Write a Python program to check character is Digit or not with a practical example.
Python Program to check character is Digit or not
This python program allows a user to enter any character. Next, we use If Else Statement to check whether the user given character is a digit or not. Here, If statement checks the character is greater than or equal to 0 and, less than or equal to 9. If it is TRUE, it is a digit. Otherwise, it is not a digit.
# Python Program to check character is Digit or not ch = input("Please Enter Your Own Character : ") if(ch >= '0' and ch <= '9'): print("The Given Character ", ch, "is a Digit") else: print("The Given Character ", ch, "is Not a Digit")
Python character is digit or not output
Please Enter Your Own Character : 1
The Given Character 1 is a Digit
>>>
Please Enter Your Own Character : i
The Given Character i is Not a Digit
Python Program to find the character is Digit or not using ASCII Values
In this Python example, we use ASCII Values to check the character is a digit or not.
# Python Program to check character is Digit or not ch = input("Please Enter Your Own Character : ") if(ord(ch) >= 48 and ord(ch) <= 57): print("The Given Character ", ch, "is a Digit") else: print("The Given Character ", ch, "is Not a Digit")
Please Enter Your Own Character : 7
The Given Character 7 is a Digit
>>>
Please Enter Your Own Character : @
The Given Character @ is Not a Digit
Python Program to verify character is Digit using isdigit functions
In this example python code, we use the isdigit string function inside the If Else Statement to check whether a given character is a digit or not.
# Python Program to check character is Digit or not ch = input("Please Enter Your Own Character : ") if(ch.isdigit()): print("The Given Character ", ch, "is a Digit") else: print("The Given Character ", ch, "is Not a Digit")
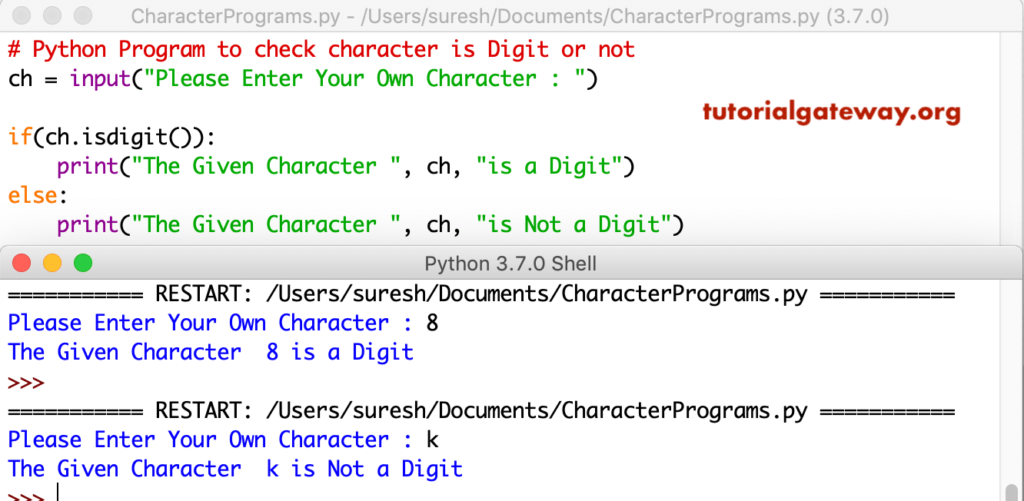