Write a Python program to check character is Vowel or Consonant with a practical example.
Python Program to check character is Vowel or Consonant
This python program allows a user to enter any character. Next, we are using the If Else Statement to check whether the user given character is Vowel or Consonant.
Here, the If statement checks whether the Python character is equal to a, e, i, o, u, A, E, I, O, U. If it is TRUE, it is a Vowel. Otherwise, it’s a Consonant.
ch = input("Please Enter Your Own Character : ") if(ch == 'a' or ch == 'e' or ch == 'i' or ch == 'o' or ch == 'u' or ch == 'A' or ch == 'E' or ch == 'I' or ch == 'O' or ch == 'U'): print("The Given Character ", ch, "is a Vowel") else: print("The Given Character ", ch, "is a Consonant")
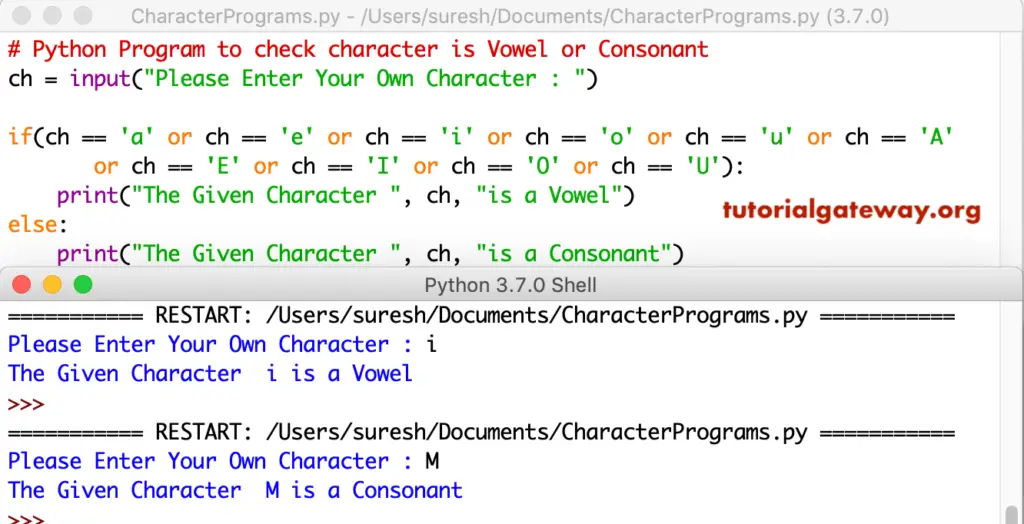
Python Program to check character is Vowel or Consonant using ASCII Values
In this example, we are using ASCII Values to check whether a given character is Vowel or Consonant.
ch = input("Please Enter Your Own Character : ") if(ord(ch) == 65 or ord(ch) == 69 or ord(ch) == 73 or ord(ch) == 79 or ord(ch) == 85 or ord(ch) == 97 or ord(ch) == 101 or ord(ch) == 105 or ord(ch) == 111 or ord(ch) == 117): print("The Given Character ", ch, "is a Vowel") elif((ord(ch) >= 97 and ord(ch) <= 122) or (ord(ch) >= 65 and ord(ch) <= 90)): print("The Given Character ", ch, "is a Consonant")
Please Enter Your Own Character : E
The Given Character E is a Vowel
>>>
Please Enter Your Own Character : l
The Given Character l is a Consonant