Write a Python program to check character is Alphabet digit or special character with a practical example.
Python Program to check character is Alphabet Digit or Special Character
This python program allows a user to enter any character. Next, we are using Elif Statement to check whether the user given character is alphabet or digit or special character.
- The first if statement checks whether the given character is between a and z or A and Z. If TRUE, it is an alphabet. Otherwise, it enters into elif statement.
- Inside the Elif Statement, we are checking whether a character is between 0 and 9. If True, it is a digit. Otherwise, it is a or special character.
# Python Program to check character is Alphabet Digit or Special Character ch = input("Please Enter Your Own Character : ") if((ch >= 'a' and ch <= 'z') or (ch >= 'A' and ch <= 'Z')): print("The Given Character ", ch, "is an Alphabet") elif(ch >= '0' and ch <= '9'): print("The Given Character ", ch, "is a Digit") else: print("The Given Character ", ch, "is a Special Character")
Python character is Alphabet, Digit or Special Character output
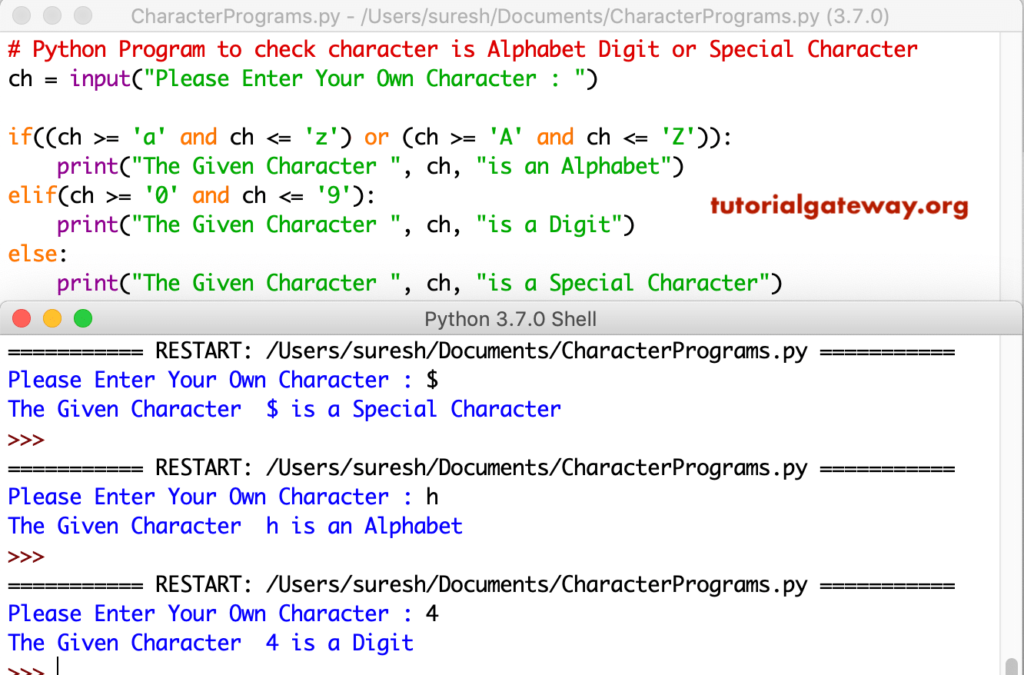
Python Program to verify character is Alphabet Digit or Special Character using ASCII Values
In this Python example, we are using ASCII Values to check the character is an alphabet or digit or special character.
# Python Program to check character is Alphabet Digit or Special Character ch = input("Please Enter Your Own Character : ") if(ord(ch) >= 48 and ord(ch) <= 57): print("The Given Character ", ch, "is a Digit") elif((ord(ch) >= 65 and ord(ch) <= 90) or (ord(ch) >= 97 and ord(ch) <= 122)): print("The Given Character ", ch, "is an Alphabet") else: print("The Given Character ", ch, "is a Special Character")
Python character is Alphabet, Digit or Special Character output
Please Enter Your Own Character : 0
The Given Character 0 is a Digit
>>>
Please Enter Your Own Character : r
The Given Character r is an Alphabet
>>>
Please Enter Your Own Character : @
The Given Character @ is a Special Character
Python Program to find character is Alphabet Digit or Special Character using isalpha, isdigit functions
In this python code, we use string functions called isdigit and isalpha to check whether a given character is an alphabet or digit or special character.
# Python Program to check character is Alphabet Digit or Special Character ch = input("Please Enter Your Own Character : ") if(ch.isdigit()): print("The Given Character ", ch, "is a Digit") elif(ch.isalpha()): print("The Given Character ", ch, "is an Alphabet") else: print("The Given Character ", ch, "is a Special Character")
Python character is Alphabet, Digit or Special Character output
Please Enter Your Own Character : 4
The Given Character 4 is a Digit
>>>
Please Enter Your Own Character : &
The Given Character & is a Special Character
>>>
Please Enter Your Own Character : b
The Given Character b is an Alphabet