterations
Python Program to Count Occurrence of a Character Example 2
This Python counting total occurrence of a character in a string program is the same as the above. However, we just replaced the For Loop with While Loop.
# Python Program to Count Occurrence of a Character in a String string = input("Please enter your own String : ") char = input("Please enter your own Character : ") i = 0 count = 0 while(i < len(string)): if(string[i] == char): count = count + 1 i = i + 1 print("The total Number of Times ", char, " has Occurred = " , count)
python character occurrence in a string output
Please enter your own String : python programs
Please enter your own Character : p
The total Number of Times p has Occurred = 2
>>>
Please enter your own String : hello
Please enter your own Character : l
The total Number of Times l has Occurred = 2
Python Program to Count Total Occurrence of a Character Example 3
This Python total occurrence of a given Character program is the same as the first example. But, this time, we used the Functions concept to separate the logic.
# Python Program to Count Occurrence of a Character in a String def count_Occurrence(ch, str1): count = 0 for i in range(len(string)): if(string[i] == char): count = count + 1 return count string = input("Please enter your own String : ") char = input("Please enter your own Character : ") cnt = count_Occurrence(char, string) print("The total Number of Times ", char, " has Occurred = " , cnt)
python character occurrence in a string output
Please enter your own String : Python tutorial
Please enter your own Character : t
The total Number of Times t has Occurred = 3
>>>
Please enter your own String : hi
Please enter your own Character : g
The total Number of Times g has Occurred = 0