Write a Python program to check character is Alphabet or Digit with a practical example.
Python Program to check character is Alphabet or Digit
This python program allows a user to enter any character. Next, we are using Elif Statement to check whether the user given character is alphabet or digit.
- Here, If statement checks whether the character is between a and z or between A and Z, if it is TRUE, it is an alphabet. Otherwise, it enters into elif statement.
- Inside the Elif Statement, we are checking whether a given character is between 0 and 9. If it is true, it is a digit; otherwise, it is not a digit or alphabet.
# Python Program to check character is Alphabet or Digit ch = input("Please Enter Your Own Character : ") if((ch >= 'a' and ch <= 'z') or (ch >= 'A' and ch <= 'Z')): print("The Given Character ", ch, "is an Alphabet") elif(ch >= '0' and ch <= '9'): print("The Given Character ", ch, "is a Digit") else: print("The Given Character ", ch, "is Not an Alphabet or a Digit")
Python character is alphabet or digit output
Please Enter Your Own Character : j
The Given Character j is an Alphabet
>>>
Please Enter Your Own Character : 6
The Given Character 6 is a Digit
>>>
Please Enter Your Own Character : .
The Given Character . is Not an Alphabet or a Digit
Python Program to verify character is Alphabet or Digit using ASCII Values
In this Python code, we are using ASCII Values to check the character is an alphabet or digit.
# Python Program to check character is Alphabet or Digit ch = input("Please Enter Your Own Character : ") if(ord(ch) >= 48 and ord(ch) <= 57): print("The Given Character ", ch, "is a Digit") elif((ord(ch) >= 65 and ord(ch) <= 90) or (ord(ch) >= 97 and ord(ch) <= 122)): print("The Given Character ", ch, "is an Alphabet") else: print("The Given Character ", ch, "is Not an Alphabet or a Digit")
Please Enter Your Own Character : q
The Given Character q is an Alphabet
>>>
Please Enter Your Own Character : ?
The Given Character ? is Not an Alphabet or a Digit
>>>
Please Enter Your Own Character : 6
The Given Character 6 is a Digit
Python Program to find character is Alphabet or Digit using isalpha, isdigit functions
In this example python code, we use string functions called isdigit and isalpha to check whether a given character is an alphabet or digit.
# Python Program to check character is Alphabet or Digit ch = input("Please Enter Your Own Character : ") if(ch.isdigit()): print("The Given Character ", ch, "is a Digit") elif(ch.isalpha()): print("The Given Character ", ch, "is an Alphabet") else: print("The Given Character ", ch, "is Not an Alphabet or a Digit")
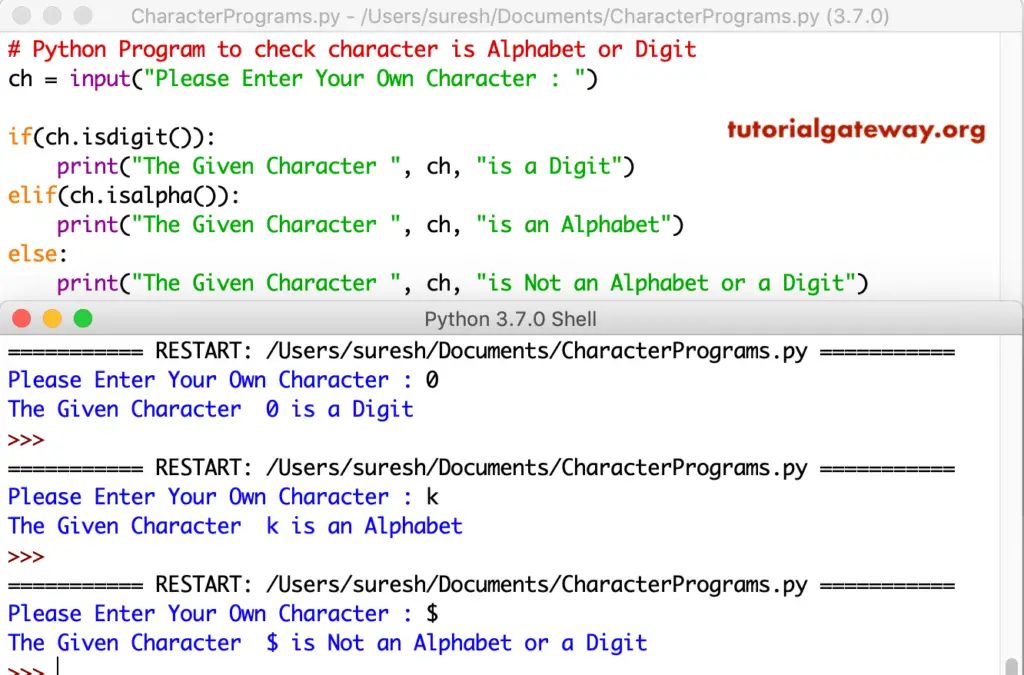