Write a Python Program to Count Even and Odd Numbers in Tuple using the for loop range (for i in range(len(evodTuple))). The if condition (if(evodTuple[i] % 2 == 0)) checks whether the Tuple item is exactly divisible by two. If True, we add one to the even Tuple count; otherwise, add one to the odd Tuple count value.
# Count of Tuple Even and Odd Numbers evodTuple = (2, 33, 45, 88, 77, 98, 54, 0, 17) print("Even and Odd Tuple Items = ", evodTuple) tEvenCount = tOddCount = 0 for i in range(len(evodTuple)): if(evodTuple[i] % 2 == 0): tEvenCount = tEvenCount + 1 else: tOddCount = tOddCount + 1 print("The Count of Even Numbers in evodTuple = ", tEvenCount) print("The Count of Odd Numbers in evodTuple = ", tOddCount)
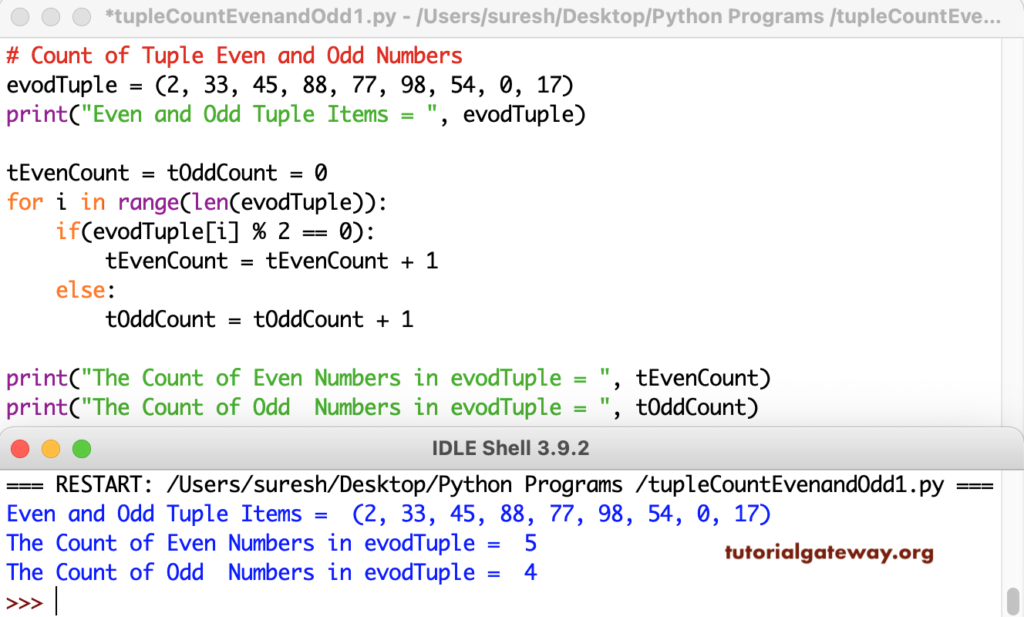
Python Program to Count Even and Odd Numbers in Tuple
In this Python example, we used the for loop (for oetup in evodTuple) to iterate the actual tuple values and inspect whether they divisible by two equal to zero.
# Count of Tuple Even and Odd Numbers evodTuple = (11, 34, 17, 44, 66, 19, 89, 64, 90) print("Even and Odd Tuple Items = ", evodTuple) tEvenCount = tOddCount = 0 for oetup in evodTuple: if(oetup % 2 == 0): tEvenCount = tEvenCount + 1 else: tOddCount = tOddCount + 1 print("The Count of Even Numbers in evodTuple = ", tEvenCount) print("The Count of Odd Numbers in evodTuple = ", tOddCount)
Even and Odd Tuple Items = (11, 34, 17, 44, 66, 19, 89, 64, 90)
The Count of Even Numbers in evodTuple = 5
The Count of Odd Numbers in evodTuple = 4
Python Program to Count Tuple Even and Odds using the While Loop.
# Count of Tuple Even and Odd Numbers evodTuple = (64, 45, 12, 15, 68, 55, 37, 25, 120, 205) print("Even and Odd Tuple Items = ", evodTuple) tEvenCount = tOddCount = 0 i = 0 while (i < len(evodTuple)): if(evodTuple[i] % 2 == 0): tEvenCount = tEvenCount + 1 else: tOddCount = tOddCount + 1 i = i + 1 print("The Count of Even Numbers in evodTuple = ", tEvenCount) print("The Count of Odd Numbers in evodTuple = ", tOddCount)
Even and Odd Tuple Items = (64, 45, 12, 15, 68, 55, 37, 25, 120, 205)
The Count of Even Numbers in evodTuple = 4
The Count of Odd Numbers in evodTuple = 6
In this Python Tuple example, we created a CountOfEvenOddOddNumbers function that returns the Even and Odd numbers count.
# Count of Tuple Even and Odd Numbers def CountOfEvenOddOddNumbers(evodTuple): tEvenCount = tOddCount = 0 for oetup in evodTuple: if(oetup % 2 == 0): tEvenCount = tEvenCount + 1 else: tOddCount = tOddCount + 1 return tEvenCount, tOddCount evodTuple = (12, 26, 77, 99, 66, 75, 14, 256, 19, 81, 11, 33) print("Even and Odd Tuple Items = ", evodTuple) evenCount, oddCount = CountOfEvenOddOddNumbers(evodTuple) print("The Count of Even Numbers in evodTuple = ", evenCount) print("The Count of Odd Numbers in evodTuple = ", oddCount)
Even and Odd Tuple Items = (12, 26, 77, 99, 66, 75, 14, 256, 19, 81, 11, 33)
The Count of Even Numbers in evodTuple = 5
The Count of Odd Numbers in evodTuple = 7